Program for factorial of a number
Last Updated :
12 Oct, 2023
What is the factorial of a number?
- Factorial of a non-negative integer is the multiplication of all positive integers smaller than or equal to n. For example factorial of 6 is 6*5*4*3*2*1 which is 720.
- A factorial is represented by a number and a ” ! ” mark at the end. It is widely used in permutations and combinations to calculate the total possible outcomes. A French mathematician Christian Kramp firstly used the exclamation.
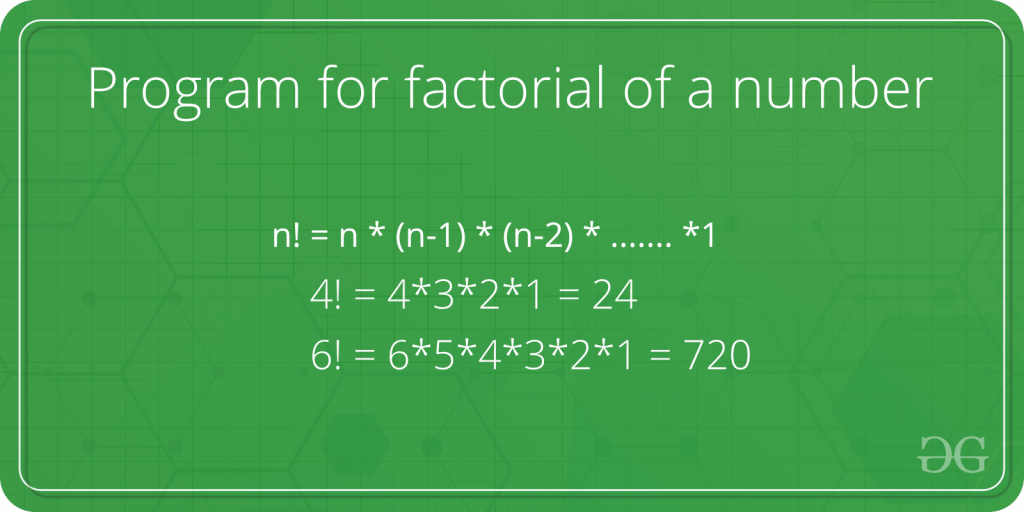
Let’s create a factorial program using recursive functions. Until the value is not equal to zero, the recursive function will call itself. Factorial can be calculated using the following recursive formula.
n! = n * (n – 1)!
n! = 1 if n = 0 or n = 1
Below is the implementation:
C++
#include <iostream>
using namespace std;
unsigned int factorial(unsigned int n)
{
if (n == 0 || n == 1)
return 1;
return n * factorial(n - 1);
}
int main()
{
int num = 5;
cout << "Factorial of "
<< num << " is " << factorial(num) << endl;
return 0;
}
|
C
#include <stdio.h>
unsigned int factorial(unsigned int n)
{
if (n == 0)
return 1;
return n * factorial(n - 1);
}
int main()
{
int num = 5;
printf ( "Factorial of %d is %d" , num, factorial(num));
return 0;
}
|
Java
class Test {
static int factorial( int n)
{
if (n == 0 )
return 1 ;
return n * factorial(n - 1 );
}
public static void main(String[] args)
{
int num = 5 ;
System.out.println( "Factorial of " + num
+ " is " + factorial( 5 ));
}
}
|
Python3
def factorial(n):
if n = = 0 :
return 1
return n * factorial(n - 1 )
num = 5 ;
print ( "Factorial of" , num, "is" ,
factorial(num))
|
C#
using System;
class Test {
static int factorial( int n)
{
if (n == 0)
return 1;
return n * factorial(n - 1);
}
public static void Main()
{
int num = 5;
Console.WriteLine( "Factorial of "
+ num + " is " + factorial(5));
}
}
|
PHP
<?php
function factorial( $n )
{
if ( $n == 0)
return 1;
return $n * factorial( $n - 1);
}
$num = 5;
echo "Factorial of " , $num , " is " , factorial( $num );
?>
|
Javascript
<script>
function factorial(n) {
if (n == 0) return 1;
return n * factorial(n - 1);
}
let num = 5;
document.write( "Factorial of " + num + " is " + factorial(num));
</script>
|
Output
Factorial of 5 is 120
Time Complexity: O(n)
Auxiliary Space: O(n)
Iterative Solution to find factorial of a number:
Factorial can also be calculated iteratively as recursion can be costly for large numbers. Here we have shown the iterative approach using both for and while loops.
