Next Greater Element (NGE) for every element in given Array
Last Updated :
29 Sep, 2023
Given an array, print the Next Greater Element (NGE) for every element.
The Next greater Element for an element x is the first greater element on the right side of x in the array. Elements for which no greater element exist, consider the next greater element as -1.
Example:
Input: arr[] = [ 4 , 5 , 2 , 25 ]
Output: 4 –> 5
5 –> 25
2 –> 25
25 –> -1
Explanation: except 25 every element has an element greater than them present on the right side
Input: arr[] = [ 13 , 7, 6 , 12 ]
Output: 13 –> -1
7 –> 12
6 –> 12
12 –> -1
Explanation: 13 and 12 don’t have any element greater than them present on the right side
The idea is to use two loops , The outer loop picks all the elements one by one. The inner loop looks for the first greater element for the element picked by the outer loop. If a greater element is found then that element is printed as next, otherwise, -1 is printed.
Follow the steps mentioned below to implement the idea:
- Traverse the array from index 0 to end.
- For each element start another loop from index i+1 to end.
- If a greater element is found in the second loop then print it and break the loop, else print -1.
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
void printNGE( int arr[], int n)
{
int next, i, j;
for (i = 0; i < n; i++) {
next = -1;
for (j = i + 1; j < n; j++) {
if (arr[i] < arr[j]) {
next = arr[j];
break ;
}
}
cout << arr[i] << " --> " << next << endl;
}
}
int main()
{
int arr[] = { 11, 13, 21, 3 };
int n = sizeof (arr) / sizeof (arr[0]);
printNGE(arr, n);
return 0;
}
|
C
#include <stdio.h>
void printNGE( int arr[], int n)
{
int next, i, j;
for (i = 0; i < n; i++) {
next = -1;
for (j = i + 1; j < n; j++) {
if (arr[i] < arr[j]) {
next = arr[j];
break ;
}
}
printf ( "%d -- %dn" , arr[i], next);
}
}
int main()
{
int arr[] = { 11, 13, 21, 3 };
int n = sizeof (arr) / sizeof (arr[0]);
printNGE(arr, n);
return 0;
}
|
Java
class Main {
static void printNGE( int arr[], int n)
{
int next, i, j;
for (i = 0 ; i < n; i++) {
next = - 1 ;
for (j = i + 1 ; j < n; j++) {
if (arr[i] < arr[j]) {
next = arr[j];
break ;
}
}
System.out.println(arr[i] + " -- " + next);
}
}
public static void main(String args[])
{
int arr[] = { 11 , 13 , 21 , 3 };
int n = arr.length;
printNGE(arr, n);
}
}
|
Python
def printNGE(arr):
for i in range ( 0 , len (arr), 1 ):
next = - 1
for j in range (i + 1 , len (arr), 1 ):
if arr[i] < arr[j]:
next = arr[j]
break
print ( str (arr[i]) + " -- " + str ( next ))
arr = [ 11 , 13 , 21 , 3 ]
printNGE(arr)
|
C#
using System;
class GFG {
static void printNGE( int [] arr, int n)
{
int next, i, j;
for (i = 0; i < n; i++) {
next = -1;
for (j = i + 1; j < n; j++) {
if (arr[i] < arr[j]) {
next = arr[j];
break ;
}
}
Console.WriteLine(arr[i] + " -- " + next);
}
}
public static void Main()
{
int [] arr = { 11, 13, 21, 3 };
int n = arr.Length;
printNGE(arr, n);
}
}
|
Javascript
<script>
function printNGE(arr, n)
{
var next, i, j;
for (i = 0; i < n; i++)
{
next = -1;
for (j = i + 1; j < n; j++)
{
if (arr[i] < arr[j])
{
next = arr[j];
break ;
}
}
document.write(arr[i] + " -- " + next);
document.write( "<br>" );
}
}
var arr = [11, 13, 21, 3];
var n = arr.length;
printNGE(arr, n);
</script>
|
PHP
<?php
function printNGE( $arr , $n )
{
for ( $i = 0; $i < $n ; $i ++)
{
$next = -1;
for ( $j = $i + 1; $j < $n ; $j ++)
{
if ( $arr [ $i ] < $arr [ $j ])
{
$next = $arr [ $j ];
break ;
}
}
echo $arr [ $i ]. " -- " . $next . "\n" ;
}
}
$arr = array (11, 13, 21, 3);
$n = count ( $arr );
printNGE( $arr , $n );
?>
|
Output
11 --> 13
13 --> 21
21 --> -1
3 --> -1
Time Complexity: O(N2)
Auxiliary Space: O(1)
Find Next Greater Element using Stack:
The idea is to store the elements for which we have to find the next greater element in a stack and while traversing the array, if we find a greater element, we will pair it with the elements from the stack till the top element of the stack is less than the current element.
