How to use refs in React with TypeScript ?
Last Updated :
15 Dec, 2023
In this example, we will learn how we can use the React refs with TypeScript. In React, refs are a special kind of syntax that can be used to access the DOM elements directly without the interference of React. We can get as well as set the values of the DOM elements using refs as we are used to doing this with pure JavaScript.
We can also use the TypeScript and React combined to write more clean and correct code. But, while using React with TypeScript, we need to save files with the “.tsx” extension and also need to keep the features of TypeScript in mind, we can not directly define refs in React while using it with TypeScript, we need to explicitly type the refs at the time of their declaration.
We will see the use of the React refs with TypeScript inside the Class-Based Components and Functional Components with the help of practical implementation using the code examples.
Using refs Inside the class-based component with TypeScript
In the class-based components, the React refs will be created using the React.createRef() method. But, we will use this method with an explicit type to specify the type of the ref using the <refType>.
Syntax:
private ref_name = React.createRef<refType>();
Example: The below code example will explain how you can create typed refs in React using TypeScript.
Javascript
import React, { Component } from 'react' ;
import { render } from 'react-dom' ;
class App extends Component {
private inputRef = React.createRef<HTMLInputElement>();
private divRef = React.createRef<HTMLDivElement>();
formSubmitHandler = (e) => {
e.preventDefault();
if (inputRef.current.value){
divRef.current.textContent =
"The entered value accessed using typed refs is: " +
inputRef.current.value;
}
else {
divRef.current.textContent =
"Input field can not be Empty!!" ;
}
};
render() {
return (
<div style={{textAlign: 'center' }}>
<h1 style={{color: 'green' }}>GeeksforGeeks</h1>
<form onSubmit={ this .formSubmitHandler}>
<input type= "text" ref={ this .inputRef} style={
{
padding: '8px' ,
borderRadius: '5px'
}} />
<br />
<br />
<button style={
{
color: '#fff' ,
background: 'green' ,
border: 'none' ,
padding: '10px' ,
cursor: 'pointer' ,
borderRadius: '5px'
}}>
Get Entered Value
</button>
</form>
<div style={{marginTop: '30px' }}
ref={ this .divRef}></div>
</div>
);
}
}
render(<App />, document.getElementById( 'root' ));
|
Output:
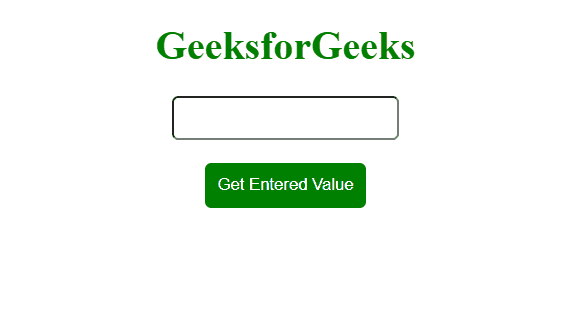
Using refs inside the functional components with TypeScript
The refs will be added using the useRef() hook when working with the functional components. We will use the same syntax <refType> to explicitly type the refs with a null value passed inside the parenthesis as parameter to set the initial value.
Syntax:
const refName = React.useRef<refType>(null);
Example: The below code example will explain how you can use the React refs with TypeScript inside the functional components.
Javascript
import React, { useRef } from 'react' ;
import { render } from 'react-dom' ;
const App = () => {
const inputRef = React.useRef < HTMLInputElement > ( null );
const divRef = React.useRef < HTMLDivElement > ( null );
const formSubmitHandler = (e) => {
e.preventDefault();
if (inputRef.current.value) {
divRef.current.textContent =
"The entered value accessed using typed refs is: " +
inputRef.current.value;
}
else {
divRef.current.textContent =
"Input field can not be Empty!!" ;
}
};
return (
<div style={{ textAlign: 'center' }}>
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<form onSubmit={formSubmitHandler}>
<input type= "text" ref={inputRef} style={
{
padding: '8px' ,
borderRadius: '5px'
}
} />
<br />
<br />
<button style={
{
color: '#fff' ,
background: 'green' ,
border: 'none' ,
padding: '10px' ,
cursor: 'pointer' ,
borderRadius: '5px'
}}>
Get Entered Value
</button>
</form>
<div style={{ marginTop: '30px' }} ref={divRef}></div>
</div>
);
}
|
Output:
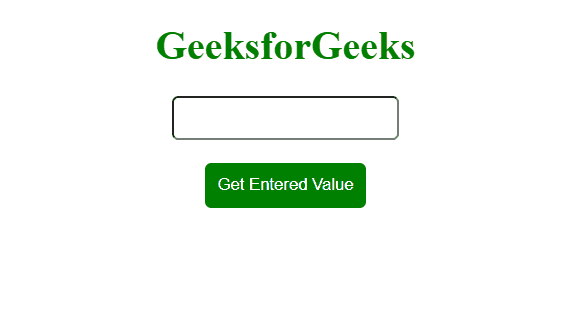
Share your thoughts in the comments
Please Login to comment...