How to Use React Suspense to Improve your React Projects ?
Last Updated :
15 Mar, 2024
Suspense is a React component used to suspend the rendering process to perform some asynchronous tasks like getting data from API or loading any other component lazily. In simple words, Suspense is like waiting for something to happen, where we are not sure when it will happen. Suspense is beneficial in code splitting and data fetching processes.
When Suspense suspends the rendering process, for that period we can show fallback UI like loading spinner or skeleton loading. In this article, we’ll see an example where Suspense is beneficial.
Code Splitting:
In Code Splitting, we can dynamically load files when required or data when required. In our case, we can load feeds, friends, and other pages only when we need them. So when we are loading files dynamically since this is an asynchronous task and takes time. So here comes the concept of Suspense, where we tell react that there is some code that will come in near future so for the time being show user some fallback UI.
Syntax:
<Suspense fallback={// show until component loads}>
// component to load
</Suspense>
If you’re confused, don’t worry. Let’s setup our project and then we will see code splitting using Suspense in practical.
Steps to Create React Application:
Create a React application and Navigate to the project directory the following command:
npx create-react-app my-project
cd my-project
Project Structure:
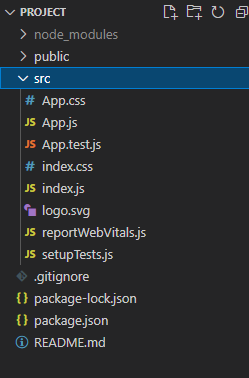
Project Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"redux": "^5.0.1"
}
Example: Below is the code example:
Javascript
// index.js
import React, {
Suspense,
lazy
} from "react";
import ReactDOM from "react-dom/client";
import App from "./App.jsx";
import "./index.css";
import {
RouterProvider,
createBrowserRouter
} from "react-router-dom";
// lazy loading components
const Home = lazy(() => import("./Home.jsx"));
const Feed = lazy(() => import("./Feed.jsx"));
const Friends = lazy(() => import("./Friends.jsx"));
const router = createBrowserRouter([
{
path: "/",
element: <App />,
children: [
{
path: "/",
element: (
// shows Loading... until Home loads
<Suspense fallback={
<h1>
Loading...
</h1>
}>
<Home />
</Suspense>
),
},
{
path: "/feed",
element: (
// shows Loading... until Feedback loads
<Suspense fallback={
<h1>
Loading...
</h1>
}>
<Feed />
</Suspense>
),
},
{
path: "/friends",
element: (
// shows Loading... until Friends loads
<Suspense fallback={
<h1>
Loading...
</h1>
}>
<Friends />
</Suspense>
),
},
],
},
]);
ReactDOM.createRoot(document.getElementById("root")).render(
<RouterProvider router={router}>
<App />
</RouterProvider>
);
Javascript
// NavBar.js
import {
NavLink
} from 'react-router-dom';
function Navbar() {
return (
<nav>
<ul>
<li><NavLink to="/">
Home
</NavLink></li>
<li><NavLink to="/feed">
Feed
</NavLink></li>
<li><NavLink to="/friends">
Friends
</NavLink></li>
</ul>
</nav>
);
}
export default Navbar;
Steps to run the Application:
npm start
Output:
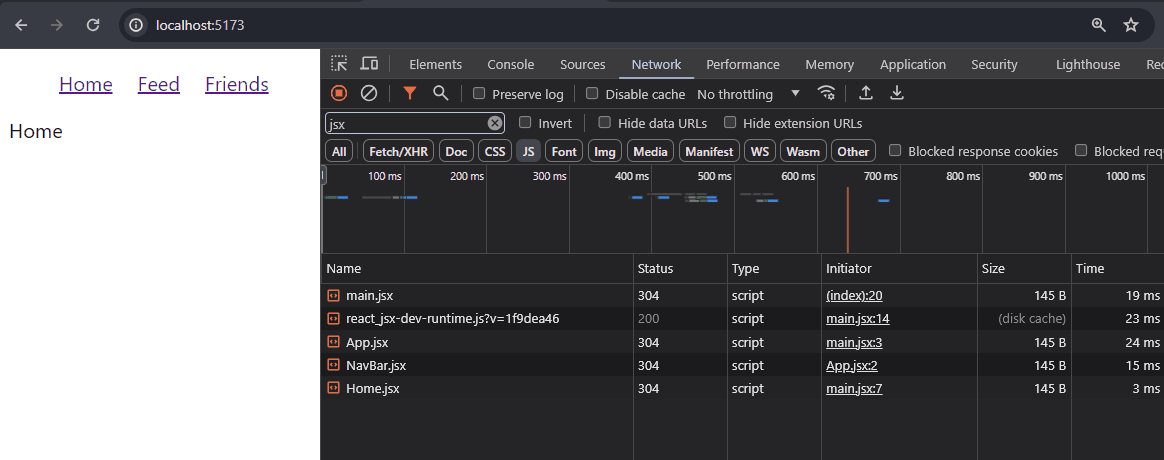
Output
Share your thoughts in the comments
Please Login to comment...