How to use third-party middleware in Express JS?
Last Updated :
12 Dec, 2023
Express JS is a robust web application framework of Node JS which has the capabilities to build web and mobile applications. Middleware is the integral part of the framework. By using the third party middleware we can add additional features in our application.
Prerequisites
What is Third-Party Middleware?
Third-party middleware are pre-built components used to add the functionality of an application. We need to install third-party middleware with the help of NPM and import it in our application
Steps to use third-party middleware in Express.js
Step 1: In the first step, we will create the new folder as third-middleware by using the below command in the VS Code terminal.
mkdir third-middle
cd third-middle
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init-y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
Step 4: As we need to use the third-party middleware, we need to install it using npm. So for this article, we will be using third-party middleware as a body-parser. So install it using the below command.
npm i body-parser
Project Structure:
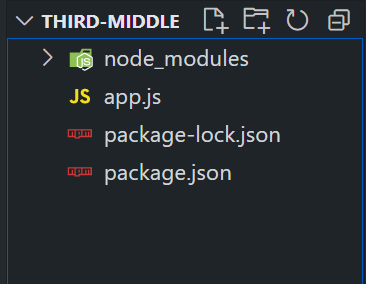
The updated dependencies in package.json file will look like:
"dependencies": {
"body-parser": "^1.20.2",
"express": "^4.18.2"
}
Example: Write the following code in App.js file
Javascript
const express = require( 'express' );
const parser = require( 'body-parser' );
const app = express();
const port = 3000;
app.use(parser.json());
app.post( '/api/data' , (req, res) => {
const reqData = req.body;
console.log( 'Received data:' , reqData);
res.status(200).json({ message: 'Data received successfully!' });
});
app.listen(port, () => {
console.log(`Server is running on http:
});
|
To run the application, we need to start the server by using the below command.
node app.js
Output:
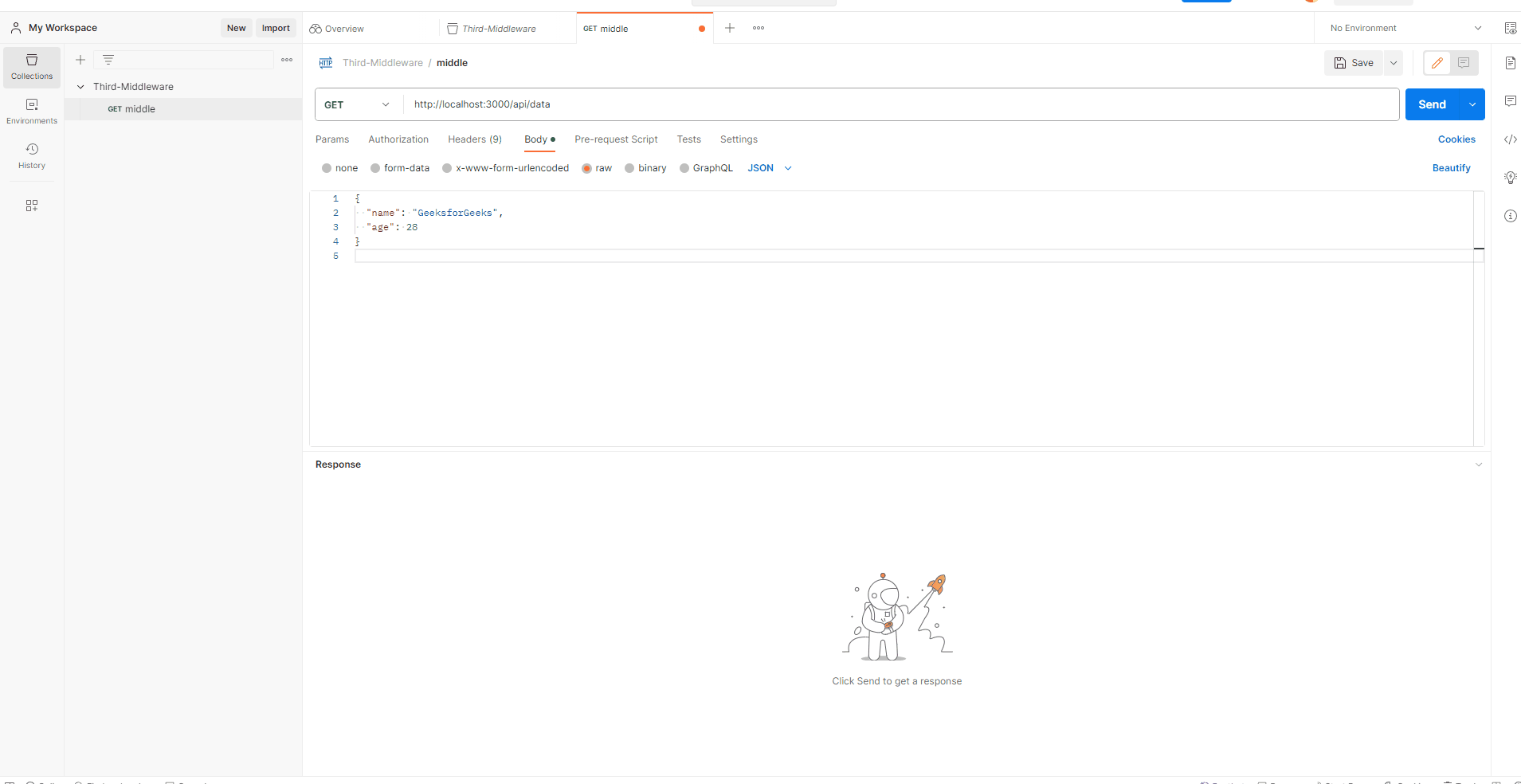
Explanation:
- In the above example, we have used third-party middleware as a body-parser. We have integrated it using ‘app.use()’.
- Then we configured the body-parser to parse incoming JSON requests in the handler ‘api/data‘.
- By using the req.body in the POST request handler we can access and process the parsed JSON data.
Share your thoughts in the comments
Please Login to comment...