Approach 1: Using For loop
Follow the steps to solve the problem:
- Using a for loop, we will write a program for finding the factorial of a number.
- An integer variable with a value of 1 will be used in the program.
- With each iteration, the value will increase by 1 until it equals the value entered by the user.
- The factorial of the number entered by the user will be the final value in the fact variable.
Below is the implementation for the above approach:
C++
#include <iostream>
using namespace std;
unsigned int factorial(unsigned int n)
{
int res = 1, i;
for (i = 2; i <= n; i++)
res *= i;
return res;
}
int main()
{
int num = 5;
cout << "Factorial of "
<< num << " is "
<< factorial(num) << endl;
return 0;
}
|
C
#include <stdio.h>
unsigned int factorial(unsigned int n)
{
int res = 1, i;
for (i = 2; i <= n; i++)
res *= i;
return res;
}
int main()
{
int num = 5;
printf (
"Factorial of %d is %d" , num, factorial(num));
return 0;
}
|
Java
class Test {
static int factorial( int n)
{
int res = 1 , i;
for (i = 2 ; i <= n; i++)
res *= i;
return res;
}
public static void main(String[] args)
{
int num = 5 ;
System.out.println(
"Factorial of " + num
+ " is " + factorial( 5 ));
}
}
|
Python3
def factorial(n):
res = 1
for i in range ( 2 , n + 1 ):
res * = i
return res
num = 5 ;
print ( "Factorial of" , num, "is" ,
factorial(num))
|
C#
using System;
class Test {
static int factorial( int n)
{
int res = 1, i;
for (i = 2; i <= n; i++)
res *= i;
return res;
}
public static void Main()
{
int num = 5;
Console.WriteLine(
"Factorial of " + num
+ " is " + factorial(5));
}
}
|
PHP
<?php
function factorial( $n )
{
$res = 1; $i ;
for ( $i = 2; $i <= $n ; $i ++)
$res *= $i ;
return $res ;
}
$num = 5;
echo "Factorial of " , $num , " is " ,
factorial( $num );
?>
|
Javascript
<script>
function factorial(n)
{
var res = 1, i;
for (i = 2; i <= n; i++)
res *= i;
return res;
}
var num = 5;
document.write( "Factorial of " + num + " is " + factorial(5));
</script>
|
Output
Factorial of 5 is 120
Time Complexity: O(n)
Auxiliary Space: O(1)
Approach 2: This example uses a while loop to implement the algorithm and find the factorial program.
