Next.js Components : <Script>
Last Updated :
27 Mar, 2024
Next JS is a React-based web framework that enables developers to build modern, dynamic web applications easily. Vercel developed it, and NextJS introduces a set of conventions and tools that streamline the developer process making it faster and more efficient.
This post will teach us about this project’s <Script> component.
What are Script Components?
The ‘<Script>’ component is a way to include external scripts or libraries in your application. It is often used for loading third-party scripts or adding custom scripts to specific pages.
Syntax of Script Component
import Script from 'next/script'
export default function Dashboard() {
return (
<>
<Script src="https://example.com/script.js" />
</>
)
}
Props in <Script>:
There are the props available for the Script Component
- src: It is required prop in <Script> Component. This prop define the external javascript url.
- strategy: This prop allow you to control the behaviour of loading and executing the external script.
- onLoad: It is optional prop, It run when the external script finished the loading.
- onLoad: It is optional prop, This event code run after the script has finished loading and every time the when the component is mounted.
- onError: It is optional prop, This event occurs when your script fails to load.
Required Props:
There are the some required props in the <Script/> Component.
- src: A path string that specifying the URL of an external script. It can be absolute external URL or internal path. This prop is required otherwise inline script will be used.
Optional Props:
The <Script/> components also suppors the some additonal properties. It is not required properities.
Strategy
The ‘strategy’ property allows you to control how the script is loaded and executed. It accepts four values.
- beforeInteractive: When you use this value and It will be loaded and executed as soon as possible, before any other scripts on the page. This is useful for critical scripts that need to be executed early in the page lifecycle.
- afterInteractive: When you use this value in strategy property. It will be loaded and eecuted after the page’s content has been parsed and the initial HTML has been rendered. This is the default behavior.
- lazyOnload: This value load the script later during browser idle time.
- worker: It is experimental value. It will load the script in a web worker.
beforeInteractive:
This strategy is used for scripts that need to execute before the page’s content Interactive. This script is always downloaded prior to any NextJS module and then run in the sequence they appear.
JavaScript
import Script from 'next/script';
const MyPage = () => {
return (
<div>
<h1>My Page</h1>
{/* Script with hypothetical "beforeInteractive" behavior */}
<Script src="https://example.com/script.js" beforeInteractive />
</div>
);
};
export default MyPage;
afterInteractive:
This ‘afterInteractive‘ strategy in NextJS ‘<Script/>’ component allow you to specify that a script should be executed after that page’s content becomes interactive. This is the default strategy of the Script Component in NextJS afterInteractive scripts can be placed of any page or layout and will only load and execute when that page is opend in the browser.
JavaScript
import Script from 'next/script'
export default function Page() {
return (
<>
<Script src="https://example.com/script.js"
strategy="afterInteractive" />
</>
)
}
lazyOnload:
The ‘lazyOnload‘ strategy in NextJS ‘<Script/>’ component allows you to defer the loading and execution of a script until after all other resources (such as HTML , CSS, and other scripts) have finshed loading. It is benefical for improving page load perfomance.
JavaScript
import Script from 'next/script';
const MyPage = () => {
return (
<div>
<h1>My Page</h1>
{/* Script loaded with lazyOnload strategy */}
<Script src="https://example.com/lazy-load-script.js"
strategy="lazyOnload" />
</div>
);
};
export default MyPage;
worker:
The ‘worker‘ strategy in NextJS ‘<Script/>’ component allows freeing up the main thread to process only critcal first party resource first. It’s an advanced usage senario and may not support all third party scripts.
If you want to use worker as a strategy, is to enable the nextScriptWorker flag in the next.config.js
JavaScript
module.exports = {
experimental: {
nextScriptWorkers: true,
},
}
- After ,Then you can use worker as strategy as follows:
JavaScript
import Script from "next/script";
export default function Page() {
return (
<>
<Script
src='https://external-script-source.com/script.js'
strategy='worker'
/>
</>
);
}
onLoad:
It is work only the client Component not server component. Some third party scripts rquies users to run JavaScript code once after the script has finished loading in order to instantiate content or call a function.
