How to Create Emoji Picker in React Native ?
Last Updated :
12 Sep, 2023
React Native is a popular cross-platform framework for mobile app development. Emojis have become common in modern applications, providing personalization and enhancing user engagement. In this article, we’ll see how we can add an emoji picker to a React Native application.
React Native doesn’t have an inbuilt emoji picker. So we are going to use an external emoji picker library called “react-native-emoji-selector“.
Prerequisites:
Installation:
Step 1: Set Up the Development Environment
Install Expo CLI globally by running this command:
npm install -g expo-cli​
Step 2: Create React Native Application With Expo CLI
Create a new native project with Expo CLI by using this command:
expo init emoji-picker-native
Step 3: ​Navigate to the project directory by using this command:
cd emoji-picker-native
Project Structure:
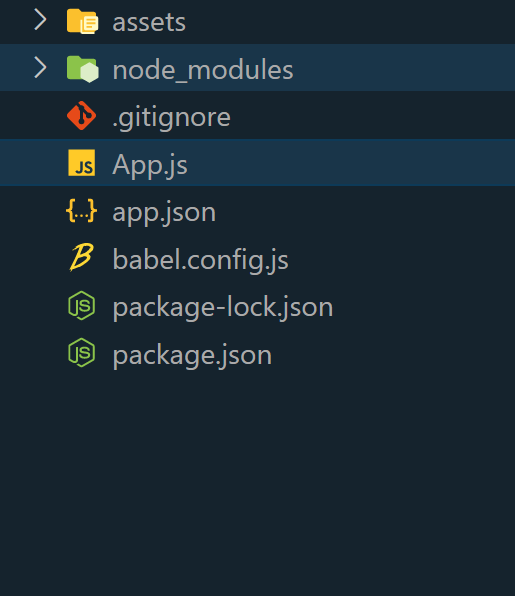
Project Structure
Step 4: Install Required Dependencies
Install the react-native-emoji-selector library in your project directory using this command:
npm install react-native-emoji-selector
Approach:
- Install “react-native-emoji-selector” library.
- Import necessary components and create an emoji picker component.
- Render the emoji picker and handle emoji selection.
- Add a button to open the emoji picker.
- Close the emoji picker after selecting an emoji and display or use the selected emoji in the app.
Example: In this example, the Emoji Picker App component. It uses the useState hook to manage showPicker and selectedEmoji state. The EmojiPicker component renders a button, displays the selected emoji, and conditionally shows the EmojiSelector component. The App component renders the EmojiPicker component with custom styles using StyleSheet.create.
Step 5: Open the App.js file. And paste the code into the App.js.
Javascript
import React, { useState } from "react" ;
import { View, TouchableOpacity, Text,
StyleSheet } from "react-native" ;
import EmojiSelector, { Categories }
from "react-native-emoji-selector" ;
const EmojiPicker = () => {
const [showPicker, setShowPicker] = useState( false );
const [selectedEmoji, setSelectedEmoji] = useState( "" );
const handleEmojiSelect = (emoji) => {
setSelectedEmoji(emoji);
setShowPicker( false );
};
return (
<View>
<TouchableOpacity
onPress={() => setShowPicker( true )}
style={styles.pickerButton}
>
<Text style={styles.buttonText}>
Open Emoji Picker
</Text>
</TouchableOpacity>
<View style={{ marginTop: 10 }}>
{selectedEmoji !== "" && (
<Text style={{ fontSize: 20 }}>
Selected Emoji: {selectedEmoji}
</Text>
)}
</View>
{showPicker && (
<EmojiSelector
onEmojiSelected={handleEmojiSelect}
category={Categories.all}
showTabs={ true }
showSearchBar={ true }
showHistory={ true }
columns={10}
placeholder= "Search emoji..."
/>
)}
</View>
);
};
const App = () => {
return (
<View style={styles.container}>
<EmojiPicker />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center" ,
alignItems: "center" ,
backgroundColor: "#f5f5f5" ,
},
pickerButton: {
backgroundColor: "#007bff" ,
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
},
buttonText: {
color: "#fff" ,
fontSize: 16,
fontWeight: "bold" ,
textAlign: "center" ,
},
});
export default App;
|
Step 6: To run the react native application, open the Terminal and enter the following command.
npx expo start
To run on Android:
npx react-native run-android
To run on Ios:
npx react-native run-ios
Output:
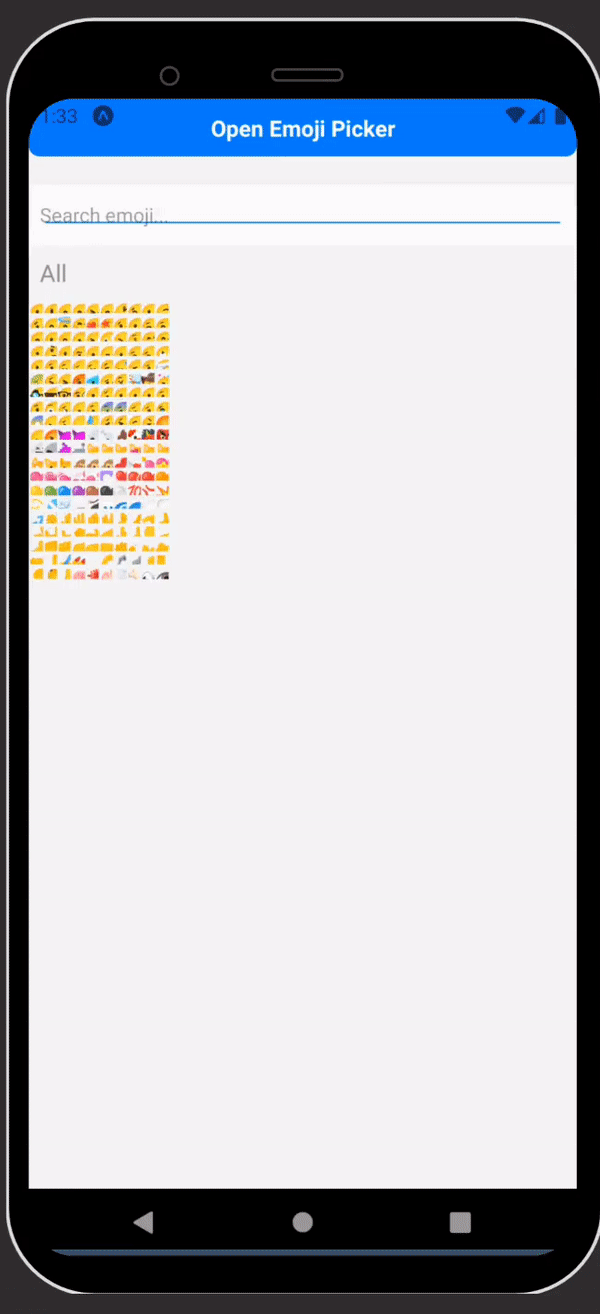
How To Add Emoji Picker in a React Native
Share your thoughts in the comments
Please Login to comment...