C
#include <stdio.h>
unsigned int factorial(unsigned int n)
{
if (n == 0)
return 1;
int i = n, fact = 1;
while (n / i != n) {
fact = fact * i;
i--;
}
return fact;
}
int main()
{
int num = 5;
printf ( "Factorial of %d is %d" , num, factorial(num));
return 0;
}
|
C++
#include <iostream>
using namespace std;
unsigned int factorial(unsigned int n)
{
if (n == 0)
return 1;
int i = n, fact = 1;
while (n / i != n) {
fact = fact * i;
i--;
}
return fact;
}
int main()
{
int num = 5;
cout << "Factorial of "
<< num << " is "
<< factorial(num) << endl;
return 0;
}
|
Java
class Test {
static int factorial( int n)
{
if (n == 0 )
return 1 ;
int i = n, fact = 1 ;
while (n / i != n) {
fact = fact * i;
i--;
}
return fact;
}
public static void main(String[] args)
{
int num = 5 ;
System.out.println(
"Factorial of " + num
+ " is " + factorial( 5 ));
}
}
|
Python3
def factorial(n):
if (n = = 0 ):
return 1
i = n
fact = 1
while (n / i ! = n):
fact = fact * i
i - = 1
return fact
num = 5 ;
print ( "Factorial of" , num, "is" ,
factorial(num))
|
C#
using System;
class Test {
static int factorial( int n)
{
if (n == 0)
return 1;
int i = n, fact = 1;
while (n / i != n) {
fact = fact * i;
i--;
}
return fact;
}
public static void Main()
{
int num = 5;
Console.WriteLine(
"Factorial of " + num
+ " is " + factorial(5));
}
}
|
Javascript
<script>
function factorial(n) {
if (n == 0)
return 1;
let i = n, fact = 1;
while (Math.floor(n / i) != n) {
fact = fact * i;
i--;
}
return fact;
}
let num = 5;
document.write( "Factorial of "
+ num + " is "
+ factorial(num) + "<br>" );
</script>
|
Output
Factorial of 5 is 120
Time complexity: O(N)
Auxiliary Space: O(1)
Approach 3: A ternary operator can be thought of as a shorthand for an if…else statement. The conditions are provided, along with statements to be executed based on them. Here’s the program for factorial using a ternary operator.
C++
#include <iostream>
using namespace std;
int factorial( int n)
{
return (n == 1 || n == 0) ? 1 : n * factorial(n - 1);
}
int main()
{
int num = 5;
cout << "Factorial of " << num << " is " << factorial(num);
return 0;
}
|
C
#include <stdio.h>
int factorial( int n)
{
return (n == 1 || n == 0) ? 1 : n * factorial(n - 1);
}
int main()
{
int num = 5;
printf ( "Factorial of %d is %d" , num, factorial(num));
return 0;
}
|
Java
class Factorial {
int factorial( int n)
{
return (n == 1 || n == 0 ) ? 1 : n * factorial(n - 1 );
}
public static void main(String args[])
{
Factorial obj = new Factorial();
int num = 5 ;
System.out.println(
"Factorial of " + num
+ " is " + obj.factorial(num));
}
}
|
Python3
def factorial(n):
return 1 if (n = = 1 or n = = 0 ) else n * factorial(n - 1 )
num = 5
print ( "Factorial of" , num, "is" ,
factorial(num))
|
C#
using System;
class Factorial {
int factorial( int n)
{
return (n == 1 || n == 0) ? 1 : n * factorial(n - 1);
}
public static void Main()
{
Factorial obj = new Factorial();
int num = 5;
Console.WriteLine(
"Factorial of " + num
+ " is " + obj.factorial(num));
}
}
|
PHP
<?php
function factorial( $n )
{
return ( $n == 1 || $n == 0) ? 1:
$n * factorial( $n - 1);
}
$num = 5;
echo "Factorial of " , $num , " is " , factorial( $num );
?>
|
Javascript
<script>
function factorial(n)
{
return (n == 1 || n == 0) ? 1 : n * factorial(n - 1);
}
var num = 5;
document.write( "Factorial of " +num + " is " + factorial(num));
</script>
|
Output
Factorial of 5 is 120
Time complexity: O(n)
Auxiliary Space: O(n)
Problems in writing code of factorial
When the value of n changes increases by 1, the value of the factorial increases by n. So the variable storing the value of factorial should have a large size. Following is the value of n whose factorial can be stored in the respective size.
1. integer –> n<=12
2. long long int –> n<=19
From the above data, we can see that a very small value of n can be calculated because of the faster growth of the factorial function. We can however find the mod value of factorial of larger values by taking mod at each step.
The above solution cause overflow for large numbers. Please refer factorial of large number for a solution that works for large numbers.
Please write comments if you find any bug in the above code/algorithm, or find other ways to solve the same problem.
Share your thoughts in the comments
Please Login to comment...