How to Connect Front End and Backend
Last Updated :
02 Jan, 2024
“Connecting front-end to back-end is like building a bridge between worlds. How can you construct a pathway that seamlessly connects user interface with server functionality?”
Let’s dive into this in detail……
Every successful web application is built on the synergy between how frontend interacts with backend. Whether you’re building a dynamic website or a robust web application, the seamless connection between these two realms is predominant. In this guide, we’ll unravel the mysteries behind connecting the front end with back-end, shedding light on the process in a friendly format. But before directly jumping into that, let’s first discuss what these technologies actually are.
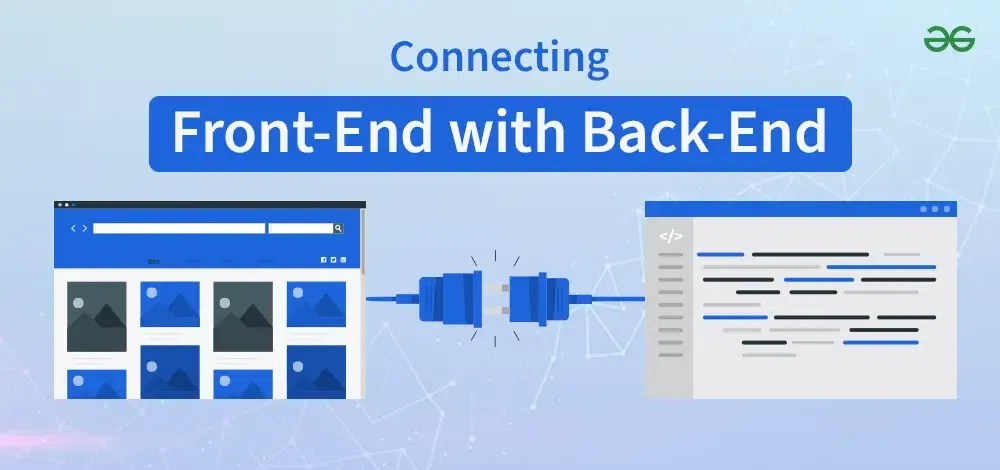
What is Frontend?
Front-end development, also known as client-side is the art of making websites look good and work well for the people who use them. It is like painting a picture on a canvas, but the canvas is your web browser and the paint is code. A beautiful and useful website may be made essentially by knowing how to use different colors, forms, and designs.
Languages like HTML, CSS, and JavaScript are used in front-end development to construct visually appealing and logically functional websites. The elements and structure of a website are defined by HTML, styled by CSS to produce an eye-catching visual, and integrated with JavaScript to add interaction and general functionality. Additionally, some frameworks like React, Vue, or Angular make development easier and faster by providing specific environment components.
What is Backend?
The back-end development, also known as server side is the process of making websites work and perform well for the users and other applications. It is like building the engine and the wiring of a car, but the car is your website and the engine and the wiring are code. To develop and maintain the server-side (backend) applications for your website, you must be very proficient in using a variety of languages, tools, and frameworks.
In backend development use languages like Python, Java, Ruby, PHP, and JavaScript to build and manage server-side software. This phase manages requests from the frontend, interacts with databases and APIs, and then ensures data storage, security, and performance. Various Tools like PostgreSQL, MongoDB, Express.js, Django, Laravel, and Flask simplify and enhance backend development.
If you want to learn about the differences between these two in-depth, refer Frontend vs Backend
Now let’s discuss our main topic-How to connect backend with front end?
Communication Methods
1. RESTful APIs:
REST (Representational State Transfer) is an architectural style for creating web services. Â This is the most popular approach. It generally uses HTTP request and response methods in order to exchange data in a normalize format. The backend exposes different endpoints for multiple functionalities, and then frontend makes calls to these endpoints in order to retrieve or manipulate data.
Procedure:
- Client (Frontend):
- Makes an HTTP request to a specific API endpoint (URL) on the server.
- Specifies the request method (GET, POST, PUT, DELETE) and the desired action.
- May include request body with data for specific actions like creation or update.
- Server (Backend):
- Receives the request and identifies the targeted endpoint based on the URL and method.
- Processes the request, accessing databases, performing calculations, or interacting with other services.
- Prepares a response containing the requested data, status code (e.g., 200 for success), and any additional information.
- Client:
- Receives the response and interprets the status code and data content.
- Updates the user interface or performs further actions based on the returned information.
Example Source Code:
Frontend (JavaScript):
Javascript
fetch( '/products/123' , {
method: 'GET' ,
})
.then(response => response.json())
.then(data => {
});
|
Backend (Node.js):
Node
app.get(‘/products/:id’, (req, res) => {
const productId = req.params.id;
// Fetch product data from database
db.getProduct(productId).then(product => {
res.json(product); // Send product data as JSON response
}).catch(error => {
res.status(500).send(error.message); // Handle error
});
});
2. WebSockets:
A persistent, bi-directional communication protocol that connects a client and a server is called WebSockets. WebSockets, in contrast to conventional HTTP, allow for continuous communication, which makes them appropriate for applications that need real-time updates.
Procedure:
- Client:
- Establishes a WebSocket connection with the server using a specific URL.
- Sends messages to the server containing data or requests.
- Server:
- Receives messages from the client and processes them.
- May send messages back to the client with updates or responses.
- Can maintain persistent connections with multiple clients simultaneously.
- Client:
- Receives messages from the server and updates the user interface accordingly.
- Can react to server updates in real-time, enhancing user experience.
