How to implement useContext Hook in a Functional Component ?
Last Updated :
08 Mar, 2024
In React, the useContext hook is used to consume values from a context. Context in React is a way to share values like themes, authentication status, or any other global state between components without explicitly passing the props through each level of the component tree.
Syntax of useContext Hook:
const authContext = useContext(initialValue);
Steps to implement useContext Hook in React App:
Step 1: Create a React application using the following command:
npx create-react-app react-app
Step 2: After creating your project folder, i.e., react-app, move to it using the following command:
cd react-app
Project Structure: It will look like the following:
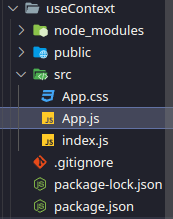
File Structure
The updated dependencies in the package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"run": "^1.4.0",
"web-vitals": "^2.1.4"
}
Approach to implement useContext Hook in Functional Component:
- MyContext is created using createContext.
- MyProvider is a component that wraps its children with MyContext.Provider and provides a value to the context.
- MyComponent is a functional component that uses the useContext hook to access the value from the context.
- Finally, in the App component, we use MyProvider to wrap MyComponent, establishing the context for the component tree.
Example: Below is the code showing the example of use of the useContext to display the value from the context.
CSS
body {
margin : 0 ;
padding : 0 ;
display : flex;
justify- content : center ;
align-items: center ;
text-align : center ;
height : 100 vh;
}
.main{
color : green ;
}
h 2 {
color : blue ;
}
|
Javascript
import React, { createContext, useContext } from 'react' ;
import './App.css'
const MyContext = createContext();
const MyProvider = ({ children }) => {
const value = "Hello! This is from Context!" ;
return <MyContext.Provider value={value}>{children}</MyContext.Provider>;
};
const MyComponent = () => {
const contextValue = useContext(MyContext);
return (
<div>
<h1 className= 'main' >GeeksForGeeks </h1>
<h2>Example of UseContext</h2>
<h1>{contextValue}</h1>
</div>
);
};
const App = () => {
return (
<MyProvider>
<MyComponent />
</MyProvider>
);
};
export default App;
|
To Run the Application, type the following command in terminal:
npm start
Output: Type the following link in your browser http://localhost:3000/
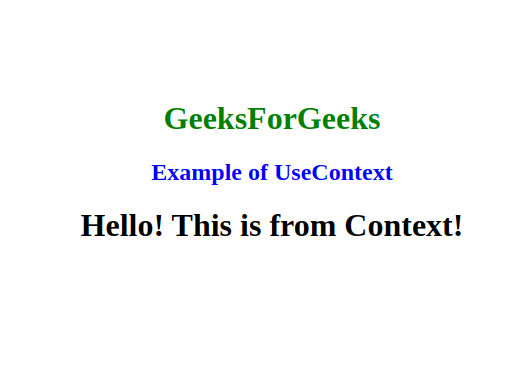
Implementing useContext Hook in a Functional Component
Share your thoughts in the comments
Please Login to comment...