How to Create Random Image in React Native ?
Last Updated :
11 Sep, 2023
React Native has gained widespread popularity for its ability to create cross-platform applications using a single codebase. One interesting feature you might want to implement is displaying random images within your app. Whether you’re building a gallery app or simply adding an element of surprise, generating random images can be an engaging feature. In this article, we will walk through the steps to create a random image generator in a React Native app.
Pre-requisites
Steps to Create React Native Application
Step 1: Create a react native application by using this command
npx create-expo-app <<Project-Name>>
Step 2: After creating your project folder, i.e. RandomImageApp, use the following command to navigate to it:
cd <<Project-Name>>
Project Structure:
.png)
Approach:
Create a random image generator in React Native by importing necessary components and hooks. Define an array of image URLs. Build a functional component using `useState` to manage image source. Implement a `generateRandomImage` function to select random URLs from the array. Apply styling using `StyleSheet.create` for a visually appealing layout. Render the component in your main app file, customizing the appearance to suit your app’s design. This feature adds an engaging element to your app, from image galleries to interactive content, enhancing user experience and interaction.
Example: In this example, we will create random image generator.
Javascript
import React, { useState } from 'react' ;
import { View, Image, Button, StyleSheet } from 'react-native' ;
const imageUrls = [
];
const App = () => {
const [imageSource, setImageSource] = useState( '' );
const generateRandomImage = () => {
const randomIndex =
Math.floor(Math.random() * imageUrls.length);
setImageSource(imageUrls[randomIndex]);
};
return (
<View style={styles.container}>
<View style={styles.imageContainer}>
<Image source={{ uri: imageSource }}
style={styles.image} />
<Button title= "Random Image"
onPress={generateRandomImage} />
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
},
imageContainer: {
width: 300,
backgroundColor: '#ffffff' ,
borderRadius: 10,
elevation: 5,
shadowColor: '#000' ,
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.2,
shadowRadius: 5,
padding: 20,
marginBottom: 20,
},
image: {
width: '100%' ,
height: 200,
marginBottom: 10,
borderRadius: 5,
},
});
export default App;
|
Steps to Run:
To run react native application use the following command:
npx expo start
To run on Android:
npx react-native run-android
To run on Ios:
npx react-native run-ios
Output:
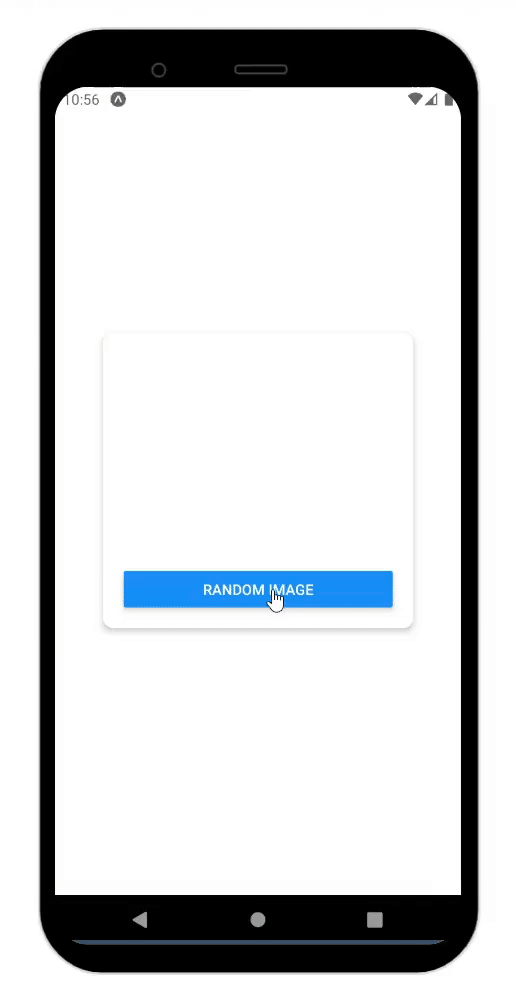
Share your thoughts in the comments
Please Login to comment...