A Web Application is software that executes on the web browser. It links the customers and the service providers as a medium of exchange. For example, Amazon provides a marketplace where sellers and buyers can coexist. It provides a mechanism to exchange services and perform operations seamlessly.
Understanding Web Clients and Servers
For any operation to be performed, a service provider and a service consumer need. The same relies on web applications. The one which provides the service is the web server and the one which requires it is the web client. The web client requests the client, in return for which the client responds. This is usually done through HTTP protocol and that’s the reason, why sometimes a web server is also known as an HTTP server.
The web server stores, processes, and provides the output to the client which further makes it user-friendly. A web browser is an example of a web client that requests with the required payload, and the server will respond, usually in JSON format.
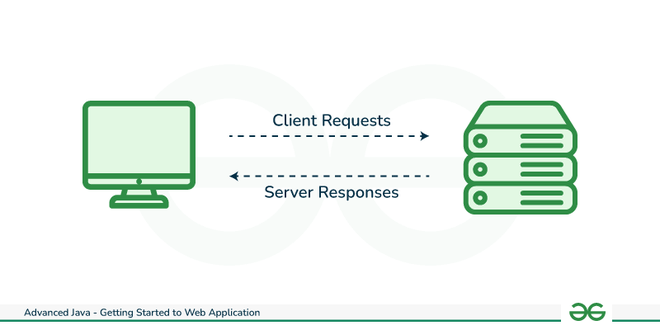
Client-Server Communication
Client-server communication is usually done through TCP/IP protocol. TCP covers part of transport and the sessions layer in a typical OSI model. TCP being a connection-oriented protocol makes sure that the connection remains in place till the messages are exchanges at the very end of the queue.
On the other hand, IP is a connectionless protocol which don’t communicate following the initial transmission of messages. TCP organizes the packets of information is a sequence which the IP treats as an independent unit. IP belongs to network layer in the OSI model.
HTTP Protocol Basics
Following are some of the methods in HTTP protocol.
HEAD
|
Gets the status of a resource.
|
GET
|
Retrieval of a resource.
|
POST
|
Creation of a new resource.
|
PUT
|
Update an already existing resource.
|
DELETE
|
Delete a resource.
|
HTML Language Basics
HTML stands for Hyper Text Markup Language which provides the structure of a web application. It consists of different elements each responsible for the different view of a component in the browser.
HTML
<!DOCTYPE html>
< html >
< head >
< title >GFG : Overview to HTML</ title >
</ head >
< body >
< h1 >Welcome To GFG</ h1 >
< p >This is an overview to HTML</ p >
</ body >
</ html >
|
Code Explanation:
- The <!DOCTYPE html> provides the reference that this is an HTML followed by its root element <html>.
- <head> provides the meta information of the HTML page which contains the links and <title> to the web page.
- <body> contains all the components and the modules of the web application. <h1> provides large headings while <p> denotes the paragraph.
- The boundary of the respective element is marked by its closing tag.
Introduction to Servlets
Servlets acts as the server-side heroes that processes the requests from the users’ web browser and sends back the response after the required manipulation is completed. Imagine it to be a communication channel between your server and users’ web browsers.
- Working of Servlets: Servlets catches the requests when the user interacts with the web application either let say, by clicking the button or submitting the form, process them using Java code, and then send a response back to the user’s browser.
- Servlet Lifecycle: Servlets lifecycle can be compared to a human one where they wake up during initialization, their active life will go on with handling requests, and say their goodbyes when the server is shutting down.
Popular Containers (In-Built Servlets)
Consider your web browser to be a house and the requests to be someone taking care of the tasks. So you can imagine the someone taking care of the tasks as the superhero which is nothing but a servlet container in the Java web world, such as Tomcat or Jetty.
They come pre-equipped with a bunch of built-in servlets which are somewhat ready-to-use, efficient, and optimized like smart butlers. You don’t have to create them; they’re already there to handle common tasks like serving static files, managing sessions, or handling error pages. It’s like assuming a team already present inside your house to complete the tasks as and when they receive.
Manual Servlets
For some additional tasks where maybe, you want a personalized caretaker who does things exactly the way you like, this is where in-built servlets lack and exactly that’s where manual servlets come in.
It is somewhat like hiring your own superhero. You write Java code for the servlet behavior, you decide the tasks, how it processes requests, and what responses it sends back, getting a full control over the things.
Example:
Imagine you want a servlet that welcomes users with a “Hello World!” message. You’d write a bit of Java code, compile it, set it up in your web application, and voila! Now, every time a user interacts with your servlet, it responds with a friendly greeting.
Java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class GFG extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
out.println( "<html><body>" );
out.println( "<h2>Hello World!</h2>" );
out.println( "</body></html>" );
}
}
|
Servlets are like those workers who works backstage of the web applications. Right from handling requests, they process information, and make sure everything runs smoothly behind the application making it more user friendly. Whether it’s saying, “Hello World!” or managing complex operations, servlets are there to make your web applications come to life.
