Functions in Next JS
Last Updated :
12 Feb, 2024
Next JS is a React-based open-source web development framework created by Vercel. It is used to build production-ready web applications and comes equipped with features like hot code reloading, data fetching utilities, dynamic API routes, and optimized builds. The framework is built upon React, Webpack, and Babel. NextJS is commonly used for creating landing pages and marketing websites, and it also includes inbuilt features to improve webpage search ranking. One of the biggest advantages of using NextJS is that it solves the routing problem that we often encounter while using React.
Functions in Next JS:
- Functions are crucial in NextJS application development as they provide sets of instructions to execute actions and yield results.
- In NextJS, functions can be either functional components or arrow functions that return JSX.
- NextJS supports various types of functions such as Data Fetching functions, Cookies functions, Header functions, Routing functions, redirect functions, and revalidatePath functions.
- Function names in NextJS should start with a capital letter.
- Functions can be exported from one file to another for reuse, typically accomplished using the
export
statement.
- To use functions from other files, the
import
statement is employed to import them.
Syntax:
export default function function_name() {
return {
// function body with JSX
}
}
Steps to Create the NextJS Applcation:
- To create a NextJS project we require NodeJS installed on our system. You can install it from the official website of NodeJS
- To create a project run the following script in the terminal.
npx create-next-app app_name
- Navigate to the root directory of your project.
cd app_name
Project Structure:
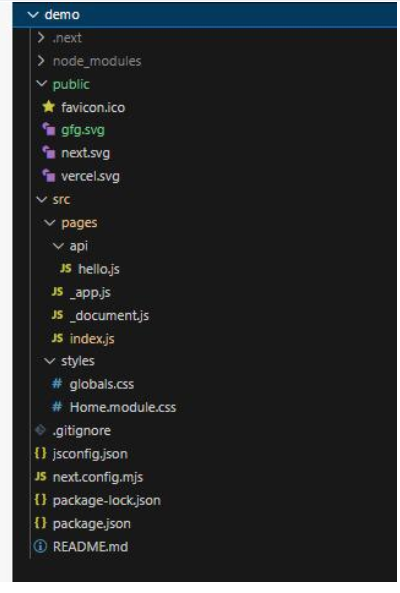
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.1.0"
}
Example: Below is the example of NextJS function.
- List function is declared to return the list items provided through the props.
- props.items.map() iterates over the item which are provided through the props.
- Home function is the main component ,It renders the list items.
Javascript
import Head from "next/head" ;
import { Inter } from "next/font/google" ;
import styles from "../app/globals.css" ;
const inter = Inter({ subsets: [ "latin" ] });
const List = (props) => (
<ul>
{
props.items.map((item) => (
<li key={item}>{item}</li>
))
}
</ul>
);
export default function Home() {
return (
<>
<div>
<h1>Welcome To Nextjs Functions</h1>
</div>
<main className={`${styles.main} ${inter.className}`}>
<div className= "container" >
<p>
List of Functions in NextJS
</p>
<List items={[ "Data Fetching function" ,
"Cookies function" ,
"Header function" ,
"Routing Function" ,
"redirect function" ,
"revalidatePath function" ]} >
</List>
</div>
</main>
</>
);
}
|
CSS
h 1 {
text-align : center ;
}
.container {
border : 4px dashed green ;
padding : 1.5 rem;
}
ul {
margin : 1 rem;
padding : 1 rem;
}
li{
padding-top : 0.5 rem;
}
@media (prefers-color-scheme: dark) {
html {
color-scheme: dark;
}
}
|
Start your application using the following command.
npm run dev
Output:
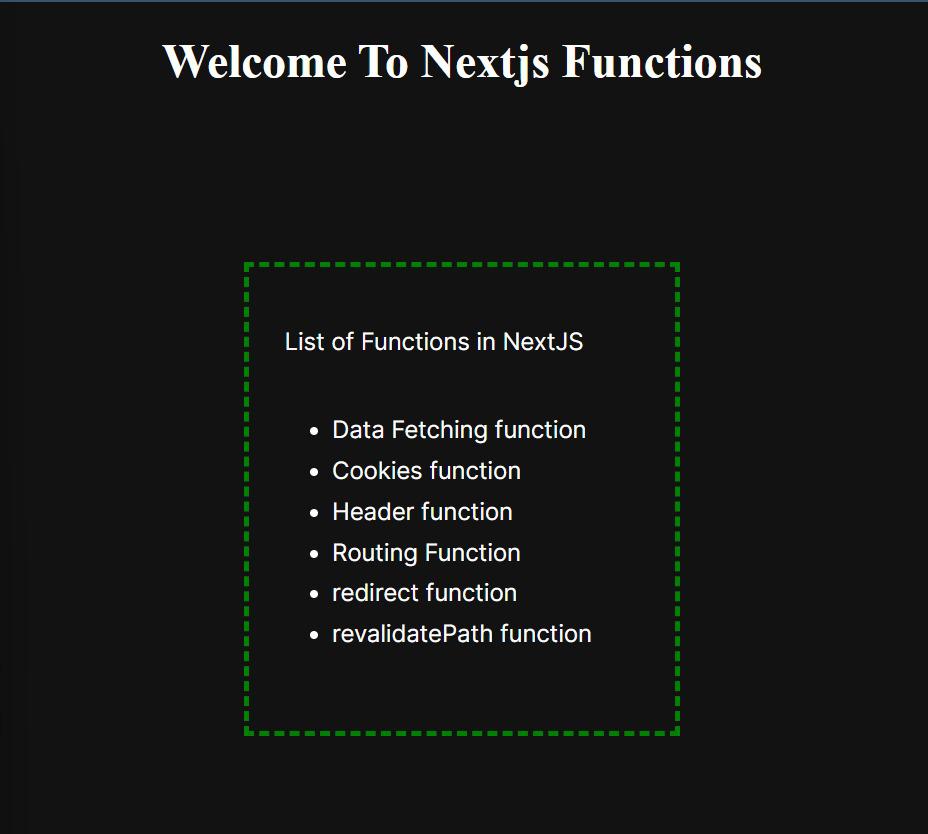
Output
Share your thoughts in the comments
Please Login to comment...