How to test file uploads in Postman using Express?
Last Updated :
07 Dec, 2023
In web development, handling file uploads is a major requirement for various APIs. Postman is a powerful tool used for API testing, facilitates the process of testing file uploads. In this article we will see how we can test file upload with the help of Postman.
Prerequisites:
Steps to create Express JS Project:
Step 1: Create a new directory for your project. Open a terminal and run the following commands:
mkdir express-file-upload-example
cd express-file-upload-example
Step 2: Run the following command to initialize a new Node.js project and create a package.json file.
npm init -y
Step 3: Install Express and Multer (middleware for handling file uploads) by running the following commands.
npm install express multer
Step 4: Create an ‘uploads’ directory in your project to store uploaded files.
mkdir uploads
Step 5: Create a new file named app.js in your project directory.
Project Structure:
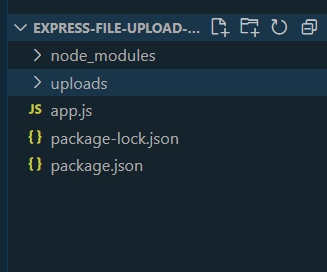
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"multer": "^1.4.5-lts.1"
}
Step 6: Add this code in your app.js file.
Javascript
const express = require( 'express' );
const multer = require( 'multer' );
const path = require( 'path' );
const app = express();
const port = 8000;
const storage = multer.diskStorage({
destination: function (req, file, cb) {
cb( null , 'uploads/' );
},
filename: function (req, file, cb) {
cb( null , Date.now() + path.extname(file.originalname));
}
});
const upload = multer({ storage: storage });
app.get( '/' , (req, res) => {
res.send( 'Hello from server' )
})
app.post( '/api/files/upload' , upload.single( 'file' ), (req, res) => {
const fileName = req.file.filename;
res.status(200).json({ message: `File uploaded successfully: ${fileName}` });
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Step 7: Open a terminal and run the following command to start your Express server.
node app.js
Your server should start and listen on port 8000.
Explanation:
In the above code we used the multer middleware to handle file uploads. The uploaded files are stored in the ‘uploads’ directory with a unique filename to avoid overwriting. The /api/files/upload endpoint is defined to handle POST requests for file uploads. The upload.single(‘file’) middleware specifies that we are expecting a single file with the field name ‘file’. The uploaded file information, such as the filename, can be accessed from “req.file”. The server responds with a JSON message indicating the success of the file upload.
Testing API using Postman.
Step 1: Open the Postman application or use the Postman web version (https://www.postman.com/).
Step 2: Create a new collection named GFG by clicking + icon on the collection section.
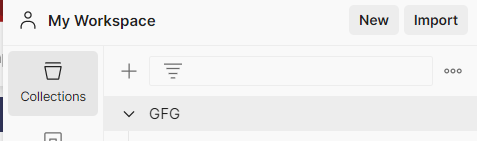
Step 3: Now click on the + icon in GFG to create a new POST request.
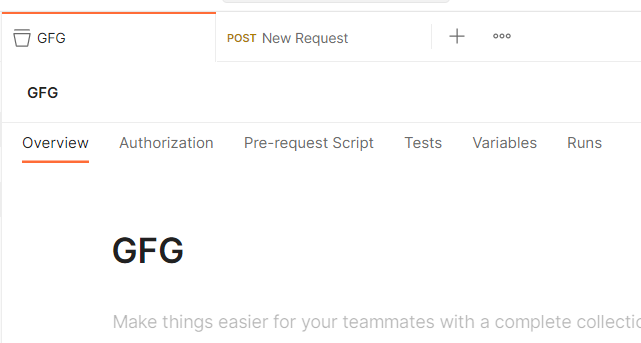
Step 4: Set the request type to POST and Enter the URL:
http://localhost:8000/api/files/upload

Step 5: Switch to Body tab and select form-data.
Step 6: Change the type of key to File and add key value pair, where the key is “file” and the value is the file you want to upload.