Web Application Architecture
1. Monolithic Architecture
- Monolithic architecture is considering your web app as one big, self-contained unit where everything, from its behavior to its working, is packed together.
- Under this, the entire web application is developed and deployed as a single unit.
- All components, including user interface, business logic, and database access, are tightly integrated.
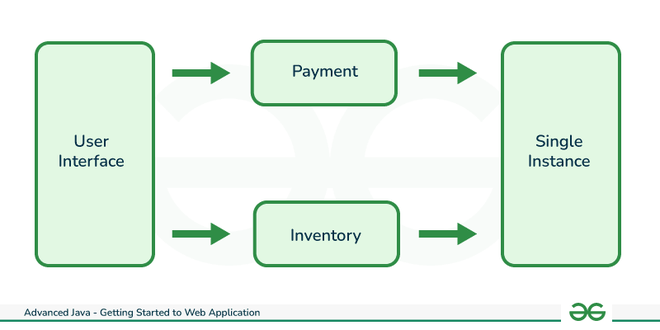
2. Client-Server Architecture
- Consider the process followed on a restaurant. The waiter takes the orders from customers and communicates with the chef in the kitchen, who will actually cook the food.
- Now relate waiter to the client, customers to be users and the chef to be the server. The client takes the requests from the users and processes it to the server where the actual processing is done.
- In the client-server model, the application is split into two main parts: the client which handles the user interface and the server which manages the data processing.
- Communication occurs over a network, often using HTTP.
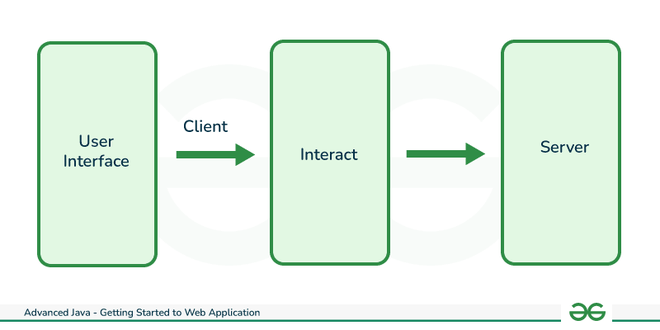
3. Microservices Architecture
- Consider a normal town where each shop (microservice) has a specialty in some work.
- This way, if one shop changes something, it doesn’t affect the others.
- Microservices break down the application into small, independent services, each focusing on a specific function.
- Services communicate through APIs.
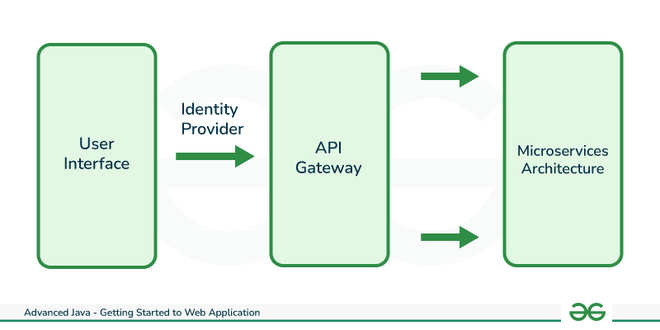
4. Serverless Architecture
- In a serverless setup, you don’t have to worry about maintaining the kitchen (server).
- It’s like ordering your food (function) which is prepared somewhere else, and you only pay for what you consume.
- Serverless architecture involves running code without managing the underlying infrastructure.
- Applications are built using small, stateless functions triggered by events.
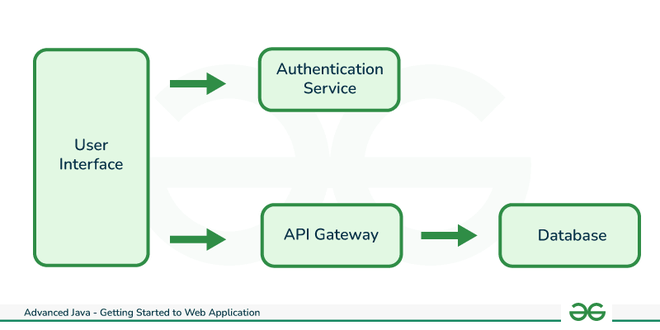
5. Progressive Web App (PWA) Architecture
- It can work even when there’s no internet, like a person who doesn’t need a specific headquarters.
- PWAs make sure your experience is seamless by using smart strategies (service workers), even if your internet connection isn’t.
- PWAs aim to provide a native app-like experience within a web browser.
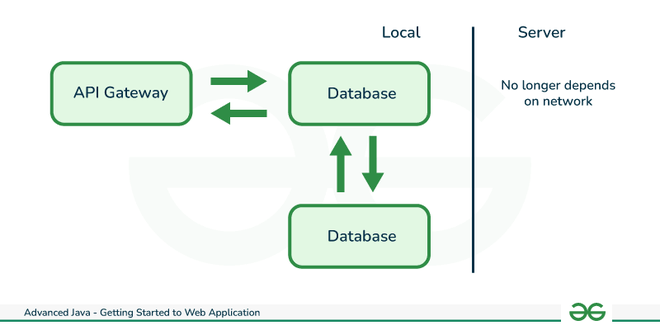
Java Web Frameworks
Java web frameworks acts like toolkits for building web applications. These frameworks provide you with a set of rules to make your job easier, you are being the master for the application. Two popular ones are Spring MVC and its close friend, Hibernate.