Example Source Code:
Frontend (JavaScript):
Javascript
ws.onmessage = (event) => {
const message = JSON.parse(event.data);
};
ws.send( 'Hello from the client!' );
|
Backend (Node.js):
Node
const wsServer = new WebSocket.Server({ port: 3000 });
wsServer.on(‘connection’, (socket) => {
// Event listener for handling incoming messages from a client
socket.onmessage = (event) => {
// Parsing the JSON message received from the client
const message = JSON.parse(event.data);
// Process the message (e.g., handle business logic)
// Sending a response back to the client
socket.send(‘Server response’);
};
});
3. Server-Side Rendering (SSR):
In Server-Side Rendering, the server crafts the webpage’s HTML and sends it to the browser, sparing the client’s browser from this hefty task. The initial page loads much more quickly with this technique, which also improves search engine optimisation (SEO) and makes it easier for search engines to understand the content.
Procedure:
- Client:
- Sends a request to the server for a specific page.
- Server:
- Generates the complete HTML page with the requested content using server-side scripting languages.
- Embeds any necessary JavaScript code within the HTML.
- Client:
- Receives the entire HTML page and displays it directly.
- Once loaded, the embedded JavaScript code takes over for dynamic interactions.
Example Source Code:
Backend (Python):
Python
from flask import Flask, render_template
app = Flask(__name__)
@app .route( '/' )
def index():
data = get_data_from_database()
return render_template( 'index.html' , data = data)
def get_data_from_database():
return { 'message' : 'Hello, data from the database!' }
if __name__ = = '__main__' :
app.run(debug = True )
|
Frontend (HTML):
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Flask App</ title >
</ head >
< body >
< h1 >Data from the Database</ h1 >
< p >{{ data }}</ p >
</ body >
</ html >
|
4. GraphQL
Client interactions with backend services are revolutionised by Facebook’s GraphQL query language for APIs. It gives developers a more effective, adaptable, and developer-friendly method of retrieving data by addressing many of the issues that our conventional RESTful APIs pose.
Procedure:
- Client:
- Defines a GraphQL query specifying the desired data structure and fields.
- Sends the query to the GraphQL server.
- Server:
- Receives the query and parses it to understand the requested data.
- Fetches data from various sources (databases, APIs, etc.) based on the query.
- Combines the data into a single response matching the requested structure.
- Client:
- Receives the response and easily extracts the specific data needed.
- Updates the user interface based on the retrieved information.
Example Source Code:
Frontend (JavaScript):
Javascript
const query = `
query {
user {
id
name
posts {
id
title
content
}
}
}
`;
fetch( '/graphql' , {
method: 'POST' ,
headers: {
'Content-Type' : 'application/json' ,
},
body: JSON.stringify({ query }),
})
.then(response => response.json())
.then(data => {
});
|
Backend (Node.js):
Node
const { ApolloServer, gql } = require(‘apollo-server’);
// Define GraphQL schema using the gql tag
const typeDefs = gql`
type User {
id: ID!
name: String!
posts: [Post!]!
}
type Post {
id: ID!
title: String!
content: String!
}
type Query {
user: User
}
`;
// Define resolvers to handle GraphQL queries
const resolvers = {
Query: {
user: () => {
// Fetch user data and posts from the database (mock data for illustration)
return {
id: ‘123’,
name: ‘John Doe’,
posts: [{
id: ‘456’,
title: ‘My first post’,
content: ‘This is my first post!’,
}],
};
},
},
};
// Create an Apollo Server instance with the defined schema and resolvers
const server = new ApolloServer({ typeDefs, resolvers });
// Start the server and listen for incoming GraphQL requests
server.listen().then(({ url }) => {
console.log(`GraphQL server running on ${url}`);
});
Conclusion
Connecting the frontend and backend is like establishing a secure communication channel between two distinct domains: the user interface and server functionality. By grasping the unique roles of each side and adopting suitable communication methods, you pave the way for efficient data exchange and enhanced user interaction.
Whether opting for RESTful APIs, leveraging WebSockets, implementing Server-Side Rendering, or embracing GraphQL, the crux lies in selecting the strategy aligned with your project’s specific needs. With careful planning and well-written code, you can create a strong communication channel that will provide your users with a smooth and effective experience.
Explore these technical concepts thoroughly, perfect your strategy, and create solutions that will enhance your web applications to a whole new level!
FAQs
1. What is the significance of connecting the backend with the frontend in web development?
Users can interact with the system seamlessly when the frontend and backend are connected. The frontend offers the user interface(UI) that allows users to engage with these capabilities, while the backend manages data, business logic, and other essential functions. Web applications that are dynamic and interactive are made possible by their connection.
2. Can you explain RESTful APIs in simple terms?
RESTful APIs use the principles of Representational State Transfer (REST) to create a standard for communication. They define how resources (data entities) are identified and manipulated using standard HTTP methods (GET, POST, PUT, DELETE). Each resource has a unique URL, and interactions are generally stateless which means each request contains all the information needed to fulfill it.
3. What are the main ways the frontend and backend communicate in web development?
Different forms of communication meet different purposes. RESTful APIs provide a standard way for the frontend to request and receive data. WebSockets allow real-time, bidirectional communication for live updates. Server-Side Rendering (SSR) enhances initial page load performance. The frontend can request specific data in a streamlined and effective manner with GraphQL.
4. How WebSocket communication is different from traditional RESTful API communication?
WebSockets generally provide a continuous, two directional communication channel, making them suitable for real-time updates. Traditional RESTful APIs follow stateless request-response interactions, where each request is independent and contains all the necessary information.
Share your thoughts in the comments
Please Login to comment...