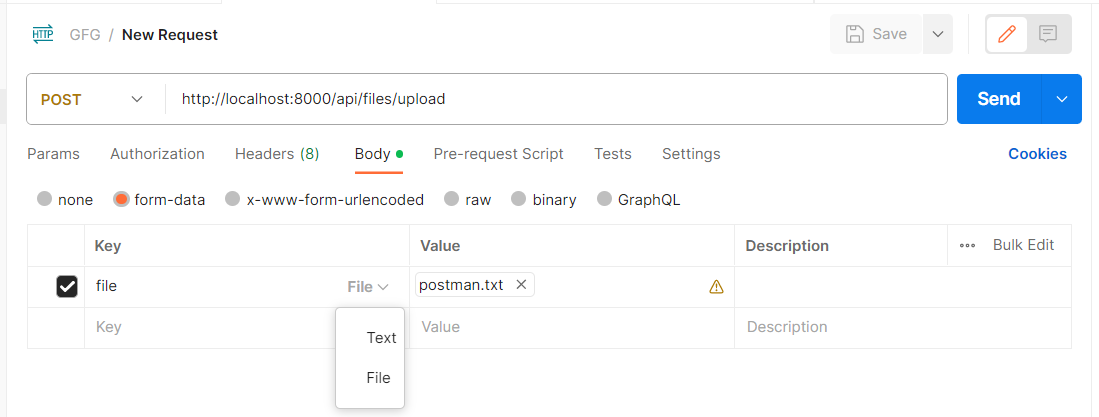
Step 7: Click on the send button to upload the file. You will see the message that file has uploaded.
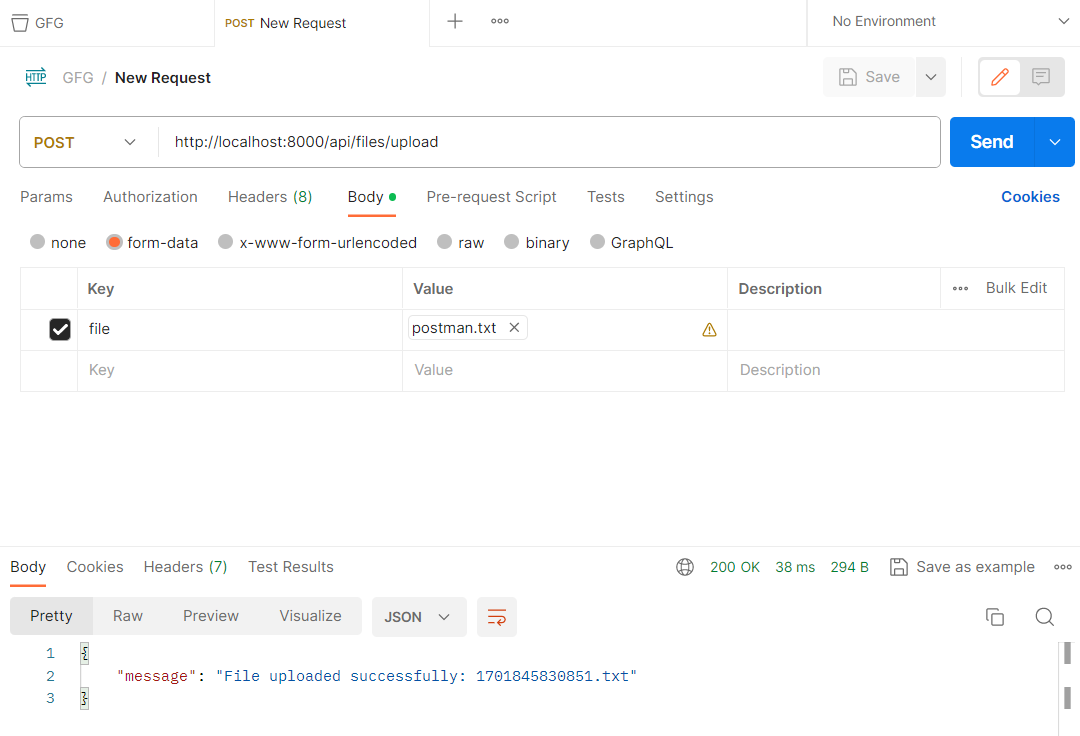
Share your thoughts in the comments
Please Login to comment...