illustration:
Below is the illustration of the above approach:
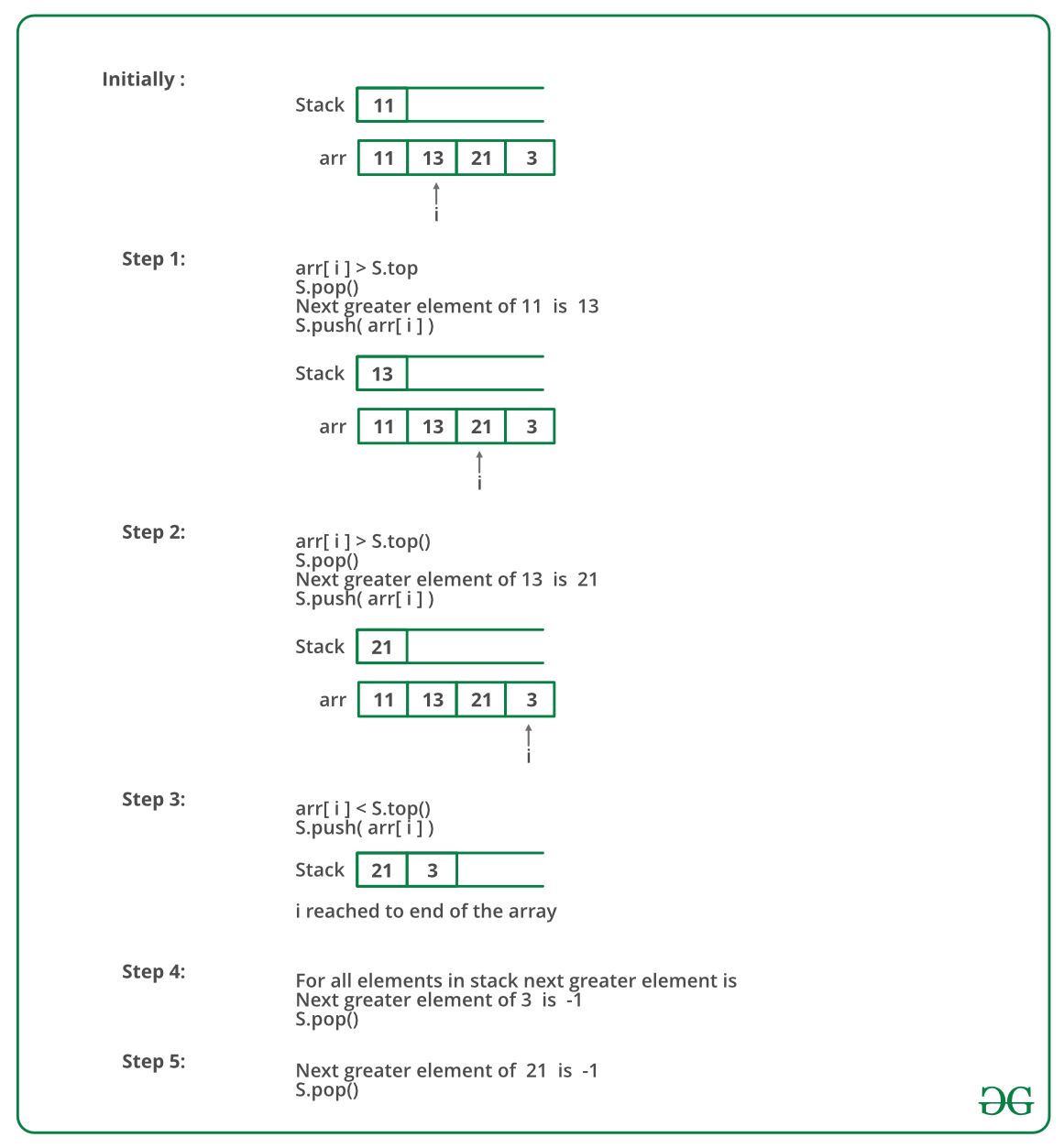
Follow the steps mentioned below to implement the idea:
- Push the first element to stack.
