Node First Application
Last Updated :
11 Mar, 2024
Node is an open-source, cross-platform server environment that executes JavaScript using the V8 JavaScript Engine. Node helps to write front-end and back-end code in the same language. It helps to write efficient code for real-time applications.
As a beginner in development, most developers find it difficult to create their first Node.js Application. In this article, we will help you in building Node applications.
We will discuss the following two approaches to create our first application in Node:
Creating Console-based Node Application
The Node console-based applications are run using the Node command prompt. The console module in Node.js provides a simple debugging console. Node is a global console that can be used for synchronous as well as asynchronous communication.Â
The console.log() function is used to display output on the console. This function prints output to stdout with newline.
Syntax
console.log([data][, ...]);
Here, data is the content to be displayed on the console.
Example 1: Hello World application using Node.js.Â
Create a geeks.js file containing the following code:
javascript
console.log( 'Hello World' );
|
Run the file on the Node.js command prompt using the command node geeks.js i.e. node <file_name>.
Output:
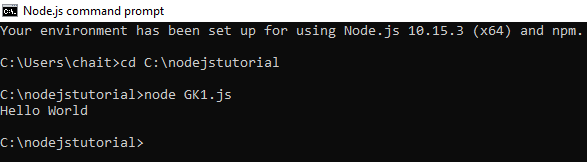
Example 2: Hello World application Receiving the User Input
Create a gfg.js file containing the following code.
javascript
console.log(process.argv.slice(2));
|
The process.argv is used to provide a command line argument to a program. Use the slice function with 2 as its argument to get all the elements of argv that come after its second element, i.e. the arguments the user entered.
The first argument is the location of the Node.js binary which runs the program and the second argument is the location of the file being run.
Output:
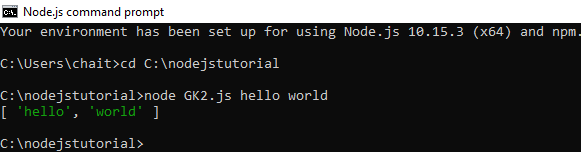
Creating Web-based Node Application
A web-based Node application consists of the following three important components:
- Import required module
- Create server
- Read Request and return response
Let us learn more about them in detail
Steps to Create Node Application (Web-Based)
Step 1: Import required modules
Load Node modules using the required directive. Load the http module and store the returned HTTP instance into a variable.
Syntax:
var http = require("http");
Step 2: Creating a server in Node
Create a server to listen to the client’s requests. Create a server instance using the createServer() method. Bind the server to port 8080 using the listen method associated with the server instance.
Syntax:
http.createServer().listen(8080);
Step 3: Read request and return response in Node:
Read the client request made using the browser or console and return the response. A function with request and response parameters is used to read client requests and return responses.
Syntax:
http.createServer(function (request, response) {...}).listen(8080);
First Node Application
After combining all these techniques, you can create a Node application. This code below creates a Hello World web-based application using Node.js.Â
javascript
var http = require( 'http' );
http.createServer( function (req, res) {
res.writeHead(200, { 'Content-Type' : 'text/html' });
res.end( 'Hello World!' );
}).listen(8080);
|
Step to Run Node Application:
Step 1: To run the program type the following command in terminal
node firstprogram.js
Step 2: Then type the following URL in the browser
http://127.0.0.1:8080/
Output:

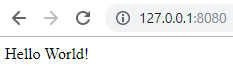
Share your thoughts in the comments
Please Login to comment...