How to use React with TypeScript ?
Last Updated :
05 Feb, 2024
TypeScript is a JavaScript variant with strongly bounded variable types. You need to tell the type of the created variable to the compiler before executing the code. ReactJS is a JavaScript library that is used to develop the User Interface of a website. You can use both of them together to combine their features and write clean and more efficient code.
Steps to setup the Project Environment
Step 1: Create a React app
You have to create a React app before start using TypeScript with React. Use the below command to create and move into your React project directory.
npx create-react-app project_name
cd project_name
Step 2: Install TypeScript
Now, you have to install TypeScript into your current project directory to use it with React using the below npm command.
npm install --save typescript
Step 3: Install React types definition
Install the latest type definition for the react and react-dom using the below command.
npm install @types/react @types/react-dom
Step 4: Install React dependencies
Now, install the React dependencies by running the below command into the Terminal of your IDE.
npm install
Step 5: Create files and Write code
Create different files with .tsx extension in your project directory and write the code using TypeScript and React.
Step 6: Run Project
Now, it’s time to run the application on the web browser to see the output of the written code using the below command.
npm run
Project Structure:
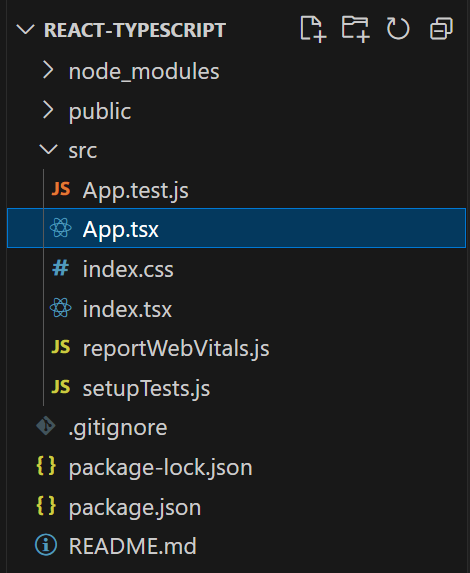
Example 1: The below code is a basic implementation of TypeScript with react to show some text on output screen.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import App from './App.tsx' ;
ReactDOM.render(
<React.StrictMode>
<App prop= "Geek" />
</React.StrictMode>,
document.getElementById( 'root' )
);
|
Javascript
import React from 'react' ;
interface AppProps {
prop: string;
};
const App: React.FC<AppProps> = ({ prop }) => {
return (
<div style={{textAlign: 'center' }}>
<h1 style={{color: 'green' }}>GeeksforGeeks</h1>
<h1>Hey {prop}!</h1>
<h2>Welcome to GeeksforGeeks</h2>
<h3>A Computer Science Portal.</h3>
</div>
);
};
export default App;
|
Output:
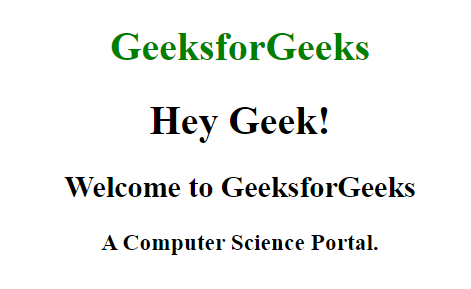
Example 2: The below code will show some advanced usage of the TypeScript with React.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import App from './App.tsx' ;
ReactDOM.render(
<React.StrictMode>
<App prop= "Geek" />
</React.StrictMode>,
document.getElementById( 'root' )
);
|
Javascript
import React, { useState } from 'react' ;
interface AppProps {
prop: string;
};
const App: React.FC<AppProps> = ({ prop }) => {
const [isHidden, setIsHidden] = useState( false )
function btnClickHandler() {
setIsHidden((prev) => !prev);
}
return (
<div style={{ textAlign: 'center' }}>
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<h1>Hey {prop}!</h1>
<h2>Welcome to GeeksforGeeks</h2>
<h3>A Computer Science Portal.</h3>
<button onClick={btnClickHandler}
style={
{
backgroundColor: "green" ,
color: '#fff' ,
padding: '15px' ,
cursor: 'pointer' ,
border: 'none' ,
borderRadius: '10px'
}}>
Click to toggle more page content
</button>
{
isHidden &&
<p>
This content is toggled dynamically using
click event with button.
</p>
}
</div>
);
};
export default App;
|
Output:
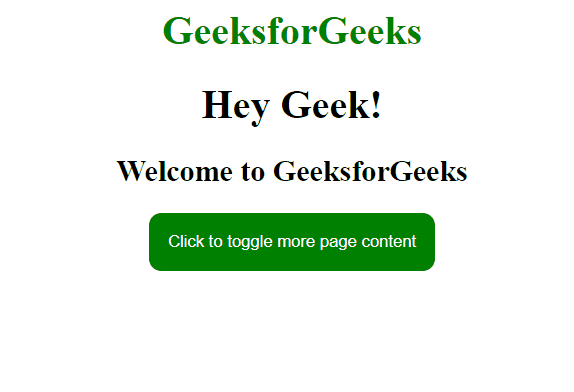
Share your thoughts in the comments
Please Login to comment...