How to Change input type=”date” Format in HTML ?
Last Updated :
11 Jan, 2024
The <input> element with type=”date” is used to allow the user to choose a date. By default, the date format displayed in the input field is determined by the user’s browser and operating system settings. However, it is possible to change the format of the date displayed in the input field using HTML.
- JavaScript can be employed to enforce a specific format as browsers may not directly support changing the format.
- Include a
placeholder
attribute with the desired date format, like “dd-mm-yyyy.”
Syntax:
<input type="date" name="date" id="date">
Approaches: There are a few approaches to changing the format of the date displayed in the input field using HTML only.
Using the “pattern” attribute:
The “pattern” attribute allows you to specify a regular expression that the date input value must match. By using the “pattern” attribute, you can specify a custom date format that the user must enter. However, this approach does not change the format of the date displayed in the input field.
Syntax:
<label for="date">Enter a date (YYYY-MM-DD):</label>
<input type="date" name="date" id="date" pattern="\d{4}-\d{2}-\d{2}">
Example: Implementation of the “pattern” attribute.
HTML
<!DOCTYPE html>
< html >
< body >
< h1 style = "text-align: center; color: green;" >
GeeksforGeeks
</ h1 >
< form style = "text-align: center;" >
< label for = "name"
style = "display: block; margin-bottom: 10px;" >
Name:
</ label >
< input type = "text" name = "name" id = "name" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "class"
style = "display: block; margin-bottom: 10px;" >
Class:
</ label >
< input type = "text" name = "class" id = "class" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "date" style = "display: block; margin-bottom: 10px;" >
Enter a date (YYYY-MM-DD):
</ label >
< input type = "text" name = "date" id = "date"
pattern = "\d{4}-\d{2}-\d{2}" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< input type = "submit" value = "Submit"
style="background-color: green; color: #fff;
padding: 10px 20px; border-radius: 5px;
border: none; cursor: pointer;">
</ form >
</ body >
</ html >
|
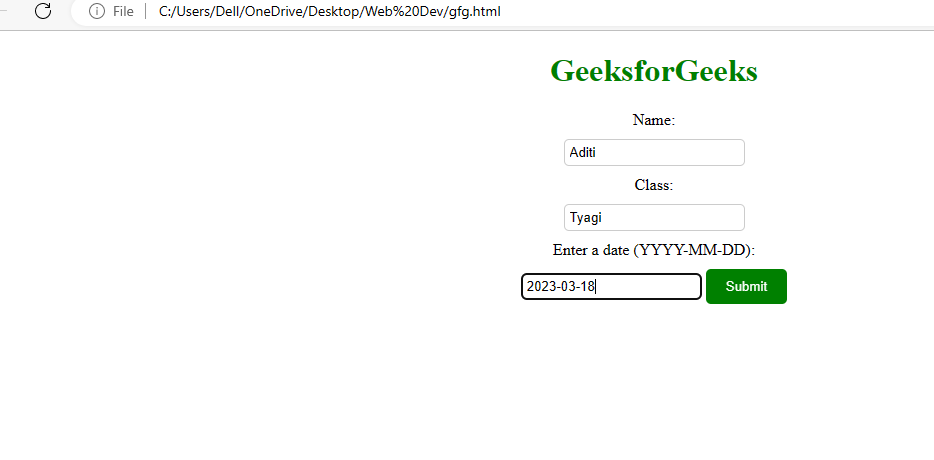
Using pattern attribute
Using the “placeholder” attribute:
The “placeholder” attribute allows you to specify a hint that describes the expected value of the input field. By using the “placeholder” attribute, you can specify a custom date format that the user can use as a guide when entering the date.
