Introduction to Chinese Remainder Theorem
Last Updated :
28 Nov, 2022
We are given two arrays num[0..k-1] and rem[0..k-1]. In num[0..k-1], every pair is coprime (gcd for every pair is 1). We need to find minimum positive number x such that:
x % num[0] = rem[0],
x % num[1] = rem[1],
.......................
x % num[k-1] = rem[k-1]
Basically, we are given k numbers which are pairwise coprime, and given remainders of these numbers when an unknown number x is divided by them. We need to find the minimum possible value of x that produces given remainders.
Examples :
Input: num[] = {5, 7}, rem[] = {1, 3}
Output: 31
Explanation:
31 is the smallest number such that:
(1) When we divide it by 5, we get remainder 1.
(2) When we divide it by 7, we get remainder 3.
Input: num[] = {3, 4, 5}, rem[] = {2, 3, 1}
Output: 11
Explanation:
11 is the smallest number such that:
(1) When we divide it by 3, we get remainder 2.
(2) When we divide it by 4, we get remainder 3.
(3) When we divide it by 5, we get remainder 1.
Chinese Remainder Theorem states that there always exists an x that satisfies given congruences. Below is theorem statement adapted from wikipedia.
Let num[0], num[1], …num[k-1] be positive integers that are pairwise coprime. Then, for any given sequence of integers rem[0], rem[1], … rem[k-1], there exists an integer x solving the following system of simultaneous congruences.
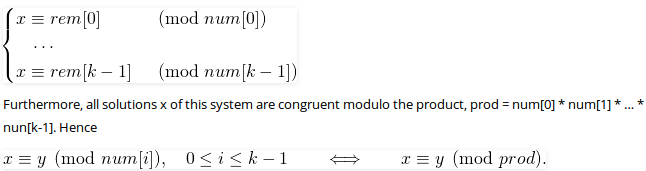
The first part is clear that there exists an x. The second part basically states that all solutions (including the minimum one) produce the same remainder when divided by-product of num[0], num[1], .. num[k-1]. In the above example, the product is 3*4*5 = 60. And 11 is one solution, other solutions are 71, 131, .. etc. All these solutions produce the same remainder when divided by 60, i.e., they are of form 11 + m*60 where m >= 0.
A Naive Approach to find x is to start with 1 and one by one increment it and check if dividing it with given elements in num[] produces corresponding remainders in rem[]. Once we find such an x, we return it.
Below is the implementation of Naive Approach.
C++
#include<bits/stdc++.h>
using namespace std;
int findMinX( int num[], int rem[], int k)
{
int x = 1;
while ( true )
{
int j;
for (j=0; j<k; j++ )
if (x%num[j] != rem[j])
break ;
if (j == k)
return x;
x++;
}
return x;
}
int main( void )
{
int num[] = {3, 4, 5};
int rem[] = {2, 3, 1};
int k = sizeof (num)/ sizeof (num[0]);
cout << "x is " << findMinX(num, rem, k);
return 0;
}
|
Java
import java.io.*;
class GFG {
static int findMinX( int num[], int rem[], int k)
{
int x = 1 ;
while ( true )
{
int j;
for (j= 0 ; j<k; j++ )
if (x%num[j] != rem[j])
break ;
if (j == k)
return x;
x++;
}
}
public static void main(String args[])
{
int num[] = { 3 , 4 , 5 };
int rem[] = { 2 , 3 , 1 };
int k = num.length;
System.out.println( "x is " + findMinX(num, rem, k));
}
}
|
Python3
def findMinX(num, rem, k):
x = 1 ;
while ( True ):
j = 0 ;
while (j < k):
if (x % num[j] ! = rem[j]):
break ;
j + = 1 ;
if (j = = k):
return x;
x + = 1 ;
num = [ 3 , 4 , 5 ];
rem = [ 2 , 3 , 1 ];
k = len (num);
print ( "x is" , findMinX(num, rem, k));
|
C#
using System;
class GFG
{
static int findMinX( int []num, int []rem,
int k)
{
int x = 1;
while ( true )
{
int j;
for (j = 0; j < k; j++ )
if (x % num[j] != rem[j])
break ;
if (j == k)
return x;
x++;
}
}
public static void Main()
{
int []num = {3, 4, 5};
int []rem = {2, 3, 1};
int k = num.Length;
Console.WriteLine( "x is " + findMinX(num,
rem, k));
}
}
|
PHP
<?php
function findMinX( $num , $rem , $k )
{
$x = 1;
while (true)
{
$j ;
for ( $j = 0; $j < $k ; $j ++ )
if ( $x % $num [ $j ] != $rem [ $j ])
break ;
if ( $j == $k )
return $x ;
$x ++;
}
return $x ;
}
$num = array (3, 4, 5);
$rem = array (2, 3, 1);
$k = sizeof( $num );
echo "x is " ,
findMinX( $num , $rem , $k );
?>
|
Javascript
<script>
function findMinX(num , rem , k)
{
var x = 1;
while ( true )
{
var j;
for (j=0; j<k; j++ )
if (x%num[j] != rem[j])
break ;
if (j == k)
return x;
x++;
}
}
var num = [3, 4, 5];
var rem = [2, 3, 1];
var k = num.length;
document.write( "x is " + findMinX(num, rem, k));
</script>
|
Output :
x is 11
Time Complexity : O(M), M is the product of all elements of num[] array.
Auxiliary Space : O(1)
See below link for an efficient method to find x.
Chinese Remainder Theorem | Set 2 (Inverse Modulo based Implementation)
Share your thoughts in the comments
Please Login to comment...