- Pick the rest of the elements one by one and follow the following steps in the loop.
- Mark the current element as next.
- If the stack is not empty, compare top most element of stack with next.
- If next is greater than the top element, Pop element from the stack. next is the next greater element for the popped element.
- Keep popping from the stack while the popped element is smaller than next. next becomes the next greater element for all such popped elements.
- Finally, push the next in the stack.
- After the loop in step 2 is over, pop all the elements from the stack and print -1 as the next element for them.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void printNGE( int arr[], int n)
{
stack< int > s;
s.push(arr[0]);
for ( int i = 1; i < n; i++) {
if (s.empty()) {
s.push(arr[i]);
continue ;
}
while (s.empty() == false && s.top() < arr[i]) {
cout << s.top() << " --> " << arr[i] << endl;
s.pop();
}
s.push(arr[i]);
}
while (s.empty() == false ) {
cout << s.top() << " --> " << -1 << endl;
s.pop();
}
}
int main()
{
int arr[] = { 11, 13, 21, 3 };
int n = sizeof (arr) / sizeof (arr[0]);
printNGE(arr, n);
return 0;
}
|
C
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#define STACKSIZE 100
struct stack {
int top;
int items[STACKSIZE];
};
void push( struct stack* ps, int x)
{
if (ps->top == STACKSIZE - 1) {
printf ( "Error: stack overflown" );
getchar ();
exit (0);
}
else {
ps->top += 1;
int top = ps->top;
ps->items[top] = x;
}
}
bool isEmpty( struct stack* ps)
{
return (ps->top == -1) ? true : false ;
}
int pop( struct stack* ps)
{
int temp;
if (ps->top == -1) {
printf ( "Error: stack underflow n" );
getchar ();
exit (0);
}
else {
int top = ps->top;
temp = ps->items[top];
ps->top -= 1;
return temp;
}
}
void printNGE( int arr[], int n)
{
int i = 0;
struct stack s;
s.top = -1;
int element, next;
push(&s, arr[0]);
for (i = 1; i < n; i++) {
next = arr[i];
if (isEmpty(&s) == false ) {
element = pop(&s);
while (element < next) {
printf ( "n %d --> %d" , element, next);
if (isEmpty(&s) == true )
break ;
element = pop(&s);
}
if (element > next)
push(&s, element);
}
push(&s, next);
}
while (isEmpty(&s) == false ) {
element = pop(&s);
next = -1;
printf ( "n %d --> %d" , element, next);
}
}
int main()
{
int arr[] = { 11, 13, 21, 3 };
int n = sizeof (arr) / sizeof (arr[0]);
printNGE(arr, n);
getchar ();
return 0;
}
|
Java
public class NGE {
static class stack {
int top;
int items[] = new int [ 100 ];
void push( int x)
{
if (top == 99 ) {
System.out.println( "Stack full" );
}
else {
items[++top] = x;
}
}
int pop()
{
if (top == - 1 ) {
System.out.println( "Underflow error" );
return - 1 ;
}
else {
int element = items[top];
top--;
return element;
}
}
boolean isEmpty()
{
return (top == - 1 ) ? true : false ;
}
}
static void printNGE( int arr[], int n)
{
int i = 0 ;
stack s = new stack();
s.top = - 1 ;
int element, next;
s.push(arr[ 0 ]);
for (i = 1 ; i < n; i++) {
next = arr[i];
if (s.isEmpty() == false ) {
element = s.pop();
while (element < next) {
System.out.println(element + " --> "
+ next);
if (s.isEmpty() == true )
break ;
element = s.pop();
}
if (element > next)
s.push(element);
}
s.push(next);
}
while (s.isEmpty() == false ) {
element = s.pop();
next = - 1 ;
