How to use Pipes within ngModel on input Element in Angular ?
Last Updated :
05 Dec, 2023
The ngModel directive is a directive that is used to bind the values of the HTML controls (input, select, and textarea) or any custom form controls, and stores the required user value in a variable and we can use that variable whenever we require that value. In this article, we will learn about Using Pipes within ngModel on <input> Elements in Angular.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Creating pipe; go to cd/src
ng generate pipe geek
Project Structure
It will look like the following:
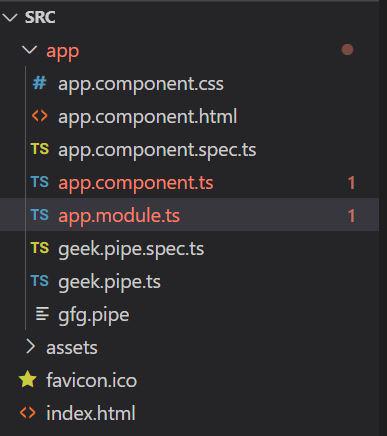
Example 1: In this example, we will use a capitalize pipe with ngModel that will help to capitalize the string that we enter in the input. Try to enter lowercase letters in the input, but our pipe will automatically make it in uppercase.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Using Pipes within ngModel
on INPUT Elements in Angular
</ h2 >
< input [ngModel]="msg | capitalize"
(ngModelChange)="msg=$event"
name = "name"
type = "text" />
< p >{{msg}}</ p >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { Router, NavigationEnd } from '@angular/router' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
msg: string = ""
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { FormsModule }
from '@angular/forms' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from '@angular/core' ;
@Pipe({
name: 'capitalize'
})
export class GeekPipe implements PipeTransform {
transform(val: string): string {
return val.toLocaleUpperCase()
}
}
|
Output:
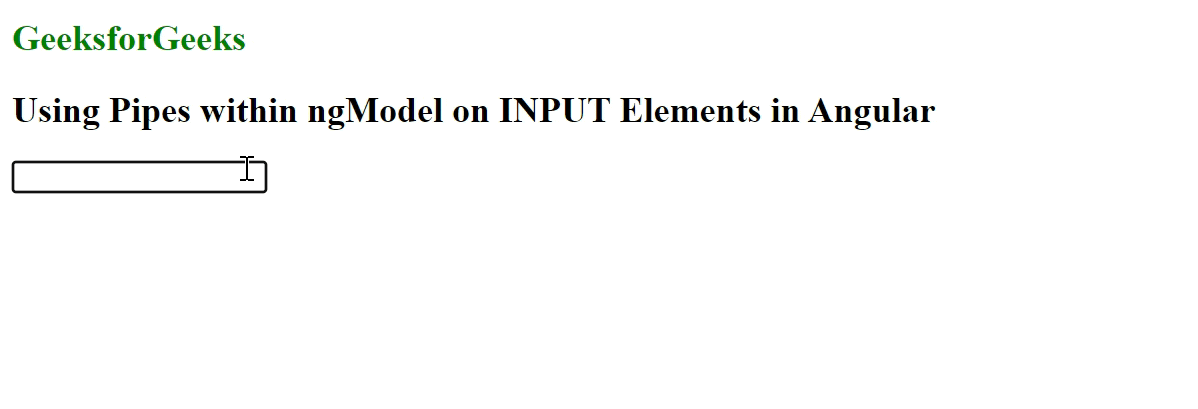
Example 2: In this example, we will see that we have used a pipe called lowercase, and it will not let you enter uppercase characters. Even if our capslock is on, it will convert our characters to lowercase.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Using Pipes within ngModel
on INPUT Elements in Angular
</ h2 >
< input [ngModel]="msg | lowercase"
(ngModelChange)="msg=$event"
name = "name"
type = "text" />
< p >{{msg}}</ p >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { Router, NavigationEnd } from '@angular/router' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
msg: string = ""
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { FormsModule }
from '@angular/forms' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from '@angular/core' ;
@Pipe({
name: 'lowercase'
})
export class GeekPipe implements PipeTransform {
transform(val: string): string {
return val.toLowerCase()
}
}
|
Output:
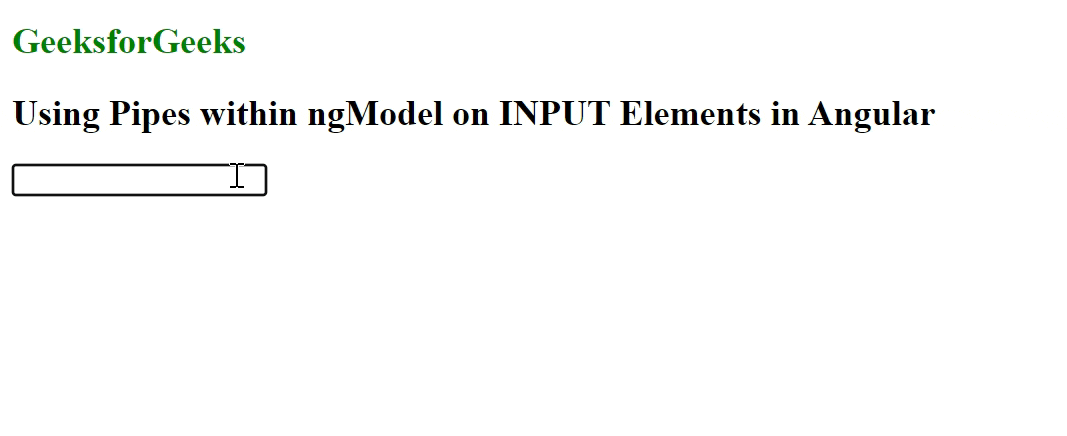
Share your thoughts in the comments
Please Login to comment...