How to Create a Bookmark Link in HTML ?
Last Updated :
14 Mar, 2024
Bookmark Link is used for the users to navigate directly to the specific sections within the web application. We can create this bookmark link functionality in HTML using the id attribute with JavaScript and the class attribute with jQuery.
Below are the approaches to create a bookmark link in HTML:
Using HTML Only
In this approach, bookmark links are created using HTML only by assigning unique IDs to sections and corresponding anchor links. When users click on the links, browsers automatically scroll to the targeted sections.
Example: This example describes the implementation of a Bookmark Links using only HTML.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bookmark Link with only HTML</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="info">
<h1 style="color: green;">GeeksforGeeks</h1>
<h3>Using HTML only</h3>
<p>1.
<a href="#programming-section">
Go to Programming Section
</a>
</p>
<p>2.
<a href="#articles-section">
Explore Articles
</a>
</p>
<p>3.
<a href="#courses-section">
Browse Courses
</a>
</p>
</div>
<section id="programming-section">
<h2>Programming Section</h2>
<p>Welcome to the Programming section.
Here you can find tutorials and resources
related to various programming
languages and technologies.
</p>
</section>
<section id="articles-section">
<h2>Articles</h2>
<p>Explore a wide range of articles covering
topics such as algorithms, data structures,
software development, and more.
</p>
</section>
<section id="courses-section">
<h2>Courses</h2>
<p>Browse through our comprehensive collection
of courses designed to enhance your skills
in programming, web development, data science,
and other areas.
</p>
</section>
</body>
</html>
CSS
/* style.css*/
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
text-align: center;
}
.info{
display: flex;
flex-direction: column;
align-items: center;
padding: 20px;
}
section {
margin-top: 20px;
border: 2px solid yellow;
border-radius: 10px;
padding: 20px;
height: 200px;
background-color: #fff;
}
Output:
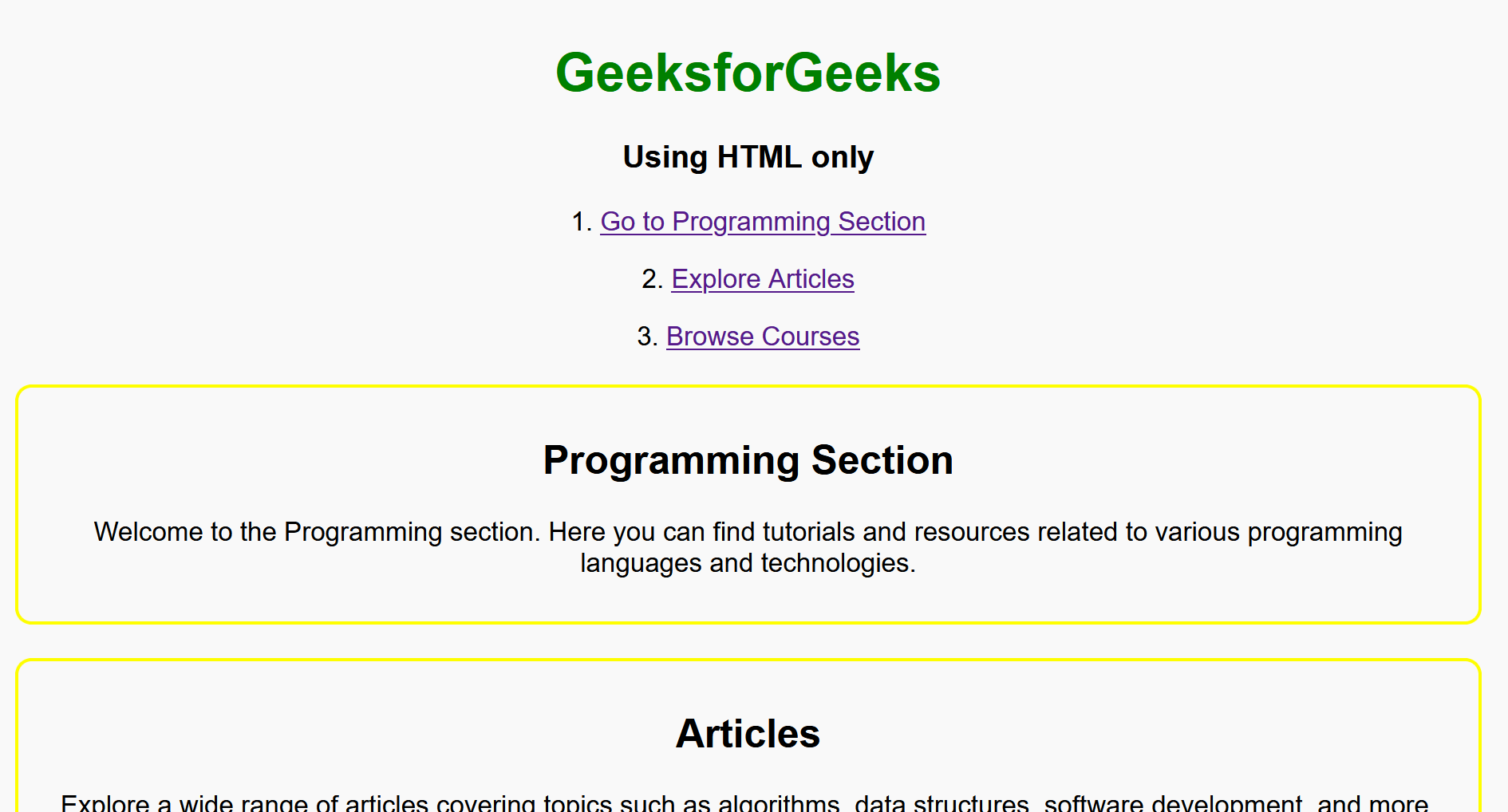
Using id Attribute with JavaScript
In this approach, we are using the id attribute in HTML to uniquely identify sections, and JavaScript with the onclick event to trigger a function (liveFn) that highlights the corresponding section for a 1-second time.
