How to handle data validation with React Hooks ?
Last Updated :
23 Feb, 2024
Data validation is a critical component of software development that ensures the accuracy, consistency, and suitability of the data that is being processed or stored in a system. It involves implementing checks and constraints to ensure that the data meets certain criteria or standards.
Key Points for Data Validation with React Hooks:
- useState Hook: Utilize the
useState
Hook to manage state in functional components.
- Data Validation in Setter Functions: Perform data validation checks within the setter function provided
useState
before updating the state.
- useEffect Hook: Employ the
useEffect
Hook to perform data validation when certain dependencies change, such as fetching data from an API.
- Custom Hooks: Encapsulate data validation logic into custom Hooks for reusability across multiple components.
- Validation Logic: Define specific validation logic according to your requirements, such as length checks, regex patterns, or custom validation functions.
Example: Below is an example of handling data validation with Hooks.
Javascript
import React, {
useState,
useEffect
} from 'react' ;
const App = () => {
const [name, setName] = useState( '' );
const [isValidName, setIsValidName] = useState( true );
const handleChange = (event) => {
const value = event.target.value;
setIsValidName(value.length >= 3);
setName(value);
};
useEffect(() => {
if (name === 'admin' ) {
alert( 'Admin name is not allowed!' );
}
}, [name]);
return (
<div>
<input
type= "text"
value={name}
onChange={handleChange}
style={{
borderColor:
isValidName ? 'initial' : 'red'
}}
/>
{!isValidName &&
<p>Name should be at
least 3 characters long.
</p>}
</div>
);
}
export default App;
|
Output:
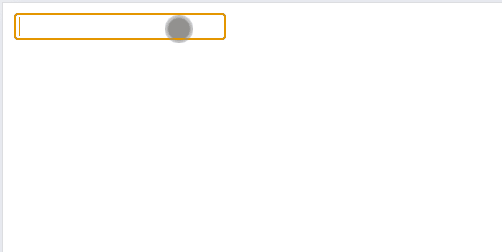
Output
Share your thoughts in the comments
Please Login to comment...