What is pipe() function in Angular ?
Last Updated :
18 Mar, 2024
Angular stands out as a liked JavaScript framework for developing web applications. In Angular, the pipe()
function plays a vital role in transforming data within templates. It allows you to apply various transformations to data before displaying it in the view. In this article, we will explore more about the pipe() function in Angular.
What is the pipe() Function?
The pipe()
function in Angular is used to chain multiple operators together to transform data. It receives an input value works on it and gives back a modified result. You can recognize pipes in your template expressions by the pipe symbol ( | ).
Syntax of the pipe() function:
value | pipe1 | pipe2 | ... | pipeN
value
: The input value to be transformed.pipe1
, pipe2
, …, pipeN
: Operators or transforms to be applied to the input value.
Features of the pipe() Function
The pipe() function, in Angular provides a variety of functionalities that streamline and enhance data manipulation.
- Data Formatting : Pipes have applications, for organizing data like adjusting dates, numbers, currency and more.
- Data Filtering: Pipes are capable of sorting, searching, or presenting subsets of data by filtering data according to predefined criteria.
- Data Transformation: Data can be transformed by pipes using operations like title case, lowercase, and uppercase, among others.
- Localization: Pipes are handy for managing localization and internationalization of data like translating text or presenting dates, in formats.
Types of Pipes in Angular
1. Built-in Pipes
Angular comes equipped with built in pipes that handle a variety of common formatting duties.
<p>Today's date: {{ today | date:'mediumDate' }}</p>
Lets take a look, at some of the ones that are commonly used.
- DatePipe-The DatePipe is utilized for date formatting. It enables us to present dates, in styles like short, medium and full. For example we can utilize to exhibit the form of the date.
{{ myDate | date: "short" }}
- UpperCasePipe – This tool changes a text to capital letters. It requires a text, as input. Gives back the text in all capital letters.
{{ myString | uppercase }}
- LowerCasePipe – This particular pipe is utilized for changing a string to lowercase. Its functionality resembles that of the UpperCasePipe except it changes the string to lowercase instead.
{{ myString | lowercase }}
- CurrencyPipe – This tool helps to convert numbers into currency values. You input a number. It gives back a string showing the number, in the desired currency format.
{{ myNumber | currency: "USD" }}
2.. Custom Pipes
When the existing pre installed pipelines are not enough you can create your customized pipelines. Here is how you can set it up:
- Create a TypeScript class: Decorate the class with @Pipe.
- To use the PipeTransform interface you need to create a transform() method that takes the input value and any additional arguments you specify. In this transform() method you will define the logic, for altering the data.
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({ name: 'Geeks' })
export class GeeksPipe implements PipeTransform {
transform(value: string, limit: number = 10): string {
if (value.length > limit) {
return value.substring(0, limit) + '...';
}
return value;
}
}
<p>{{ longText | geeks:20 }}</p>
In Angular templates, you employ pipes using the pipe operator ( | ) within expressions:
{{ inputValue | pipeName:argument1:argument2:... }}
Steps to use pipe in Angular Application:
Step 1: Create a new angular project with the following command.
ng new my-pipe-demo
cd my-pipe-demo
Step 2: Generate a Component using the following command.
ng generate component display-date
Folder Structure:
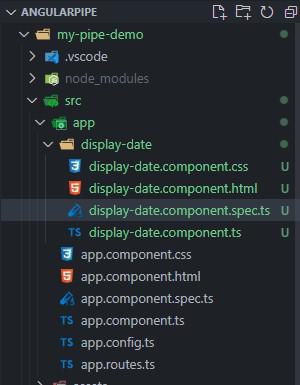
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/router": "^17.2.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.2.2",
"@angular/cli": "^17.2.2",
"@angular/compiler-cli": "^17.2.0",
"@types/jasmine": "~5.1.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.3.2"
}
Code Example: Modify the following files with these codes.
HTML
<!-- display-date.component.html -->
<p>Today's date (Default format): {{ today }}</p>
<p>Today's date (Short format): {{ today | date:'short' }}</p>
<p>Today's date (Custom format): {{ today | date:'MM/dd/yyyy' }}</p>
JavaScript
// display-date.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-display-date',
templateUrl: './display-date.component.html',
styleUrls: ['./display-date.component.css']
})
export class DisplayDateComponent implements OnInit {
today = Date.now();
}
To start the application run the following command.
ng serve
Output:
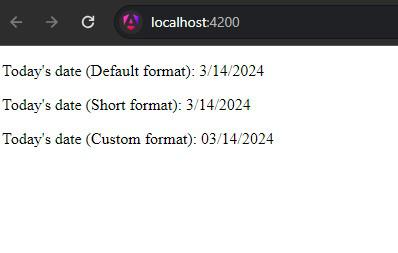
Final Output
Share your thoughts in the comments
Please Login to comment...