How to handle window resizing with Hooks?
Last Updated :
23 Feb, 2024
Handling window resizing with React Hooks involves using the useEffect
and useState
hooks to manage state and side effects in functional components. By utilizing these hooks, you can create components that dynamically respond to changes in the window size.
Handling window resizing with Hooks:
- State Management: Start by defining state variables to keep track of the window dimensions. For example, you might have
windowWidth
and windowHeight
.
- Event Listener: Inside the
useEffect
Hook, add an event listener for the resize
event on the window
object. This event will trigger whenever the window size changes.
- Update State: When the resize event occurs, update the state variables with the new window dimensions.
- Cleanup: It’s important to clean up the event listener when the component unmounts to avoid memory leaks. You can achieve this by returning a cleanup function from the
useEffect
Hook.
Example: Below is an example of handling window resizing with Hooks.
Javascript
import React, {
useState,
useEffect
} from 'react' ;
const App = () => {
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight
});
useEffect(() => {
const handleResize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight
});
};
window.addEventListener( 'resize' , handleResize);
return () => {
window.removeEventListener( 'resize' , handleResize);
};
}, []);
return (
<div>
<h2>Window Size</h2>
<p>Width: {windowSize.width}</p>
<p>Height: {windowSize.height}</p>
</div>
);
}
export default App;
|
Output:
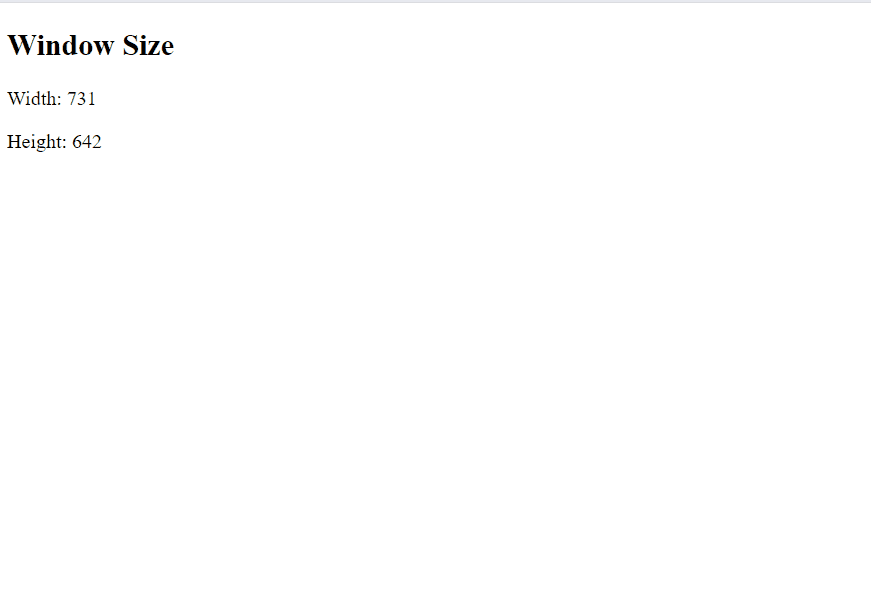
Output
Share your thoughts in the comments
Please Login to comment...