JavaScript
"use client";
import Script from "next/script";
export default function HomePage() {
return (
<div>
<h2>Script tag will be used </h2>
<Script
src="https://connect.facebook.net/en_US/sdk.js"
strategy="lazyOnload"
onLoad={() =>
console.log(`script loaded correctly, window.FB has been populated`)
}
/>
</div>
);
}
onReady:
The onReady Property in the next/script component is a function that executes every time the script is loaded and the component is mounted. Developer can use a callback function to perform action after the script has finished loading. It only work on client side component.
JavaScript
import Script from "next/script";
export default function Page() {
return (
<>
<Script
src='https://external-script-source.com/script.js'
strategy='worker'
onReady={() => {
console.info("External Script is ready for use");
}}
/>
</>
);
}
onError:
The onError Property in the next/script component is a functio that is executed if the script fails to load. Developer can define a callback function to handle errors that occur during the loading of the script. This property cannot use in server side component. It only works on client side component.
JavaScript
"use client";
import Script from "next/script";
export default function Page() {
return (
<>
<Script
src='https://external-script-source.com/script.js'
strategy='worker'
onError={() => {
console.error("An error has occurred");
}}
/>
</>
);
}
Steps to Setup NextJS App:
Step 1: Install NodeJs. Follow one of the links to install according to your system, Windows and Linux.
npm -v
node -v
Step 2: You can create a next app using the following command. It is the easiest way to developed next app using the CLI(command line interpreter) tool you can quickly start building a new Next.js application.
npx create-next-app@latest
Step 3: Navigate to your app/project directory. After creating a Next Project we should change directory to the Next Project. By using this command:
cd project_directory_name
Project Structure:
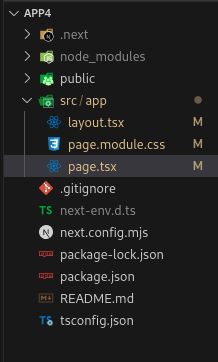
Example 1: In this example, we will use the Script tag in Next.js. This tag will include external JavaScript.
Javascript
"use client";
import Script from "next/script";
export default function HomePage() {
return (
<div>
<h2>Script tag will be used </h2>
<Script
src="https://connect.facebook.net/en_US/sdk.js"
strategy="lazyOnload"
onLoad={() =>
console.log(`script loaded correctly, window.FB has been populated`)
}
/>
</div>
);
}
Output:
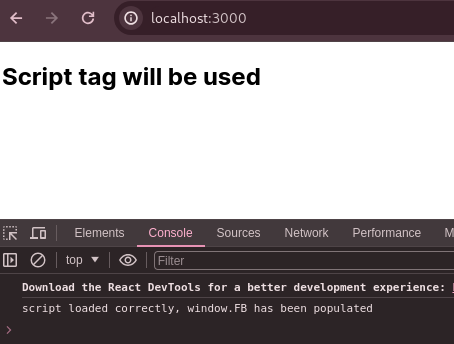
Example 2: In this example, we will use the “error” prop in Next.js
Javascript
"use client";
import Script from "next/script";
export default function HomePage() {
return (
<div>
<h2> Failed in including External Site</h2>
<Script
src="https://example.com/script.js"
onError={(e: Error) => {
console.log("Script failed to load", e);
}}
/>
</div>
);
}
Output:
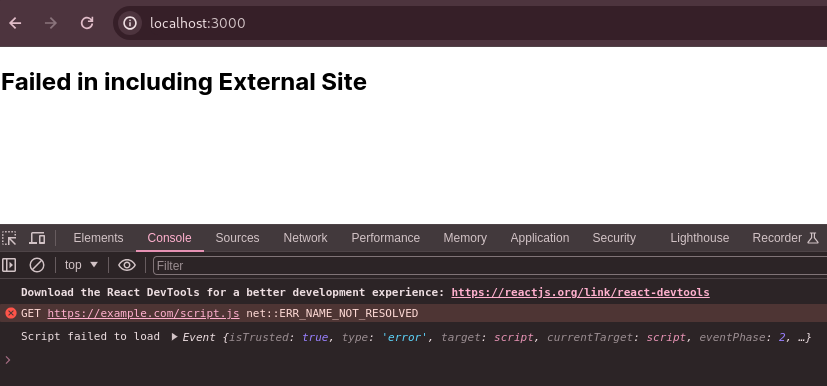
Share your thoughts in the comments
Please Login to comment...