Syntax:
<label for="date">Enter a date (YYYY-MM-DD):</label><input type="date" name="date" id="date" placeholder="YYYY-MM-DD">
Example: Implementation of the “placeholder” attribute
HTML
<!DOCTYPE html>
< html >
< body >
< h1 style = "text-align: center; color: green;" >
GeeksforGeeks
</ h1 >
< form style = "text-align: center;" >
< label for = "name"
style = "display: block; margin-bottom: 10px;" >
Name:
</ label >
< input type = "text" name = "name" id = "name" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "class"
style = "display: block; margin-bottom: 10px;" >
Class:
</ label >
< input type = "text" name = "class" id = "class" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "date"
style = "display: block; margin-bottom: 10px;" >
Enter a date (YYYY-MM-DD):
</ label >
< input type = "date" name = "date" id = "date"
placeholder = "YYYY-MM-DD" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< input type = "submit" value = "Submit"
style="background-color: green; color: #fff;
padding: 10px 20px; border-radius: 5px;
border: none; cursor: pointer;">
</ form >
</ body >
</ html >
|
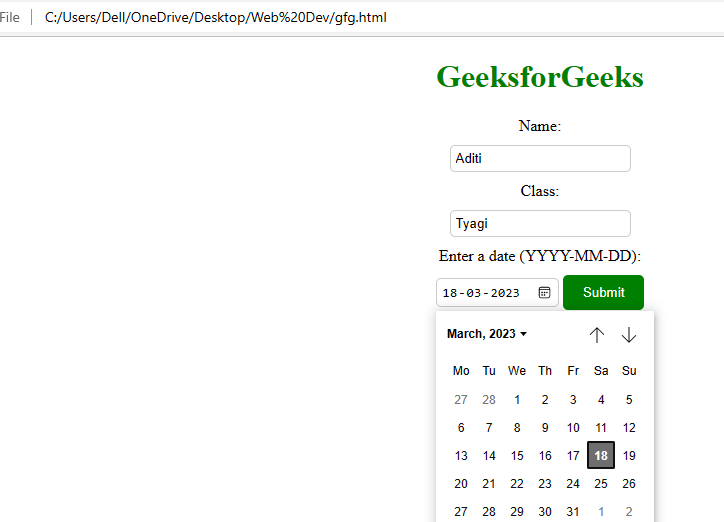
Using placeholder attribute
Using the “value” attribute:
The “value” attribute allows you to set the default value of the input field. By using the “value” attribute, you can set a custom date format that will be displayed in the input field by default. However, this approach does not allow the user to change the date format.
Syntax:
<label for="date">Enter a date (YYYY-MM-DD):</label>
<input type="date" name="date" id="date" value="2023-03-21">
Example : Implementation of the “value” attribute:
HTML
<!DOCTYPE html>
< html >
< body >
< h1 style = "text-align: center; color: green;" >
GeeksforGeeks
</ h1 >
< form style = "text-align: center;" >
< label for = "name"
style = "display: block; margin-bottom: 10px;" >
Name:
</ label >
< input type = "text" name = "name" id = "name" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "class"
style = "display: block; margin-bottom: 10px;" >
Class:
</ label >
< input type = "text" name = "class" id = "class" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< label for = "date"
style = "display: block; margin-bottom: 10px;" >
Enter a date (YYYY-MM-DD):
</ label >
< input type = "date" name = "date" id = "date"
value = "2022-01-01" required
style="padding: 5px; border-radius: 5px;
border: 1px solid #ccc; margin-bottom: 10px;">
< input type = "submit" value = "Submit"
style="background-color: green; color: #fff;
padding: 10px 20px; border-radius: 5px;
border: none; cursor: pointer;">
</ form >
</ body >
</ html >
|
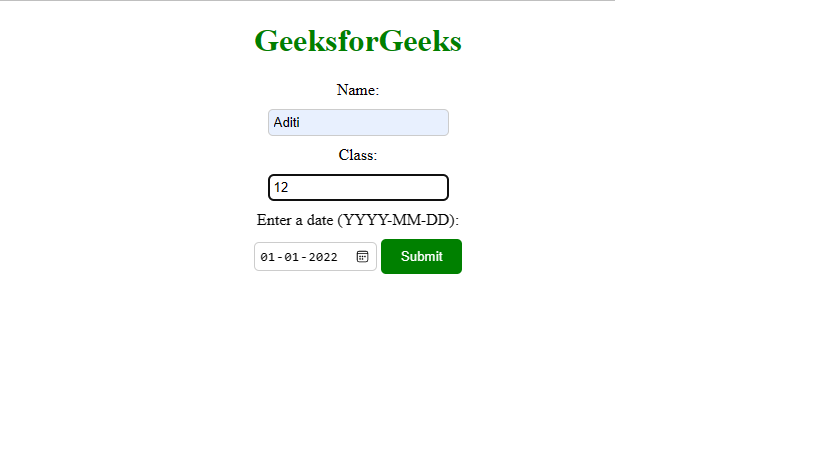
By default, date has been set using the value attribute
To use the “value” attribute you need to change the input type for the date field from “text” to “date”. In the above code, the “value” attribute of the date input field is set to “2022-01-01”, which pre-fills the input field with this date.
Share your thoughts in the comments
Please Login to comment...