How to Generate Random Numbers in React Native ?
Last Updated :
12 Sep, 2023
Generating random numbers is a fundamental aspect of React Native development. It enables various functionalities like generating game elements, creating unique identifiers, and implementing randomized UI components. In this article, we are going to see how we can generate a random number by using React Native.
To generate random numbers in React Native, use `Math.random()` for a decimal value between 0 and 1. Combine it with `Math.floor()` to obtain whole numbers within a specified range.
Prerequisites:
Approa​ch:
- Specify the range (min and max).
- Generate a random decimal number (0-1) with
Math.random()
.
- Scale the number by multiplying it with the range difference.
- Shift the number by adding the minimum value.
- Round down to the nearest integer with
Math.floor()
.
- Store or use a random number.
Step 1: Set Up the Development Environment​
Install Expo CLI globally by running this command:
npm install -g expo-cli​
Step 2: Create React Native Application With Expo CLI
Create a new react native project with Expo CLI by using this command:
expo init randomnumber-react
Step 3: ​Navigate to project directory by using this command:
cd randomnumber-react
Project Structure:
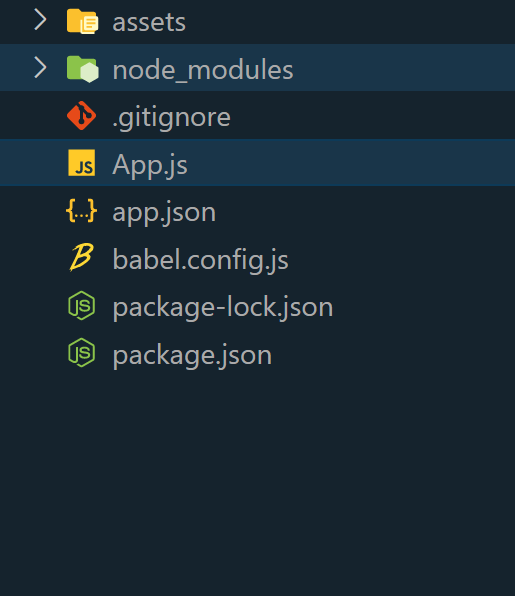
Example: In this example, the state initializes with a null randomNumber. Pressing the “Random Number” button triggers generateRandomNumber, generating a random number within a range. Updating the state triggers re-rendering. Basic styling is applied with StyleSheet.
- App.js: This file imports the react native basic components and exports it.
Javascript
import React from 'react' ;
import { View, TouchableOpacity, Text,
StyleSheet } from 'react-native' ;
class App extends React.Component {
constructor(props) {
super (props);
this .state = {
randomNumber: null ,
};
}
generateRandomNumber = () => {
const min = 1;
const max = 100;
const randomNumber =
Math.floor(Math.random() * (max - min + 1)) + min;
this .setState({ randomNumber });
};
render() {
return (
<View style={styles.container}>
<Text style={styles.heading}>
Geeksforgeeks ||
Generate Random Number In React Native
</Text>
<TouchableOpacity
style={styles.button}
onPress={ this .generateRandomNumber}>
<Text style={styles.buttonText}>
Random Number
</Text>
</TouchableOpacity>
{
this .state.randomNumber &&
<Text style={styles.randomNumber}>
Number is: { this .state.randomNumber}
</Text>
}
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
},
button: {
backgroundColor: 'green' ,
paddingHorizontal: 20,
paddingVertical: 10,
borderRadius: 5,
marginBottom: 20,
},
buttonText: {
color: 'white' ,
fontSize: 18,
fontWeight: 'bold' ,
},
randomNumber: {
fontSize: 24,
fontWeight: 'bold' ,
color: 'red' ,
},
heading: {
fontSize: 20,
marginBottom: 20,
color: "green" ,
fontWeight: 'bold' ,
}
});
export default App;
|
Step 5: To run the React Native application, use the following command in the Terminal/CMD:
npx expo start
To run on Android:
npx react-native run-android
To run on ios:
npx react-native run-ios
Output:
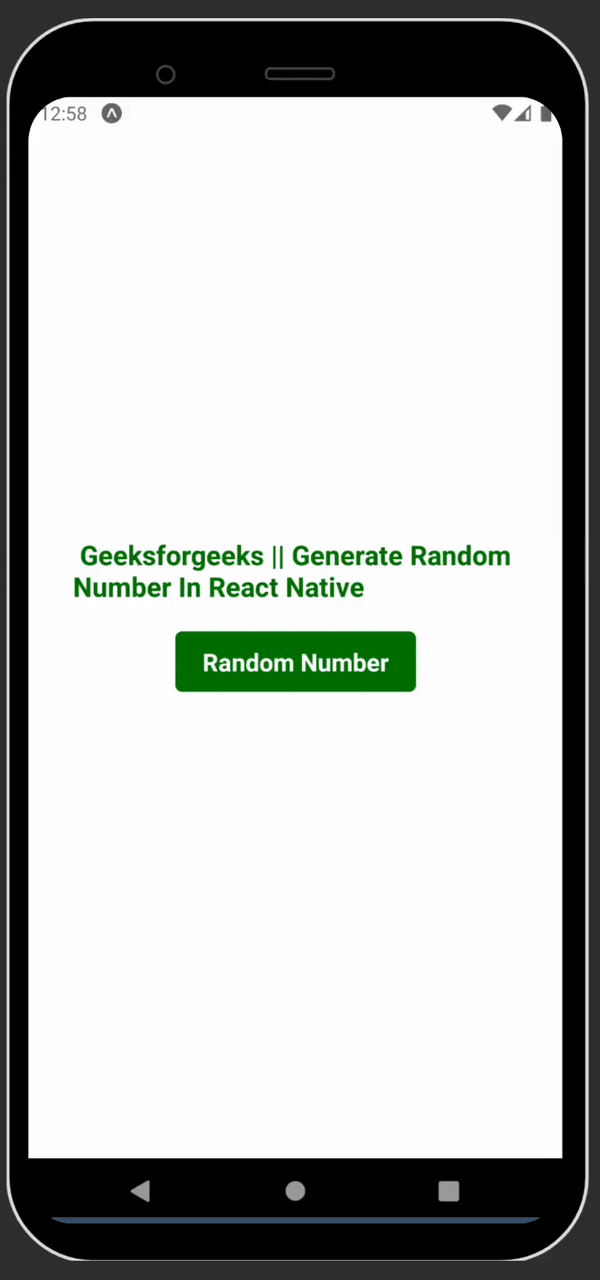
Share your thoughts in the comments
Please Login to comment...