Print hello world using Express JS
Last Updated :
28 Nov, 2023
In this article, we will learn how to print Hello World using Express jS. Express JS is a popular web framework for Node.js. It provides various features that make it easy to create and maintain web applications.
Express Js Program to Print Hello world:
“Hello, World!” in Express JS serves as an excellent entry point to familiarize yourself with the framework and embark on the exploration of its functionalities. Moreover, it provides a gratifying experience, allowing you to immerse yourself in the excitement of coding and initiate your path as an Express.js developer.
Below are the different types to print Hello World using express.js:
Approach 1: Using Basic Routes:
It is simple to print Hello World by defining a root URL (“/”). When a GET request is made to the root URL, the server responds with “Hello, World!”.
Example: Below is the example to print hello world using basic routes implementations.
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => {
res.send( '<h1> Hello, World! </h1>' );
});
app.listen(8000, () => {
console.log(`Server is listening at http:
});
|
Output:
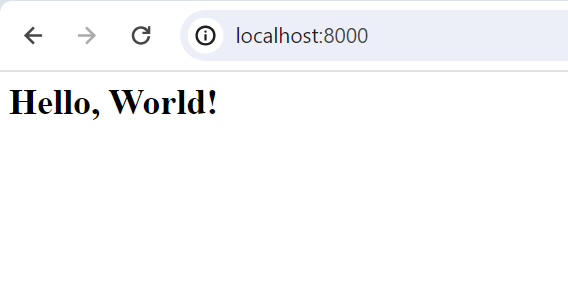
Output
Approach 2: Using Arrow Function:
Arrow functions in JavaScript offer a more streamlined way to write functions, featuring a simplified syntax, implicit return for single expressions, and lexical scoping of the `this` keyword.
Example: A basic route, employs an arrow function for a more succinct syntax. It establishes a route for the root URL and delivers the “Hello, World!” response in just one line.
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => res.send( '<h1>Hello, World!</h1>' ));
app.listen(8000, () => console.log( 'Server listening on port 8000' ));
|
Output:
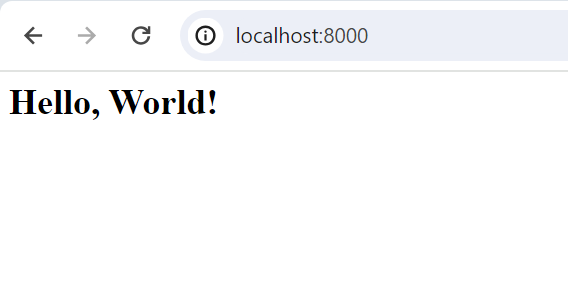
Output
Approach 3: Using Middleware:
Express middleware functions, distinct in their ability to interact with requests and responses, are employed in this case to handle all incoming requests and respond with a simple “Hello, World!” greeting.
Example: Below is the example to print hello world using middleware.
Javascript
const express = require( 'express' );
const app = express();
app.use((req, res) => {
res.send( '<h1>Hello, World!</h1>' );
});
app.listen(8000, () => console.log( 'Server listening on port 8000' ));
|
Output:
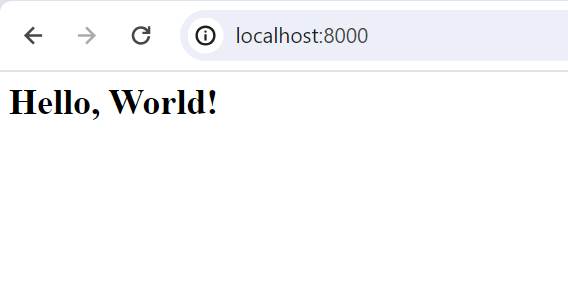
Output
Share your thoughts in the comments
Please Login to comment...