System.out.println(element + " -- " + next);
}
}
public static void main(String[] args)
{
int arr[] = { 11 , 13 , 21 , 3 };
int n = arr.length;
printNGE(arr, n);
}
}
|
Python
def createStack():
stack = []
return stack
def isEmpty(stack):
return len (stack) = = 0
def push(stack, x):
stack.append(x)
def pop(stack):
if isEmpty(stack):
print ( "Error : stack underflow" )
else :
return stack.pop()
def printNGE(arr):
s = createStack()
element = 0
next = 0
push(s, arr[ 0 ])
for i in range ( 1 , len (arr), 1 ):
next = arr[i]
if isEmpty(s) = = False :
element = pop(s)
while element < next :
print ( str (element) + " -- " + str ( next ))
if isEmpty(s) = = True :
break
element = pop(s)
if element > next :
push(s, element)
push(s, next )
while isEmpty(s) = = False :
element = pop(s)
next = - 1
print ( str (element) + " -- " + str ( next ))
arr = [ 11 , 13 , 21 , 3 ]
printNGE(arr)
|
C#
using System;
public class NGE {
public class stack {
public int top;
public int [] items = new int [100];
public virtual void push( int x)
{
if (top == 99) {
Console.WriteLine( "Stack full" );
}
else {
items[++top] = x;
}
}
public virtual int pop()
{
if (top == -1) {
Console.WriteLine( "Underflow error" );
return -1;
}
else {
int element = items[top];
top--;
return element;
}
}
public virtual bool Empty
{
get { return (top == -1) ? true : false ; }
}
}
public static void printNGE( int [] arr, int n)
{
int i = 0;
stack s = new stack();
s.top = -1;
int element, next;
s.push(arr[0]);
for (i = 1; i < n; i++) {
next = arr[i];
if (s.Empty == false ) {
element = s.pop();
while (element < next) {
Console.WriteLine(element + " --> "
+ next);
if (s.Empty == true ) {
break ;
}
element = s.pop();
}
if (element > next) {
s.push(element);
}
}
s.push(next);
}
while (s.Empty == false ) {
element = s.pop();
next = -1;
Console.WriteLine(element + " -- " + next);
}
}
public static void Main( string [] args)
{
int [] arr = new int [] { 11, 13, 21, 3 };
int n = arr.Length;
printNGE(arr, n);
}
}
|
Javascript
<script>
function printNGE(arr, n)
{
var s = [];
s.push(arr[0]);
for ( var i = 1; i < n; i++)
{
if (s.length == 0) {
s.push(arr[i]);
continue ;
}
while (s.length ==0 == false
&& s[s.length-1] < arr[i])
{
document.write( s[s.length-1]
+ " --> " + arr[i]+ "<br>" );
s.pop();
}
s.push(arr[i]);
}
while (s.length !=0) {
document.write( s[s.length-1] + " --> " + -1+ "<br>" );
s.pop();
}
}
var arr = [11, 13, 21, 3];
var n = arr.length;
printNGE(arr, n);
</script>
|
Output
11 --> 13
13 --> 21
3 --> -1
21 --> -1
Time Complexity: O(N)
Auxiliary Space: O(N)
Find Next Greater Element using Map:
In this particular approach we are using the map as our main stack
- This is same as above method but the elements are pushed and popped only once into the stack. The array is changed in place. The array elements are pushed into the stack until it finds a greatest element in the right of array. In other words the elements are popped from stack when top of the stack value is smaller in the current array element.
- Once all the elements are processed in the array but stack is not empty. The left out elements in the stack doesn’t encounter any greatest element . So pop the element from stack and change it’s index value as -1 in the array.
