Next.js Components
Last Updated :
19 Apr, 2024
NextJS is a popular and powerful React Framework that simplifies building modern web applications. It was created by Vercel (formerly Zeit), and NextJS simplifies the process of building modern web applications by providing a robust framework.
What is a Component?
Components are the building blocks in React-based applications, and Next.js provides a powerful way to work with them. It is reusable and it contains (HTML and JSX) and behavior (JavaScript logic) of a UI element, making it easier to manage and reusable code across different parts of an application.
Syntax:
<Component_Name />
Image Component
In Next.js, There is an Image component that is an evolved from of <img/> element in HTML. It use for performance optimization which helps in achieving the good core web vitals. It help to boost Google’ ranking algorithm, hense improving the ranking of our website.
Some Common features of Image Component.
- Page Loading Faster: It supports various configuration such as reszing the Image component via props.
- Improved Performance of Website: It serves different image sizes for each device, which reduce size for smaller devices and thus improvise performance.
Props of Image Component: There are the some required props
- src(required): This prop accept the path string, an URL of the image.
- width(required): This prop defines the width size of the image.
- height(required): This prop defines the height size of the image
Example: In this example, we will import the simple image in our page using the Image Component.
JavaScript
import Image from "next/image";
export default function ShowImage() {
return (
<div>
<Image
src="https://media.geeksforgeeks.org/wp-content/cdn-uploads/20210420155809/gfg-new-logo.png"
height="100"
width="400"
alt="GFG logo served from external URL"
/>
</div>
);
}
JavaScript
import ShowImage from "./components/ShowImage";
export default function Home() {
return (
<div>
<ShowImage />
</div>
);
}
JavaScript
import { hostname } from 'os';
/** @type {import('next').NextConfig} */
const nextConfig = {
images: {
remotePatterns: [
{
hostname: "media.geeksforgeeks.org",
},
],
},
};
export default nextConfig;
Output:
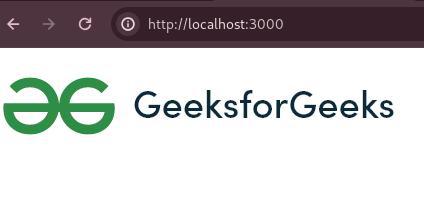
Link Component
Link is one of the prebult component in Next.js. It is used to create links between the pages in a Next.js app. It is very similar <a/> anchor HTML element. I have written.
There are some common props in Link Component
- href: The path to the page you want to link to.
- rel: The type of link. Possible value are “external”, “internal or “none”.
- title: The title of the link.
- active: Whether the link is active or not.
Example 2: In this example, we will create a two js file in page folder one is index.js other is about. In this example, we are using the Page Routing in Next.js
Project Structure:
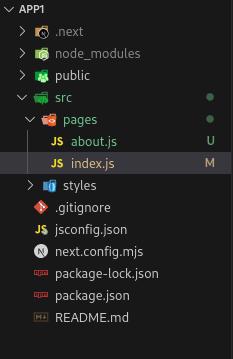
Example:
JavaScript
import Link from "next/link";
export default function Home() {
return (
<div>
<div>Home Page</div>
<Link href="/about">About Page</Link>
</div>
);
}
JavaScript
import Link from "next/link";
export default function About() {
return (
<div>
<div>About Page</div>
<Link href="/">Back To Home Page</Link>
</div>
);
}
Output:
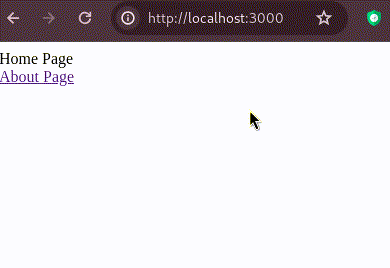
Script Component
The ‘<Script>’ component is a way to include external scripts or libraries in your application. It is often used for loading third-party scripts or adding custom scripts to specific pages. This is a prebuilt Component in Next.js
There are the some Commone Props in <Script/> Component
- src: It is required prop in <Script> Component. This prop define the external javascript url.
- strategy: This prop allow you to control the behaviour of loading and executing the external script.
Example:
JavaScript
import Script from 'next/script'
export default function Page() {
return (
<>
<Script src="https://example.com/script.js"
strategy="afterInteractive" />
</>
)
}
Share your thoughts in the comments
Please Login to comment...