Find what caused Possible unhandled promise rejection in React Native ?
Last Updated :
23 Jan, 2023
In this article, we will check out the `Possible Unhandled Promise Rejection` error in react native.
Installation: Follow the steps below to create your react-native project
Step 1: Open your terminal and run the below command.
npx create-expo-app project-name
Step 2: Now go into the created folder and start the server by using the following command.
cd "project-name"
Step 3: To start the react-native program, execute this command in the terminal of the project folder.
npx expo start
Then press ‘a’ or ‘i’ to open it in your android or ios emulator respectively.
Project Structure:
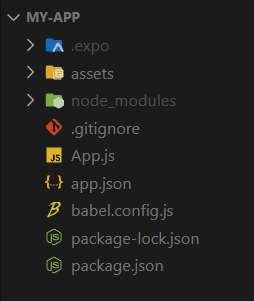
Â
Error Shown: Below is a code of error that can occur in a React Native app when a promise is rejected but the rejection is not handled. The error message is shown in the image below.
Example: The below code will show the error and problem:Â
Javascript
import React, { useState } from "react" ;
import { View, Button, Text } from "react-native" ;
import { StatusBar } from "expo-status-bar" ;
export default function App() {
const [data, setData] = useState();
function onPress() {
.then((response) => response.json())
.then((data) => {
setData(JSON.stringify(data));
})
}
return (
<View style={{ flex: 1, alignItems: "center" ,
justifyContent: "center" }}>
<StatusBar style= "auto" />
<Button title= "Load data"
onPress={onPress} />
<Text>{data}</Text>
</View>
);
}
|
Output:
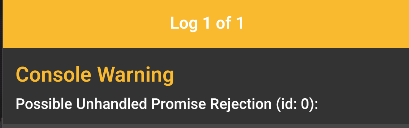
Â
If you are getting a “Possible unhandled promise rejection” warning in your React Native app, it means that a promise was rejected, but the rejection was not handled. Promises in JavaScript are used to handle asynchronous operations, and they can either be resolved or rejected. When a promise is rejected, you can handle the rejection by using the catch method.
To find the cause of this error, you can try the following steps:Â
One possible cause of this error is the use of .catch() statements in your code that are not handling promise rejections. To fix this, you can add a .catch() statement to your promises and include a rejection handler to handle any errors that might occur.
Another possible cause of this error is syntax errors or typos in your code. To fix this, you can carefully review your code and look for any mistakes that might be causing the promise rejection.
Example: Here is an example of how you can use .catch() to handle a possible unhandled promise rejection in a React Native app:
Javascript
import React, { useState } from "react" ;
import { View, Button, Text } from "react-native" ;
import { StatusBar } from "expo-status-bar" ;
export default function App() {
const [data, setData] = useState();
function onPress() {
.then((response) => response.json())
.then((data) => {
setData(JSON.stringify(data));
})
. catch ((error) => {
console.error(error);
});
}
return (
<View style={{ flex: 1, alignItems: "center" ,
justifyContent: "center" }}>
<StatusBar style= "auto" />
<Button title= "Load data"
onPress={onPress} />
<Text>{data}</Text>
</View>
);
}
|
In this example, the onPress function is called when the button is clicked. The fetchData function makes an HTTP request using the fetch function and waits for the response. If the request is successful, the response data is stored in the data state using the setData function. If the request fails, the rejection is caught by the catch function, and the error message is logged to the console.
Error after adding “.catch()”:
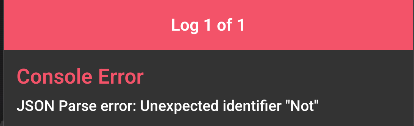
Error message
Now the function will throw more descriptive errors which you can use to debug the code.
In conclusion, the “Possible unhandled promise rejection” error can be a frustrating problem to deal with in a React Native app. However, by carefully reviewing your code, using debugging tools, and checking for issues with third-party libraries, you can often identify and fix the source of the problem.
Share your thoughts in the comments
Please Login to comment...