How to connect the components using Connect() in React Redux
Last Updated :
04 Apr, 2024
In React Redux, you use the connect()
function to connect your components to the Redux store. This function takes two parameters: mapStateToProps
and mapDispatchToProps
. mapStateToProps
maps the store’s state to the component’s props, while mapDispatchToProps
maps dispatch actions to props. By using connect( )
, your components can access the store’s state and dispatch actions easily.
Output Preview: Let us have a look at how the final output will look like.
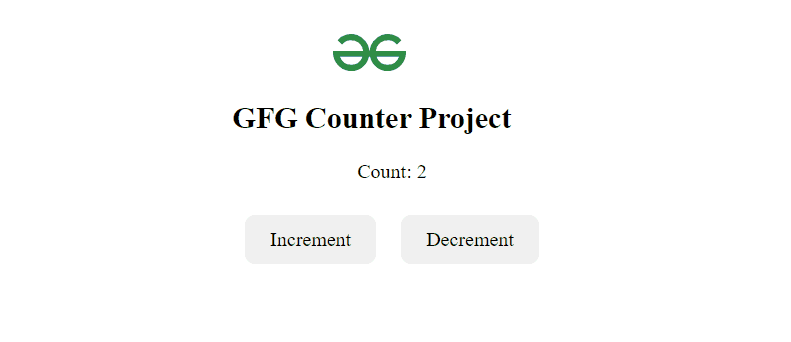
Prerequisites
Approach
- Initialize a new React project (Counter) using Create React App. Install necessary dependencies such as redux, react-redux, and any additional packages required for your project.
- Create a directory structure for Redux-related files, define your actions , reducers, creating store etc.
- Design the component hierarchy based on your application’s requirements. Create React components for UI elements, organizing them into appropriate folders (e.g., src/components). Ensure each component is designed to be reusable and focused on a single responsibility.
- Connecting Components to Redux: This is the most crucial part of state management. Here importing react-components, Defining mapStateToProps function, mapDispatchToProps function such work is done. Use connect() to connect components to the Redux store, passing in mapStateToProps and mapDispatchToProps as arguments is also done here.
Steps to create application
Step 1: Create a reactJS application by using this command
npx create-react-app my-app
Step 2: Navigate to project directory
cd my-app
Step 3: Install the necessary packages/libraries in your project using the following commands.
npm install react react-dom redux react-redux
Project Structure:
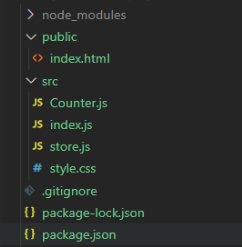
Counter Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2",
"redux": "^4.1.2",
"react-redux": "^7.2.6"
}
Example: Implementation to showcase how to connect the components using connect method in react redux.
CSS
/* style.css */
body {
margin: 0;
display: grid;
place-items: center;
height: 100vh;
}
img {
margin-left: 80px;
}
button {
border-radius: 8px;
border: 1px solid transparent;
padding: 0.6em 1.2em;
font-size: 1em;
font-weight: 500;
font-family: inherit;
cursor: pointer;
margin: 10px;
transition: border-color 0.25s;
}
p {
text-align: center;
}
button:hover {
border-color: #646cff;
}
JavaScript
// Counter.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './store';
const mapStateToProps = (state) => ({
count: state.counter,
});
const mapDispatchToProps = {
increment,
decrement,
};
const ConnectedCounter = ({ count, increment, decrement }) => (
<div>
<p id='count'>Count: {count}</p>
<button onClick={increment}>
Increment
</button>
<button onClick={decrement}>
Decrement
</button>
</div>
);
export default connect(mapStateToProps, mapDispatchToProps)(ConnectedCounter);
JavaScript
// store.js
import { createStore } from 'redux';
// actions
export const increment = () => ({
type: 'INCREMENT',
});
export const decrement = () => ({
type: 'DECREMENT',
});
// counterReducer
const initialState = {
counter: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, counter: state.counter + 1 };
case 'DECREMENT':
return { ...state, counter: state.counter - 1 };
default:
return state;
}
};
// store
const store = createStore(counterReducer);
export default store;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import { Provider } from 'react-redux'
import './style.css'
import store from './store';
import Counter from './Counter';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<div id='wrapper' >
<div id='header' >
<img src='https://media.geeksforgeeks.org/gfg-gg-logo.svg'
alt='gfg_logo' />
<h2 id='todo_banner'>
GFG Counter Project
</h2>
</div>
<Counter />
</div>
</Provider>
);
Output:
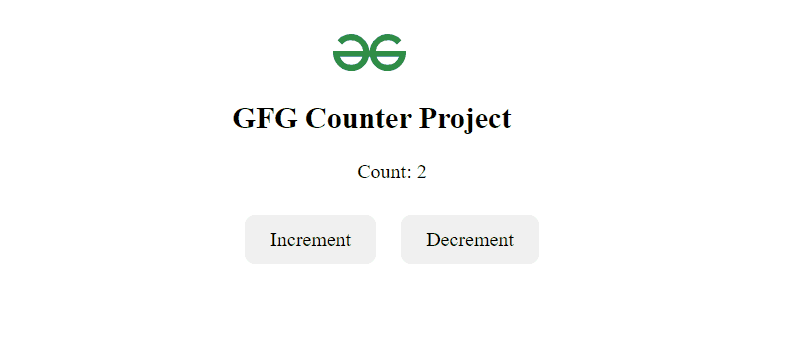
Share your thoughts in the comments
Please Login to comment...