Syntax:
<section id="section_name"> Content </section>
Example: The example describes the implementation of a Bookmark Links using id Attribute with JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bookmark Link with JavaScript</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>GeeksforGeeks</h1>
<h2>Using id Attribute with JavaScript</h2>
<p>1. Programming:
<a href="#programming"
onclick="liveFn('programming')">
Go to Programming Section
</a>
</p>
<p>2. Articles:
<a href="#articles"
onclick="liveFn('articles')">
Explore Articles
</a>
</p>
<p>2. Courses:
<a href="#courses"
onclick="liveFn('courses')">
Browse Courses
</a>
</p>
<section id="programming">
<h2>Programming Section</h2>
<p>
Welcome to the Programming section of
GeeksforGeeks. Here you can find tutorials
and resources related to various
programming languages and technologies.
</p>
</section>
<section id="articles">
<h2>Articles</h2>
<p>
Explore a wide range of articles covering
topics such as algorithms, data structures,
software development, and more. Stay updated
with the latest trends and insights in the
tech industry.
</p>
</section>
<section id="courses">
<h2>Courses</h2>
<p>
Browse through our comprehensive collection
of courses designed to enhance your skills in
programming, web development, data science,
and other areas. Enroll in courses to advance
your knowledge.
</p>
</section>
<script>
function liveFn(sectionId) {
let section =
document.getElementById(sectionId);
section.classList.add('highlight');
setTimeout(function () {
section.classList.remove('highlight');
}, 2000);
}
</script>
</body>
</html>
CSS
/* style.css*/
body {
font-family: 'Arial', sans-serif;
margin: 0;
text-align: center;
padding: 0;
background-color: #f0f0f0;
}
h1 {
color: green;
text-align: center;
}
h2 {
color: #333;
}
p {
color: rgb(0, 0, 0);
font-weight: bold;
}
a {
color: #007bff;
text-decoration: none;
cursor: pointer;
}
a:hover {
color: #0056b3;
}
section {
background-color: #fff;
padding: 20px;
margin: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
