Getting started with Scripting in the Postman
Last Updated :
27 Oct, 2023
Postman is a powerful tool for testing and automating APIs, and one of its most valuable features is the ability to write and execute scripts. With scripting in Postman, you can add dynamic behavior, automate repetitive tasks, and extract data from responses. In this article, we’ll go through the basics of scripting in Postman. Make sure you have Postman installed on your desktop. If not download it from the official website of Postman.
Why scripting in Postman?
Scripting in Postman opens up a world of possibilities for enhancing your API testing and automation workflow. Here are a few reasons why you might want to use scripting in Postman:
- Dynamic test data: You can generate dynamic test data as you execute scripts like people names, city names, phone numbers, pin codes, and so on. Thus, you don’t need to bother yourself with producing test data.
- Response validation: You can write scripts to validate the responses you receive, ensuring they meet your expected criteria. For example, Does the response have status code 200? Does the response body have a afterwardcorrected schema? This helps you catch unexpected changes and detect errors in the API.
- Automation: You can automate your testing workflows by writing scripts that execute a series of requests that are related to each other and validate responses automatically. For instance, you can simulate tasks like authentication and generating some data and then deleting it afterwardand using a series of GET, POST, PUT, DELETE requests. This is particularly helpful for creating complex testing workflows.
- Data Extraction: You can extract data from responses and store it as environment variables to use it in subsequent requests. This is useful for scenarios where you need to capture tokens, session IDs, or other dynamic values.
There are two types of scripts in Postman:
- Pre-request script: These scripts run before sending a request. These are used for doing some setup required before sending the request like setting some headers, cookies, generating request body etc.
- Test scripts: These scripts run after the response is received. They are used to validate the response body, check status codes, perform various assertions and so on.
Writing scripts in Postman
Writing test scripts in Postman is as simple as writing JavaScript since Postman scripts uses JavaScript language.
To write pre-request scripts go to pre-request scripts tab and to write test scripts go to Tests tab.
Variables in Postman test scripts
There are 4 types of variables in Postman:
- Environment variables: Environment variables enable you to scope your work to different environments, for example local development versus testing or production. One environment can be active at a time. If you have a single environment, using collection variables can be more efficient, but environments enable you to specify role-based access levels. Create and manage them in the “Environment” section of Postman. Access them using pm.environment.get(‘variableName’) in scripts.
- Collection variables: Collection variables are available throughout the requests in a collection and are independent of environments. Collection variables don’t change based on the selected environment. Collection variables are suitable if you’re using a single environment, for example for auth or URL details. Define them in the “Variables” section of your collection. Access them using pm.collectionVariables.get(‘variableName’) in scripts.
- Local variables: Local variables are temporary variables that are accessed in your request scripts. Local variable values are scoped to a single request or collection run, and are no longer available when the run is complete. Local variables are suitable if you need a value to override all other variable scopes but don’t want the value to persist once execution has ended. Set them directly in the script using pm.variables.set(‘variableName’, ‘value’). Access them using pm.variables.get(‘variableName’) within the same script.
- Global Variables: Global variables enable you to access data between collections, requests, test scripts, and environments. Global variables are available throughout a workspace. Since global variables have the broadest scope available in Postman, they’re well-suited for testing and prototyping. In later development phases, use more specific scopes. Define them in the “Global” section of your workspace. Access them using pm.globals.get(‘variableName’) in scripts.
Pre request scripts examples: To access the request properties in postman you can pm.request
Javascript
pm.request.headers.add({
key: 'Authorization' ,
value: 'Bearer <YourAccessTokenHere>'
});
pm.request.url.addQueryParams({ page: 1 });
let obj = {
fname: pm.variables.replaceIn( '{{$randomFirstName}}' ),
lname: pm.variables.replaceIn( '{{$randomLastName}}' ),
country: pm.variables.replaceIn( '{{$randomCountry}}' )
}
pm.request.body.raw = JSON.stringify(obj);
pm.environment.set( 'id' , 14234233);
pm.collectionVariables.set( 'uid' , 93782937);
pm.environment.get( 'id' );
pm.collectionVariables.get( 'uid' );
pm.sendRequest({
method: 'GET' ,
});
|
Test scripts examples
A test scripts can contain multiple pm.test() calls. Each call to pm.test() is for testing one parameter of the response.
Validate response status code
Javascript
pm.test( 'Status code is 200' , function () {
pm.response.to.have.status(200);
});
|
Check response body JSON has a certain field
Javascript
const responseBody = pm.response.json();
pm.test( 'Response contains the "username" property' , function () {
pm.expect(responseBody).to.have.property( 'username' );
});
|
Response body JSON property value validation
Javascript
const responseBody = pm.response.json();
pm.test( 'Username is correct' , function () {
pm.expect(responseBody.username).to.eql( 'expected_username' );
});
|
Response time validation
Javascript
pm.test( 'Response time is acceptable' , function () {
pm.expect(pm.response.responseTime).to.be.below(500);
});
|
Handling Authentication
Javascript
const accessToken = pm.response.json().access_token;
pm.environment.set( 'access_token' , accessToken);
pm.request.headers.add({
key: 'Authorization' ,
value: `Bearer ${accessToken}`
});
|
There are many snippets available in Postman test scripts. They can be found on the right side of the test script editor.
Postman has built in functionality of generating fake data. You can know more about it here.
A simple test script in Postman
We looked at some basics scripting examples in the above code samples. Let’s create a real test script for a GET request to implement our learnings. You can go ahead and try to write some tests by yourself!
Example: Method – GET
Javascript
pm.test( "Status code is 200" , function () {
pm.response.to.have.status(200);
});
const jsonData = pm.response.json();
pm.test( "Response contains userId" , function () {
pm.expect(jsonData.userId).to.exist;
});
pm.test( "Response contains id" , function () {
pm.expect(jsonData.id).to.exist;
});
pm.test( "Response contains title" , function () {
pm.expect(jsonData.title).to.exist;
});
pm.test( "Response contains body" , function () {
pm.expect(jsonData.body).to.exist;
});
|
Now hit the send button and look the tests passing successfully in the result bar. At last, our efforts paid off.
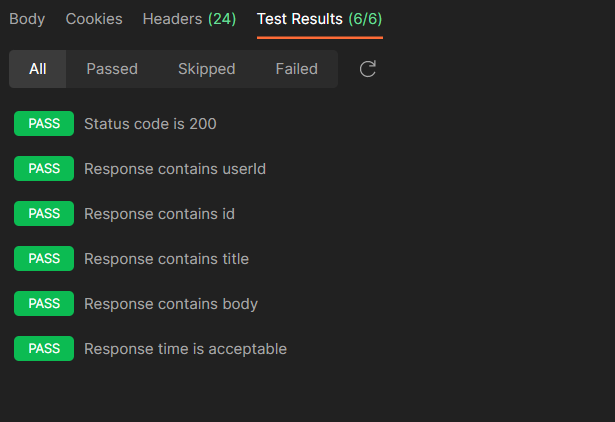
Share your thoughts in the comments
Please Login to comment...