Purpose of ProvidedIn in Angular
Last Updated :
02 Apr, 2024
Angular’s dependency injection system is a powerful mechanism that helps manage dependencies between components, services, and other parts of the application. One important aspect of this system is the providedIn property, which determines the scope and visibility of a service or module. In this article, we’ll explore the purpose of providedIn in Angular and how it relates to the overall dependency injection mechanism.
What is dependency injection?
Dependency Injection is a design pattern that helps in the creation and management of object dependencies. In Angular, components and services often have dependencies on other components or services, and dependency injection allows these dependencies to be provided to the dependent objects at runtime. This helps in modularity, reusability, and testability in Angular applications.
What is Providedln?
The providedIn property is used to specify the root injector or module where a service or module should be provided. It essentially tells Angular where the dependency should be available and how it should be instantiated. This property is typically used in the @Injectable() decorator for services or in the module metadata for modules.
When you create a service in Angular, you can specify its providedIn property to control its visibility and scope. There are three main options for the providedIn property:
- Root: If you set providedIn: ‘root’, the service will be available throughout the entire application, and only one instance of the service will be created and shared across all components and services that inject it.
- Module: If you set providedIn: SomeModule, the service will be available only within the specified module and its child modules. A new instance of the service will be created for each child module that injects it.
- Component: If you don’t specify the providedIn property or set it to null, the service will be available only within the component that creates it. A new instance of the service will be created for each component that injects it.
Features of Providedln
- Scoping and Visibility: By controlling the scope of services, you can ensure that they are only available where they are needed that helps in better code organization and maintainability.
- Singleton vs. Multiple Instances: Depending on your application’s requirements, you can choose to have a single instance of a service shared across the entire application or multiple instances for different modules or components.
- Lazy Loading: When using the Angular module system with lazy loading, specifying the providedIn property correctly can help optimize your application’s performance by ensuring that services are only loaded when needed.
Example of ProvidedIn in Angular
To illustrate the usage of providedIn, let’s create a simple Angular application with a service and two components:
Step 1: Generate a new Angular application using the Angular CLI and navigate to the project:
ng new provided-in-example
cd provided-in-example
Step 2: Generate a service named data using the following command.
ng generate service data
Step 3: Generate two components called ComponentA and ComponentB:
ng generate component component-a
ng generate component component-b
Folder Structure:
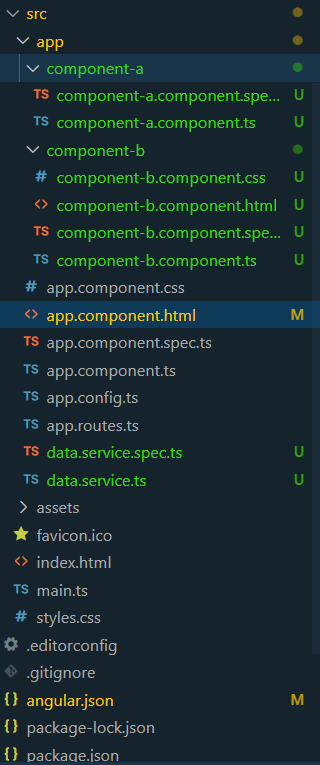
Folder Structure
The updated Dependencies in package.json file will look like:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Code Example: Follow all the steps and add the following codes in the respective files.
HTML
<!-- app.component.html -->
<app-component-a></app-component-a>
<app-component-b></app-component-b>
JavaScript
//data.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
data = 'This is data from the DataService';
getData() {
return this.data;
}
}
JavaScript
//component-a.component.ts
import { Component } from '@angular/core';
import { DataService } from '../data.service';
@Component({
selector: 'app-component-a',
template: `
<h2>Component A</h2>
<p>{{ data }}</p>
`,
standalone: true,
})
export class ComponentAComponent {
data: string;
constructor(private dataService: DataService) {
this.data = dataService.getData();
}
}
JavaScript
//component-b.component.ts
import { Component } from '@angular/core';
import { DataService } from '../data.service';
@Component({
selector: 'app-component-b',
template: `
<h2>Component B</h2>
<p>{{ data }}</p>
`,
standalone: true,
})
export class ComponentBComponent {
data: string;
constructor(private dataService: DataService) {
this.data = dataService.getData();
}
}
Step 4: Run the development server:
ng serve --open
Output: When you run the application with ng serve –open and navigate to http://localhost:4200/, you should see the following output:
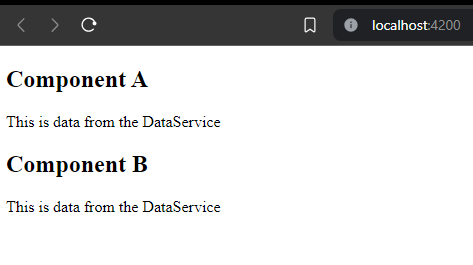
Output
Conclusion
Both components are able to access the same instance of the DataService class because it was provided at the root level.
Above example demonstrates how the providedIn property can be used to control the visibility and scope of services in an Angular application. By specifying the appropriate providedIn value, you can manage dependencies effectively and optimize your application’s performance and maintainability.
Share your thoughts in the comments
Please Login to comment...