How to get comma separated KeyValue Pipe in Angular ?
Last Updated :
30 Nov, 2023
The KeyValue Pipe is an Angular built-in feature that transforms objects or maps into an array of key-value pairs. We can use the last variable of *ngFor directive to achieve the desired result. We will compare that if the element is last then add a comma. In this article, we will learn how to get a comma-separated KeyValue Pipe in Angular.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
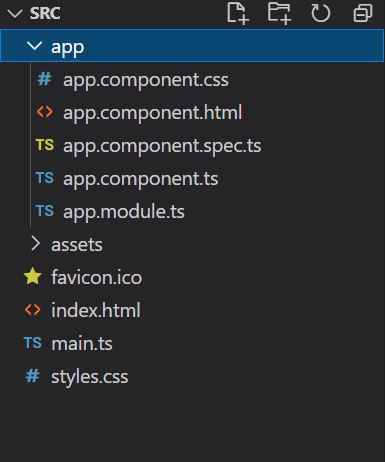
Example 1: In this example, we use the last variable of *ngFor directive to achieve the desired result. We will compare that if the element is last then add a comma.
HTML
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to get comma separated
KeyValue Pipe in Angular?
</ h2 >
< div * ngFor = "let item of gfg | keyvalue; last as isLast" >
< b >{{item.key}}:</ b > {{item.value}}
< span * ngIf = "!isLast" >, </ span >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any = {
"HTML" : "Hyper Text Markup Language" ,
"CSS" : "Cascade Style Sheet" ,
"XML" : "Xtensive Markup Language" ,
"JS" : "Javascript"
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
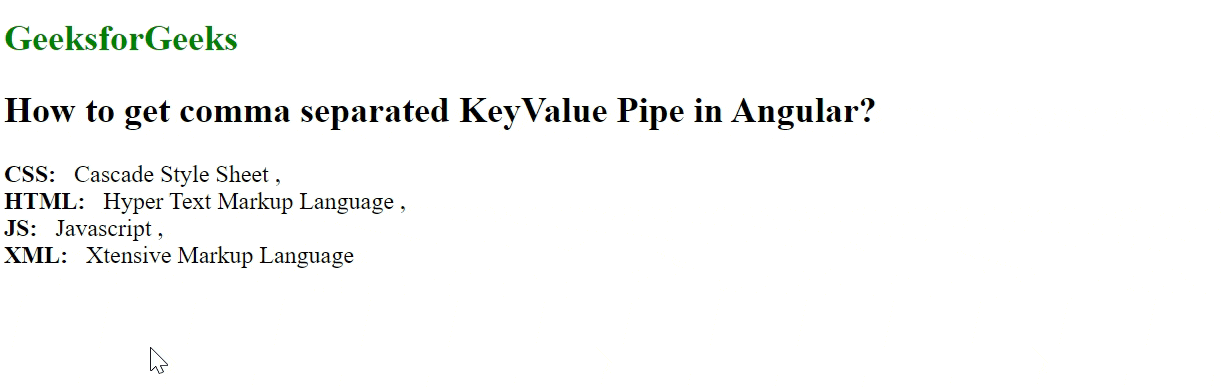
Example 2: In this example, we will comma separate the array of JSON objects using keyvalue. The keyvalue will help to get the keys and values and then we will add a comma
HTML
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to get comma separated
KeyValue Pipe in Angular?
</ h2 >
< div * ngFor = "let item of gfg | keyvalue ; last as isLast" >
< div * ngFor = "let element of display(item.value)|keyvalue" >
< b >Value: </ b >{{element.value}}
< span * ngIf = "!isLast" >, </ span >
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any =
[
{ "3" : "1700" },
{ "4" : "1900" },
{ "2" : "1400" },
{ "1" : "1300" },
{ "7" : "1200" },
{ "9" : "1800" },
{ "8" : "2100" },
{ "5" : "1100" }
]
display(obj: any) {
return Object.values(obj)
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
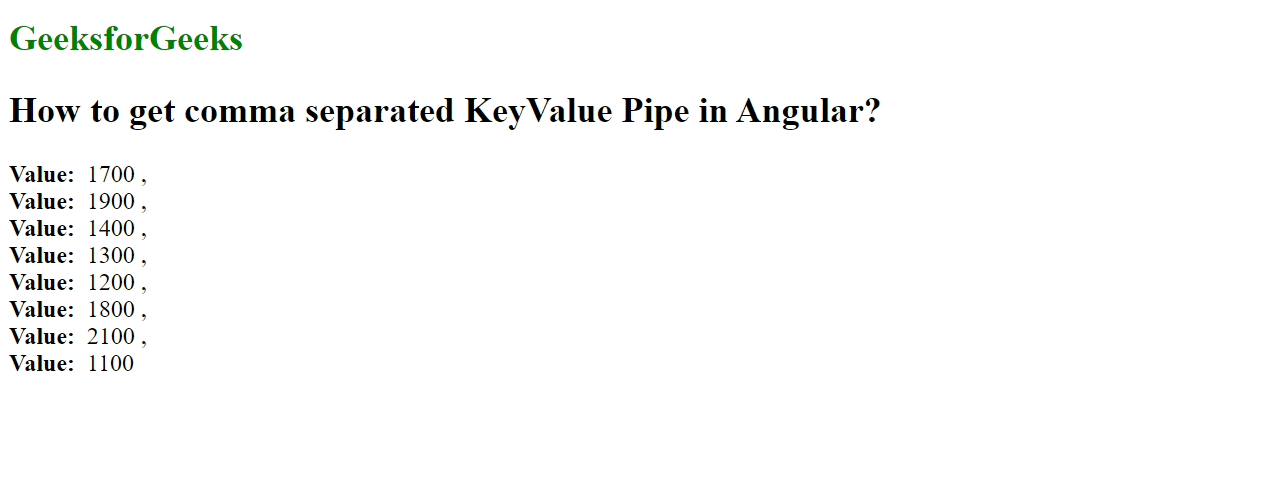
Share your thoughts in the comments
Please Login to comment...