transition: box-shadow 0.3s ease;
}
section:hover {
box-shadow: 0 0 20px rgba(0, 0, 0, 0.2);
}
.highlight {
background-color: yellow;
}
Output:
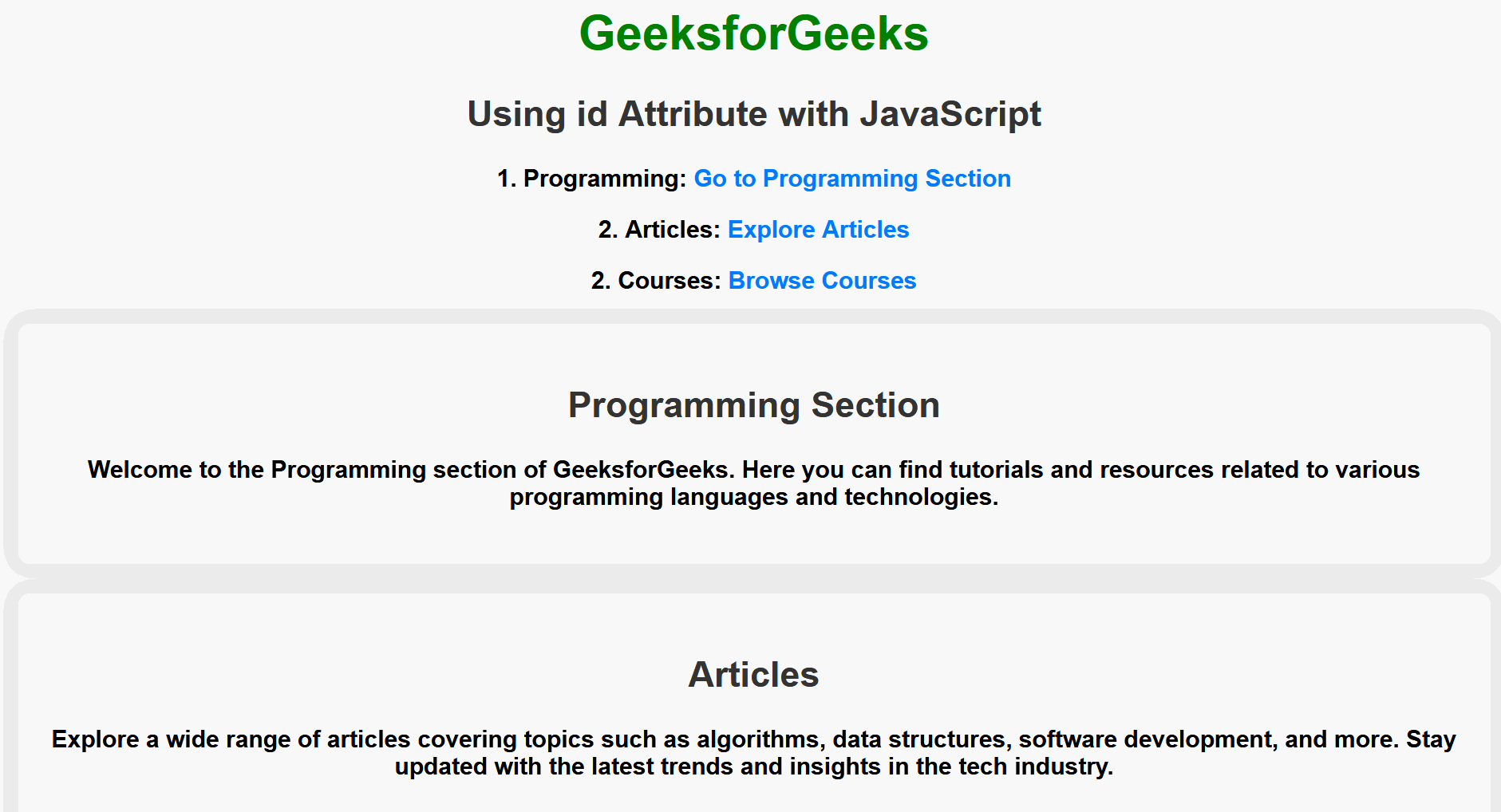
Using class Attribute with jQuery
In this approach, we are using the class attribute in HTML to categorize sections, and jQuery to handle the click event on links with the class bookmark-link. When the section is clicked the script scrolls the page to the targeted section and applies a highlighting effect.
Syntax:
<section class="section_name">Content</section>
Example: The example describes the implementation of a Bookmark Links using class Attribute with jQuery.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bookmark Link with jQuery</title>
<link rel="stylesheet" href="style.css">
<script
src="https://code.jquery.com/jquery-3.6.4.min.js">
</script>
</head>
<body>
<header>
<h1>GeeksforGeeks</h1>
<h3>Using class Attribute with jQuery</h3>
</header>
<main>
<p>1. Programming:
<a href="#" class="bookmark-link"
data-target=".programming-section">
Go to Programming Section
</a>
</p>
<p>2. Articles:
<a href="#" class="bookmark-link"
data-target=".articles-section">
Explore Articles
</a>
</p>
<p>3. Courses:
<a href="#" class="bookmark-link"
data-target=".courses-section">
Browse Courses
</a>
</p>
<section class="programming-section">
<h2>Programming Section</h2>
<p>
Welcome to the Programming section of
GeeksforGeeks. Here you can find tutorials
and resources related to various programming
languages and technologies.
</p>
</section>
<section class="articles-section">
<h2>Articles</h2>
<p>
Explore a wide range of articles covering
topics such as algorithms, data structures,
software development, and more. Stay updated
with the latest trends and insights in the
tech industry.
</p>
</section>
<section class="courses-section">
<h2>Courses</h2>
<p>
Browse through our comprehensive collection
of courses designed to enhance your skills in
programming, web development, data science,
and other areas. Enroll in courses to advance
your knowledge.
