Explain the purpose of Router services in Angular.
Last Updated :
19 Apr, 2024
The Router service in Angular is an important component that enables navigation within our single-page application (SPA). It involves mapping URLs to different components or views within the application and rendering the appropriate content based on the requested URL. When a user interacts with navigation links or changes the URL in the browser address bar, the Router intercepts these actions and dynamically updates the view displayed in the browser.
Prerequisites
What is Router Service?
The Router service is a powerful feature that enables navigation and routing between different components and views in a single-page-application (SPA). It allows us to handle and define navigation paths, and handle route parameters.
The Router service provides a comprehensive API for:
- Routes: Routes are mappings between URL paths and component classes. They define how the application’s UI should change in response to a URL change.
- Router Outlet: <router-outlet> is a directive used in Angular templates to render the component associated with the current route. It acts as a placeholder that Angular dynamically fills with the appropriate component.
- Route Parameters: Route parameters allow you to pass data to a route through the URL. For example, a route /users/:id can match /users/1, /users/2, etc., and the id parameter can be accessed in the component.
- Query Parameters: Query parameters are additional parameters added to a URL after a question mark (?). They are used to pass optional data to a route. For example, /products?category=1 might be used to filter products by category.
- Route Configuration: We can define routes using the RouterModule and Routes array in our app.module.ts file. Each route maps a URL path to a component or view function. Routes in an Angular application are configured using the RouterModule.forRoot() method in the root AppModule.
- Navigation: The Router service provides methods for navigating between routes programmatically. The navigate(), navigateByUrl(), and navigateByCommands() methods can be used to navigate to a specific route.
- URL Management: The Router interacts with the browser’s URL to reflect the current view. It also handles URL parsing, query parameters, and fragment identifiers.
- Route guards : These are used to protect routes and control access based on certain conditions. Angular provides several types of guards, including CanActivate, CanActivateChild, CanDeactivate, Resolve, and CanLoad.
- Router events : They provide information about the navigation lifecycle. Events like NavigationStart, NavigationEnd, NavigationCancel, and NavigationError can be subscribed to in order to perform actions based on the current navigation state.
Syntax:
Routes definition
import { Routes } from '@angular/router';
const routes: Routes = [
{ path: 'about', component: AboutComponent }, // Simple route
{ path: 'products/:id', component: ProductDetailComponent }, // Dynamic route with params
{ path: '**', component: NotFoundComponent } // Default route for unmatched paths
];
Router Configuration:
import { RouterModule } from '@angular/router';
@NgModule({
imports: [
RouterModule.forRoot(appRoutes) // Application-wide routing configuration
],
// ...
})
export class AppModule { }
In this way we can define and configure routes, and now we can directly use routerLink to navigate between components.
Features
Here are some key features of the Router service:
- Declarative Route Configuration: Define routes in a clear and concise manner using the RouterModule and Routes array.
- Dynamic Navigation: Navigate to different parts of your application using programmatic methods or user interactions with navigation components.
- URL Synchronization: The displayed view is always in sync with the URL in the browser address bar.
- Guards and Resolvers: Enhance security and improve performance by implementing route guards and resolvers.
- Nested Routes: Create hierarchical route structures for complex layouts.
- Event Handling: React to navigation events at different stages to perform actions like loading data or displaying loading indicators.
- Faster Page Transitions: With routing, only the necessary components and data for the requested route are loaded, resulting in faster page transitions.
- Improved User Experience: Routing enables a seamless and interactive user experience by allowing users to navigate between different views or pages within the application without a full page reload.
Purpose of Router services
The primary purpose of the routing service in Angular is to:
- URL-based navigation: The routing service enables navigation based on the URL. Each route is associated with a specific URL, and when a user navigates to a particular URL or clicks on a link, the routing service matches the URL with the defined routes and loads the associated component.
- Component rendering: The routing service is responsible for dynamically rendering the appropriate component based on the current route. When a route is activated, the routing service loads the corresponding component and inserts it into the designated area of the application’s layout or template.
- Handle Route Parameters: Angular routing also supports passing parameters through the URL. These parameters can be used to customise the behaviour or content of the displayed component. The routing service provides mechanisms to extract and utilise these parameters in your components.
- Maintain Application State: The routing service helps in maintaining the application state as users navigate between different routes. It allows us to preserve data and component states during navigation, ensuring a smooth user experience.
Steps to create Angular application
Step 1: Create the angular project using the following command.
ng new project
Folder Structure:
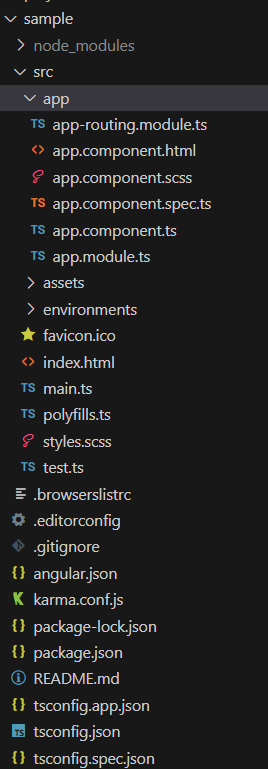
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/platform-server": "^17.3.0",
"@angular/router": "^17.3.0",
"@angular/ssr": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/express": "^4.17.17",
"@types/jasmine": "~5.1.0",
"@types/node": "^18.18.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Now let us learn how to create routes for our application
Step 2: Let us create home and about components
ng g ccomponent home
ng g component about
Step 3: Implement the Code
HTML
<!-- app.component.html -->
<h1>Angular Router Example</h1>
<nav>
<a routerLink="/home" routerLinkActive="active">Home</a>
<a routerLink="/about" routerLinkActive="active">About</a>
</nav>
<router-outlet></router-outlet>
HTML
<!-- home.component.html -->
<p>home works!</p>
HTML
<!-- about.component.html -->
<p>about works!</p>
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
constructor(private router: Router) { }
navigateToHome() {
this.router.navigate(['/home']);
}
navigateToAbout() {
this.router.navigate(['/about']);
}
}
JavaScript
//app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: '', redirectTo: '/home', pathMatch: 'full' },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule { }
To start the application run the following command.
ng serve
Output:
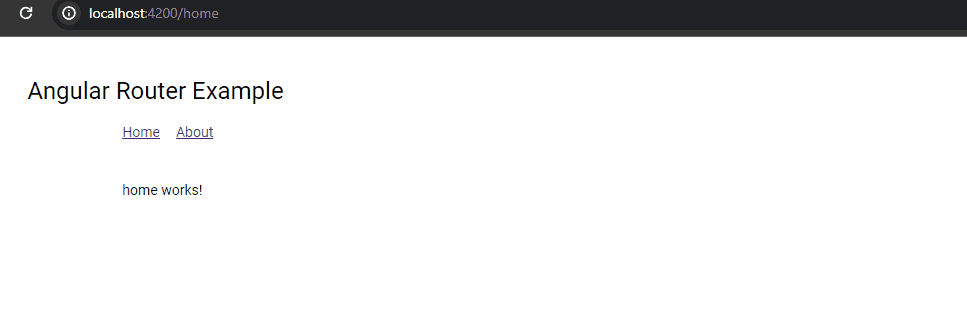
Share your thoughts in the comments
Please Login to comment...