How to handle internationalization with Hooks in React ?
Last Updated :
28 Feb, 2024
Handling internationalization (i18n) involves using the state to manage the current language and updating the UI based on the selected language. This typically includes creating language files or dictionaries for each supported language and dynamically rendering the content based on the selected language in your components.
Step to Handling Internationalization with Hooks:
- Choose an i18n Library: Select an internationalization library for React. Popular choices include
react-i18next
and react-intl
. Install the chosen library using npm or yarn.
npm install i18next react-i18next
- Initialize the i18n Library: Initialize the i18n library in your application, typically in a top-level component or in your main entry file. Configure it with the supported languages and any necessary settings.
- Create a Custom Hook for Language Switching: Write a custom hook, let’s call it
useLanguage
, to handle language switching. This hook can include functions to change the current language.
- Use the Custom Hook in Components: Import and use the
useLanguage
hook in components where you want to manage language switching.
- Handle Translation in Components: Use the
useTranslation
hook from the i18n library to translate text within your components.
Example: Below is an example of handling internationalization with Hooks.
Javascript
import React from 'react' ;
import LanguageSwitcher
from './components/LanguageSwticher' ;
import ExampleComponent
from './components/Greeting' ;
import "../src/components/i18n"
const App = () => {
return (
<div>
<LanguageSwitcher />
<ExampleComponent />
</div>
);
};
export default App;
|
Javascript
import i18n from 'i18next' ;
import {
initReactI18next
} from 'react-i18next' ;
i18n
.use(initReactI18next)
.init({
resources: {
en: {
translation: {
greeting: 'Hello!' ,
},
},
fr: {
translation: {
greeting: 'Bonjour!' ,
},
},
},
lng: 'en' ,
fallbackLng: 'en' ,
interpolation: {
escapeValue: false ,
},
});
export default i18n;
|
Javascript
import {
useState
} from 'react' ;
import i18n from './i18n' ;
const useLanguage = () => {
const [currentLanguage, setCurrentLanguage] =
useState(i18n.language);
const changeLanguage = (language) => {
i18n.changeLanguage(language);
setCurrentLanguage(language);
};
return { currentLanguage, changeLanguage };
};
export default useLanguage;
|
Javascript
import React from 'react' ;
import useLanguage from './useLanguage' ;
const LanguageSwitcher = () => {
const { currentLanguage, changeLanguage } = useLanguage();
return (
<div>
<p>Current Language: {currentLanguage}</p>
<button onClick={() =>
changeLanguage( 'en' )}>
Switch to English
</button>
<button onClick={() =>
changeLanguage( 'fr' )}>
Switch to French
</button>
</div>
);
};
export default LanguageSwitcher;
|
CSS
.language-switcher-container {
display : flex;
flex- direction : column;
align-items: center ;
padding : 20px ;
border : 1px solid #ccc ;
border-radius: 8px ;
margin-bottom : 20px ;
}
button {
margin-top : 10px ;
padding : 8px 16px ;
font-size : 14px ;
cursor : pointer ;
}
button:hover {
background-color : #f0f0f0 ;
}
|
Step to run the App:
npm start
Output:
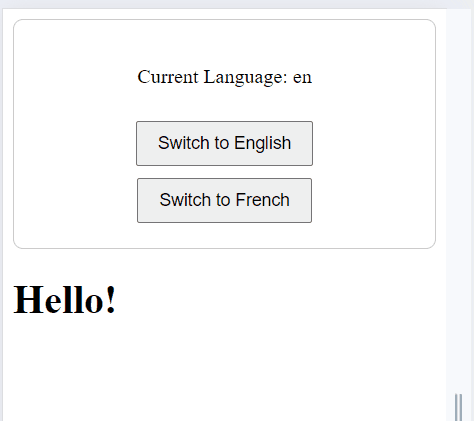
Output
Share your thoughts in the comments
Please Login to comment...