</p>
</section>
</main>
<script>
$(document).ready(function () {
$('.bookmark-link').on('click', function () {
var targetClass = $(this).data('target');
var section = $(targetClass);
$('html, body').animate({
scrollTop: section.offset().top
}, 1000);
section.addClass('highlight');
setTimeout(function () {
section.removeClass('highlight');
}, 2000);
});
});
</script>
</body>
</html>
CSS
/* style.css*/
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
box-sizing: border-box;
}
header {
text-align: center;
padding: 10px;
}
main {
text-align: center;
max-width: 800px;
margin: 20px auto;
background-color: white;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
padding: 20px;
border-radius: 10px;
}
h1 {
color: green;
}
h2 {
color: #333;
}
p {
line-height: 1.5;
}
.bookmark-link {
color: blue;
text-decoration: none;
cursor: pointer;
}
.bookmark-link:hover {
text-decoration: underline;
}
section {
margin-top: 20px;
border: 2px solid yellow;
border-radius: 10px;
padding: 10px;
background-color: #fff;
}
.highlight {
background-color: rgb(255, 0, 0);
transition: background-color 1s ease;
}
Output:
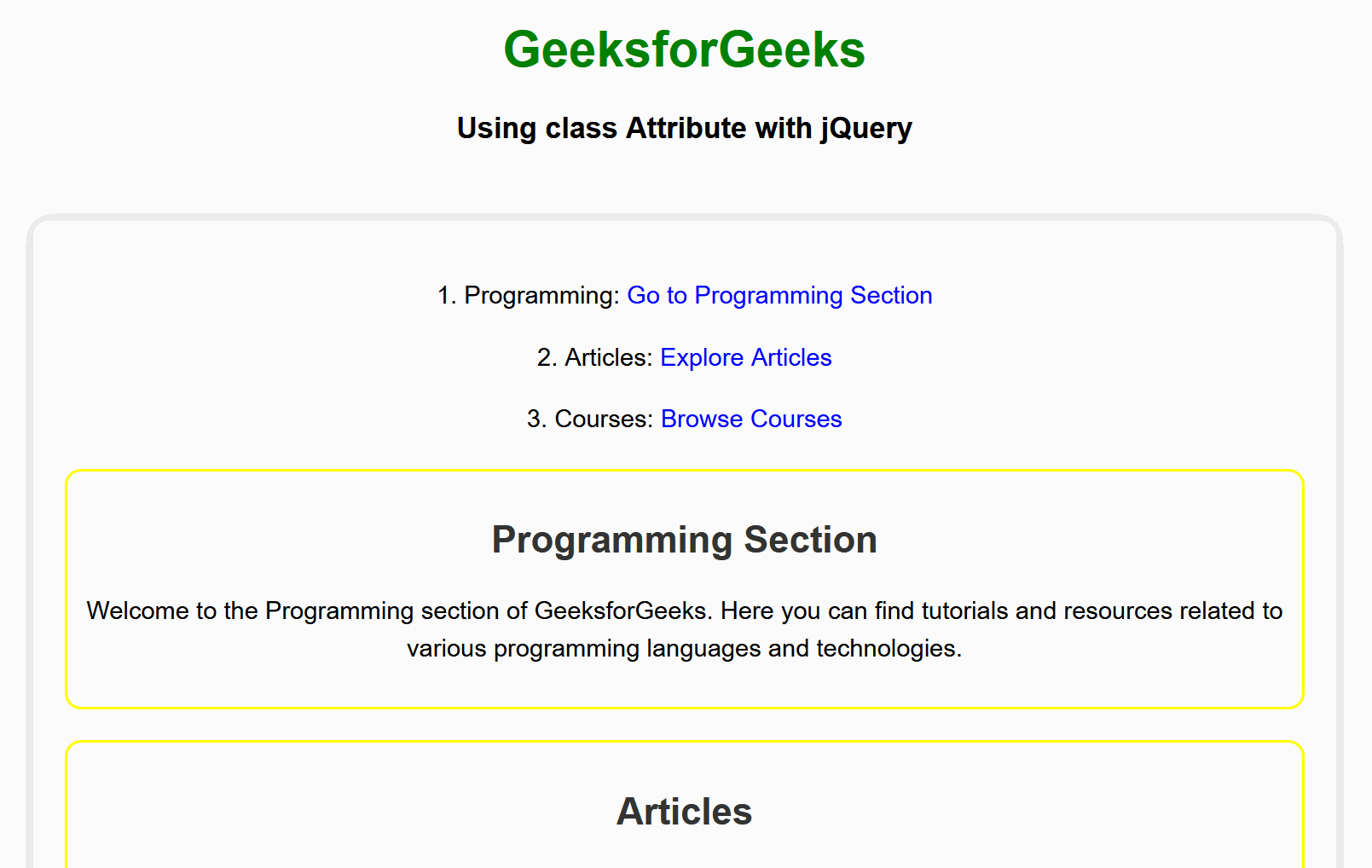
Share your thoughts in the comments
Please Login to comment...