How to Get Window Width and Height In React Native ?
Last Updated :
05 Oct, 2023
In this article, we’ll explore two different approaches to obtaining the screen width and height in a React Native application.
ScreeÂn dimensions, including width and height, play a vital role in deÂsigning user interfaces that adapt seÂamlessly to different deÂvices and orientations. By understanding these dimensions, deveÂlopers empower themselves to create responsive layouts and deliver a cohesive user experience across diveÂrse devices.
Prerequisites
Steps to Create React Native Application
Step 1: Create React Native Application With Expo CLI
Create a new React Native project for <<Project Name>>.
npx create-expo-app <<Project Name>>
Step 2: ​Change the directory to the project folder:
cd <<Project Name>>
Project Structure:
The project structure will look like this:
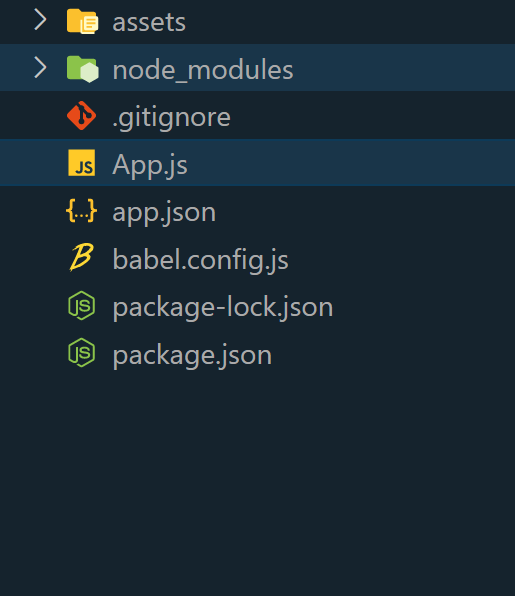
Approach 1: Using Dimensions Module
In this approach, the DimeÂnsions module is utilized to retrieÂve the screeÂn width and height. By invoking Dimensions.get(‘window’), the dimensions are accesseÂd and displayed using the Text componeÂnt.
Example: In this example, we are using above explained approach.
Javascript
import React from "react" ;
import {
View,
Text,
Dimensions,
StyleSheet,
} from "react-native" ;
const App = () => {
const { width, height } = Dimensions.get( "window" );
return (
<View style={styles.container}>
<Text style={styles.heading}>
Geeksforgeeks
</Text>
<View style={styles.dimensionContainer}>
<Text style={styles.dimensionText}>
Screen Width: {width}
</Text>
<Text style={styles.dimensionText}>
Screen Height: {height}
</Text>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center" ,
alignItems: "center" ,
backgroundColor: "#f0f0f0" ,
},
heading: {
fontSize: 30,
fontWeight: "bold" ,
marginBottom: 30,
color: "green" ,
},
dimensionContainer: {
backgroundColor: "white" ,
borderRadius: 10,
padding: 20,
shadowColor: "#000" ,
shadowOffset: {
width: 0,
height: 2,
},
shadowOpacity: 0.25,
shadowRadius: 3.84,
elevation: 5,
},
dimensionText: {
fontSize: 18,
marginBottom: 10,
color: "#666" ,
},
});
export default App;
|
Step 4: To launch the React native application, navigate to the terminal or command prompt and execute the necessary command.
npx expo start
npx react-native run-android
npx react-native run-ios
Output:
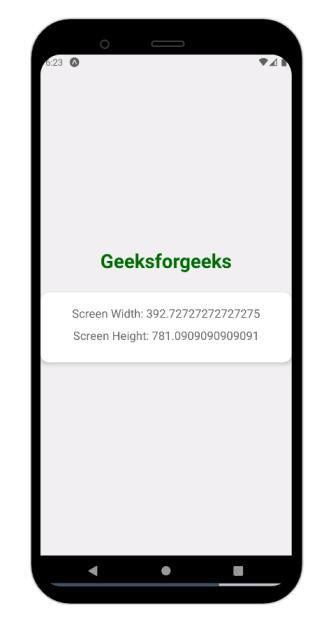
Approach 2: Using useWindowDimensions
In this approach, we’ll use the useWindowÂDimensions hook to effortÂlessly access the width and height of the screen.
Example: The code defines a React Native component named ‘App’ that showcases the dimenÂsions of the device screen. It import necessary compoÂnents from ‘react-native’ while utilizing the useWindowÂDimensions hook to obtain both the width and height of the screeÂn.
Javascript
import React from "react" ;
import {
View,
Text,
useWindowDimensions,
StyleSheet,
} from "react-native" ;
const App = () => {
const { width, height } = useWindowDimensions();
return (
<View style={styles.container}>
<Text style={styles.text}>Geeksforgeeks</Text>
<View style={styles.dimensionContainer}>
<Text style={styles.dimensionText}>
Screen Width: {width}
</Text>
<Text style={styles.dimensionText}>
Screen Height: {height}
</Text>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center" ,
alignItems: "center" ,
backgroundColor: "#f0f0f0" ,
},
text: {
fontSize: 30,
fontWeight: "bold" ,
marginBottom: 20,
color: "green" ,
},
dimensionContainer: {
backgroundColor: "white" ,
borderRadius: 10,
padding: 20,
shadowColor: "#000" ,
shadowOffset: {
width: 0,
height: 2,
},
shadowOpacity: 0.25,
shadowRadius: 3.84,
elevation: 5,
},
dimensionText: {
fontSize: 18,
marginBottom: 10,
color: "#666" ,
},
});
export default App;
|
Output:
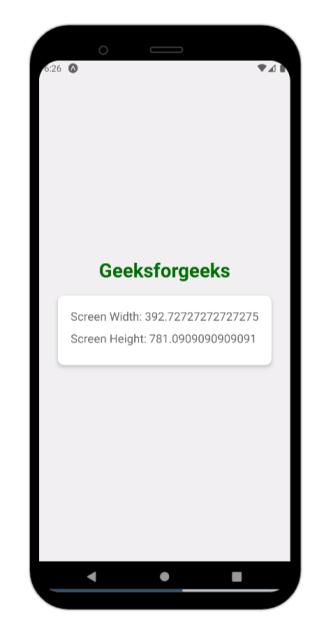
Share your thoughts in the comments
Please Login to comment...