Create a Random Quote Generator using React-Native
Last Updated :
04 Dec, 2023
React Native is the most flexible and powerful mobile application development framework, which has various features embedded into it. Using this framework, we can create different interactive applications. Creating a Random Quote Generator using React Native is one of the interactive project which utilizes the API to generate the random quote and present it to the user.
In the application, the user can click the button to fetch the random quote from the API. Along with this, the user can click on the copy button to copy the quote to the clipboard for further use. We have styled the application using CSS styling embedded in the JS file
Preview of final output: Let us have a look at how the final output will look like.
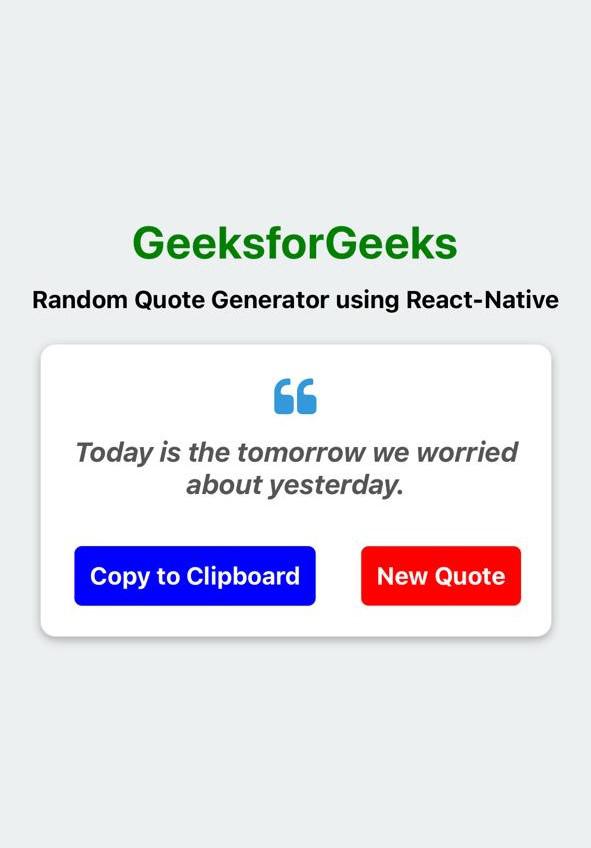
Prerequisites:
Approach:
The project code is completely written in React Native language which allows the user to look for Random quotes and copy them for further use. We have used the useState hook to manage the state of the application and the useEffect hook is used to fetch a random quote from the API when the component mounts. It also ensures that the fetchQuoteFunction is called only once when the component is initially rendered. The application has the functionality to copy the quote to the clipboard and use it in different sources.
Steps to Create React Native Application:
Step 1: Create a react native application by using this command:
npx create-expo-app random-quote
Step 2: After creating your project folder, i.e. random-quote use the following command to navigate to it:
cd random-quote
Project Structure:
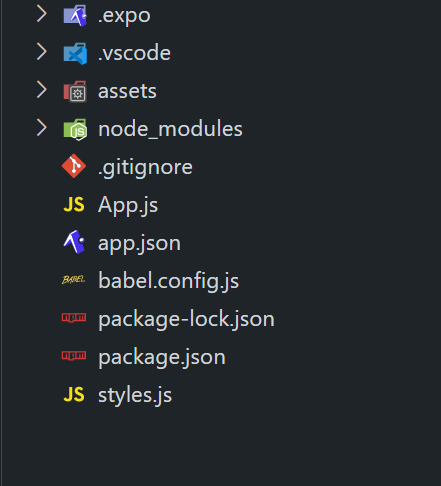
The updated dependencies in package.json file will look like:
"dependencies": {
"@expo/webpack-config": "^19.0.0",
"@react-native-async-storage/async-storage": "^1.21.0",
"@react-native-clipboard/clipboard": "^1.12.1",
"@react-navigation/native": "^6.1.9",
"@react-navigation/stack": "^6.3.20",
"axios": "^1.6.2",
"expo": "~49.0.15",
"expo-av": "^13.6.0",
"expo-status-bar": "~1.6.0",
"react": "18.2.0",
"react-native": "0.72.6",
"react-native-clipboard": "^0.0.5",
"react-native-vector-icons": "^10.0.2",
"react-native-web": "~0.19.6"
}
Example: Below is the implementation of the above-discussed approach
Javascript
import React, { useState, useEffect } from "react" ;
import {View,Text,TouchableOpacity,ScrollView,Platform,Clipboard} from "react-native" ;
import Icon from "react-native-vector-icons/FontAwesome" ;
import axios from "axios" ;
import AsyncStorage from "@react-native-async-storage/async-storage" ;
import styles from "./styles" ;
const App = () => {
const [quote, setQuote] = useState( "" );
useEffect(() => {
fetchQuoteFunction();
}, []);
const fetchQuoteFunction = async () => {
try {
const quo = res.data;
const idx = Math.floor(Math.random() * quo.length);
