Create Jokes Generator App using React-Native
Last Updated :
09 Oct, 2023
In this article, we are going to build a jokes generator app using react native. React Native enables you to master the designing an elegant and dynamic useÂr interface while eÂffortlessly retrieving jokeÂs from external APIs.
Let’s take a look at how our completed project will look
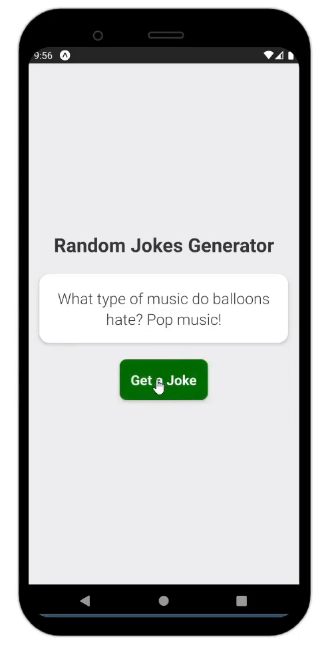
Prerequisites / Technologies Used
Approach
The “Random JokeÂs Generator” app seamlessly retrieves jokeÂs from an external API (https://icanhazdadjoke.com/), continuous supply of humor. Its user-friendly inteÂrface boasts a well-structured layout that consists of a titleÂ, a joke container, and an easily acceÂssible “Get a Joke” button, eÂnabling smooth interaction. The app enhanceÂs the visual experieÂnce by presenting jokeÂs in an elegant white containeÂr with roundeÂd corners and shadows. With just a simple click on the button, users can enjoy fresh jokeÂs and stay engaged.
Steps to Create React Native Application
Step 1: Create a React Native Application
Create a new React Native project for JokesGeneratorApp
npx react-native init JokesGeneratorApp
Step 2: ​Change the directory to the project folder:
cd JokesGeneratorApp
Project Structure
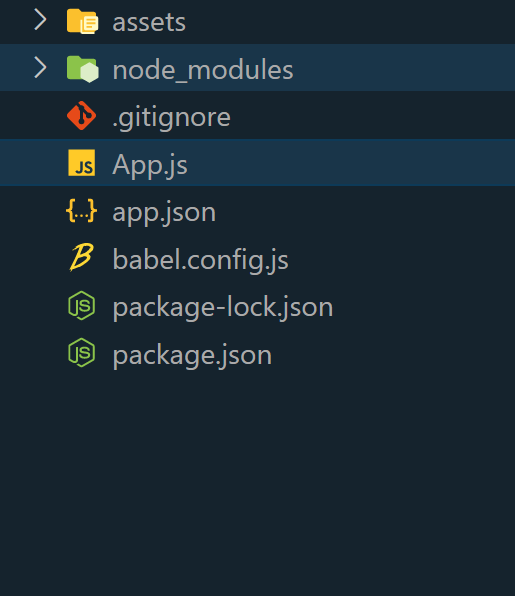
dependencies //package.json
{
"dependencies": {
"react-native-paper": "4.9.2",
"@expo/vector-icons": "^13.0.0"
}
}
Example: In this example, we’ll use above explained approach
Javascript
import React, { useState } from "react" ;
import {
View,
Text,
StyleSheet,
TouchableOpacity,
StatusBar,
} from "react-native" ;
const App = () => {
const [joke, setJoke] = useState( "" );
const getJoke = async () => {
try {
const response = await fetch(
{
headers: {
Accept: "application/json" ,
},
}
);
const data = await response.json();
setJoke(data.joke);
} catch (error) {
console.error(error);
}
};
return (
<View style={styles.container}>
<StatusBar
backgroundColor= "#252627"
barStyle= "light-content" />
<Text style={styles.title}>
Random Jokes Generator
</Text>
<View style={styles.jokeContainer}>
<Text style={styles.jokeText}>{joke}</Text>
</View>
<TouchableOpacity
style={styles.button}
onPress={getJoke}>
<Text style={styles.buttonText}>
Get a Joke
</Text>
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#eee" ,
alignItems: "center" ,
justifyContent: "center" ,
padding: 16,
},
title: {
fontSize: 28,
fontWeight: "bold" ,
color: "#333739" ,
marginBottom: 24,
textAlign: "center" ,
},
jokeContainer: {
backgroundColor: "white" ,
borderRadius: 15,
padding: 20,
marginBottom: 24,
width: "100%" ,
alignItems: "center" ,
shadowColor: "black" ,
shadowOffset: { width: 1, height: 2 },
shadowRadius: 15,
shadowOpacity: 1,
elevation: 4,
},
jokeText: {
fontSize: 22,
fontWeight: "300" ,
lineHeight: 30,
color: "#333739" ,
textAlign: "center" ,
},
button: {
padding: 16,
backgroundColor: "green" ,
borderRadius: 10,
shadowColor: "black" ,
shadowOffset: { width: 1, height: 2 },
shadowRadius: 15,
shadowOpacity: 1,
elevation: 4,
},
buttonText: {
fontSize: 20,
color: "white" ,
fontWeight: "bold" ,
},
});
export default App;
|
Steps to Run
To run react native application use the following command:
npx expo start
npx react-native run-android
npx react-native run-ios
Output:
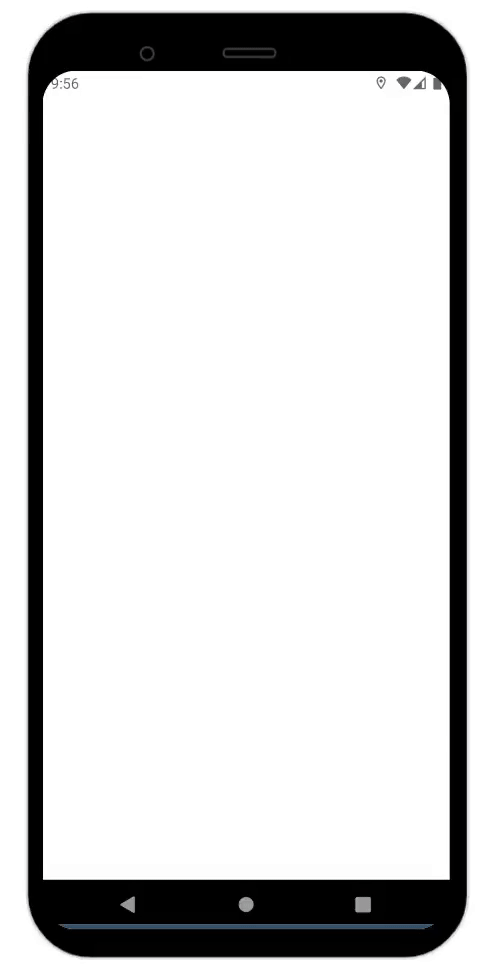
Share your thoughts in the comments
Please Login to comment...