C++
#include <bits/stdc++.h>
using namespace std;
void nextLargerElement( int arr[], int n)
{
vector<unordered_map<string, int > > s;
for ( int i = 0; i < n; i++) {
while (s.size() > 0
&& s[s.size() - 1][ "value" ] < arr[i]) {
unordered_map<string, int > d = s[s.size() - 1];
s.pop_back();
arr[d[ "ind" ]] = arr[i];
}
unordered_map<string, int > e;
e[ "value" ] = arr[i];
e[ "ind" ] = i;
s.push_back(e);
}
while (s.size() > 0) {
unordered_map<string, int > d = s[s.size() - 1];
s.pop_back();
arr[d[ "ind" ]] = -1;
}
}
int main()
{
int arr[] = { 6, 8, 0, 1, 3 };
int n = 5;
nextLargerElement(arr, n);
for ( int i = 0; i < n; i++)
cout << arr[i] << " " ;
}
|
Java
import java.util.*;
class GFG
{
static void nextLargerElement( int [] arr, int n)
{
ArrayList<HashMap<String, Integer> > s = new ArrayList<HashMap<String, Integer> >();
for ( int i = 0 ; i < n; i++) {
while (s.size() > 0
&& s.get(s.size() - 1 ).get( "value" ) < arr[i]) {
HashMap<String, Integer> d = s.get(s.size() - 1 );
s.remove(s.size() - 1 );
arr[d.get( "ind" )] = arr[i];
}
HashMap<String, Integer> e = new HashMap<String, Integer>();
e.put( "value" , arr[i]);
e.put( "ind" , i);
s.add(e);
}
while (s.size() > 0 ) {
HashMap<String, Integer> d = s.get(s.size() - 1 );
s.remove(s.size() - 1 );
arr[d.get( "ind" )] = - 1 ;
}
}
public static void main(String[] args)
{
int [] arr = { 6 , 8 , 0 , 1 , 3 };
int n = 5 ;
nextLargerElement(arr, n);
for ( int i = 0 ; i < n; i++)
System.out.print(arr[i] + " " );
}
}
|
Python3
class Solution:
def nextLargerElement( self , arr, n):
s = []
for i in range ( len (arr)):
while s and s[ - 1 ].get( "value" ) < arr[i]:
d = s.pop()
arr[d.get( "ind" )] = arr[i]
s.append({ "value" : arr[i], "ind" : i})
while s:
d = s.pop()
arr[d.get( "ind" )] = - 1
return arr
if __name__ = = "__main__" :
print (Solution().nextLargerElement([ 6 , 8 , 0 , 1 , 3 ], 5 ))
|
C#
using System;
using System.Collections.Generic;
class GFG
{
static void nextLargerElement( int [] arr, int n)
{
List<Dictionary< string , int > > s = new List<Dictionary< string , int > >();
for ( int i = 0; i < n; i++) {
while (s.Count > 0
&& s[s.Count - 1][ "value" ] < arr[i]) {
Dictionary< string , int > d = s[s.Count - 1];
s.RemoveAt(s.Count - 1);
arr[d[ "ind" ]] = arr[i];
}
Dictionary< string , int > e = new Dictionary< string , int >();
e[ "value" ] = arr[i];
e[ "ind" ] = i;
s.Add(e);
}
while (s.Count > 0) {
Dictionary< string , int > d = s[s.Count - 1];
s.RemoveAt(s.Count - 1);
arr[d[ "ind" ]] = -1;
}
}
public static void Main( string [] args)
{
int [] arr = { 6, 8, 0, 1, 3 };
int n = 5;
nextLargerElement(arr, n);
for ( int i = 0; i < n; i++)
Console.Write(arr[i] + " " );
}
}
|
Javascript
function nextLargerElement(arr, n)
{
var s = [];
for ( var i = 0; i < arr.length; i++)
{
while (s.length > 0 && s[s.length - 1][ "value" ] < arr[i])
{
var d = s.pop();
arr[d[ "ind" ]] = arr[i];
}
s.push({ "value" : arr[i], "ind" : i});
}
while (s.length > 0)
{
d = s.pop();
arr[d[ "ind" ]] = -1;
}
return arr;
}
var arr = [6, 8, 0, 1, 3];
var n = 5;
console.log(nextLargerElement(arr, n));
|
Time Complexity: O(N)
Auxiliary Space: O(N)
Share your thoughts in the comments
Please Login to comment...