const randomQuo = quo[idx].text;
await AsyncStorage.setItem( "lastQuote" , randomQuo);
setQuote(randomQuo);
} catch (error) {
console.error( "Error fetching quotes:" , error.message);
}
};
const newQuoteFunction = () => {
fetchQuoteFunction();
};
const cpyFunction = () => {
try {
Clipboard.setString(quote);
alert( "Quote copied to clipboard!" );
} catch (error) {
console.error( "Error copying to clipboard:" , error.message);
}
};
return (
<View style={styles.container}>
<ScrollView contentContainerStyle={styles.scrollViewContent}>
<Text style={styles.geeksText}>GeeksforGeeks</Text>
<Text style={styles.header2}>
Random Quote Generator using React-Native
</Text>
<View style={styles.card}>
<Icon
name= "quote-left"
size={30}
color= "#3498db"
style={styles.quoteIcon}
/>
<Text style={styles.quoteText}>{quote}</Text>
<View style={styles.cardButtons}>
<TouchableOpacity
style={styles.copyButton}
onPress={cpyFunction}
>
<Text style={styles.copyButtonText}>
Copy to Clipboard
</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.newQuoteButton}
onPress={newQuoteFunction}
>
<Text style={styles.buttonText}>New Quote</Text>
</TouchableOpacity>
</View>
</View>
</ScrollView>
</View>
);
};
export default App;
|
Javascript
import { StyleSheet } from "react-native" ;
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#ecf0f1" ,
paddingHorizontal: 20,
justifyContent: "center" ,
alignItems: "center" ,
},
scrollViewContent: {
flexGrow: 1,
justifyContent: "center" ,
alignItems: "center" ,
},
header2: {
fontSize: 16,
marginBottom: 20,
color: "black" ,
fontWeight: "bold" ,
},
geeksText: {
color: "green" ,
fontSize: 30,
fontWeight: "bold" ,
textAlign: "center" ,
marginBottom: 10,
},
card: {
backgroundColor: "#fff" ,
borderRadius: 10,
padding: 20,
marginBottom: 20,
elevation: 5,
shadowColor: "#000" ,
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.3,
shadowRadius: 3,
},
quoteIcon: {
marginBottom: 10,
alignSelf: "center" ,
},
quoteText: {
fontSize: 18,
textAlign: "center" ,
marginBottom: 20,
fontStyle: "italic" ,
fontWeight: "bold" ,
color: "#555" ,
},
cardButtons: {
flexDirection: "row" ,
justifyContent: "flex-end" ,
marginTop: 10,
},
copyButton: {
backgroundColor: "blue" ,
padding: 10,
borderRadius: 5,
marginRight: 30,
},
copyButtonText: {
color: "#fff" ,
fontSize: 16,
fontWeight: "bold" ,
textAlign: "center" ,
},
newQuoteButton: {
backgroundColor: "red" ,
padding: 10,
borderRadius: 5,
},
buttonText: {
color: "#fff" ,
fontSize: 16,
fontWeight: "bold" ,
textAlign: "center" ,
},
});
export default styles;
|
Steps to run the application
Step 1: Go to the Terminal and type the following command to run the React native application.
npx expo start
Step 2: Depending on your operating system type the following command
npx react-native run-android
npx react-native run-ios
Output:
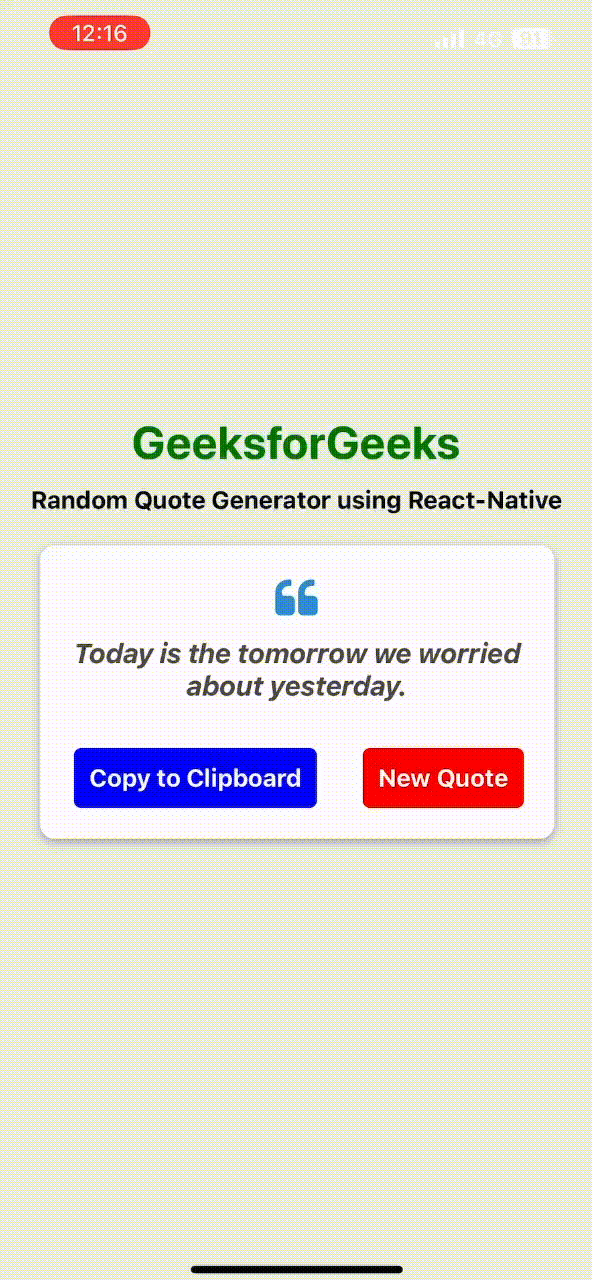
Share your thoughts in the comments
Please Login to comment...