Spring MVC
Spring MVC helps you organize and manage your web application. It provides a structured way to build different parts of your app, like handling user requests, managing data, and showing results.
Spring MVC makes it easy to divide your code into smaller, more manageable pieces, so you’re not overwhelmed by a massive pile of building blocks.
Benefits of Spring MVC and Similar Frameworks
- Structure and Organization:
It organizes the code neatly somewhat like labelling the boxes which can be easier if you want to pick something.
- Reusability:
With Spring MVC, you can package features into modules and use it in other projects.
- Flexibility:
Spring MVC lets you choose the tools you like. It’s like what you want, I already have that with me. Just you need to use the same module.
- Security:
Web apps need to be safe and secure. Spring MVC comes with built-in security features which helps protect your users and their data, making your app a safe place to hang out.
- Integration:
Sometimes, you need to connect your app with other tools. Spring MVC makes this integration easy.
Handling HTTP requests and responses in Java servlets
1. Handling HTTP GET Request
In this example, the servlet responds to a GET request by sending a simple HTML page.
Java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class MyGetServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
out.println( "<html><body>" );
out.println( "<h2>Hello from MyGetServlet!</h2>" );
out.println( "</body></html>" );
}
}
|
2. Handling HTTP POST Request
In this example, the servlet responds to a POST request by reading form data and sending a customized response.
Java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class MyPostServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
String name = request.getParameter( "name" );
out.println( "<html><body>" );
out.println( "<h2>Hello, " + name + "!</h2>" );
out.println( "</body></html>" );
}
}
|
3. Handling Both GET and POST Requests
A servlet can handle both GET and POST requests by implementing both doGet and doPost methods.
Java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class MyServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
out.println( "<html><body>" );
out.println( "<h2>Hello from MyServlet (GET)!</h2>" );
out.println( "</body></html>" );
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
String name = request.getParameter( "name" );
out.println( "<html><body>" );
out.println( "<h2>Hello, " + name + "!</h2>" );
out.println( "</body></html>" );
}
}
|
Commonly Used Databases
- MySQL:
MySQL is really good at storing structured data in tables like your structured bookshelf where each database table is like a shelf, and each row on the database is a record containing specific information. MySQL is widely used and plays well with Java.
- PostgreSQL:
PostgreSQL is a bit like MySQL, but it’s known for handling complex queries and large datasets effectively.
- SQLite:
It’s lightweight and perfect for smaller projects somewhat like a portable notebook which you can carry around.
- MongoDB:
MongoDB is a NoSQL database, meaning it’s not restricted to tables like MySQL. It’s great for handling diverse types of data somewhat resembling a drawer where you can place any object.
Interaction with Java Code
Now, let’s talk about how these databases interact with Java code.
- JDBC (Java Database Connectivity)
- JDBC acts like a translator between Java and databases. It helps Java code communicate with databases seamlessly.
- It is like a person who knows multiple languages and communicates between two persons who are not able to communicate directly because of language barrier.
- Using JDBC in Java Code
- To interact with a database, you can use JDBC in your Java code. You can specify the database, the connection details and use JDBC methods to send queries to the database and get results back.
Example:
Here’s a simplified Java code snippet using JDBC to connect to a MySQL database and retrieve data.
Java
import java.sql.*;
public class DatabaseExample {
public static void main(String[] args) {
try {
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery( "SELECT * FROM My-Table" );
while (resultSet.next()) {
System.out.println(resultSet.getString( "column1" ) + " " + resultSet.getString( "column2" ));
}
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
|
Real-world Example:
Let’s build a simple servlet which handles users’ login functionality. The servlet should receive the basic credentials such as username and the password from a HTML form. It will validate the credentials and provide with a successful message when the credentials are validated. Below is the servlet code.
Java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
public class GFG extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter out = response.getWriter();
String username = request.getParameter( "username" );
String password = request.getParameter( "password" );
if ( "user123" .equals(username) && "pass123" .equals(password)) {
out.println( "<html><body>" );
out.println( "<h2>Welcome, " + username + "!</h2>" );
out.println( "</body></html>" );
} else {
out.println( "<html><body>" );
out.println( "<h2>Login failed. Please check your username and password.</h2>" );
out.println( "</body></html>" );
}
}
}
|
Explanation of the above Program:
The LoginServlet class indicates that it is a servlet. The doPost method handles the HTTP POST requests which typically is used in case of form submission. The request.getParameter method retrieves the user input from the HTML form which are then validated against some static string value. This can be fetched from the database as an object and can be matched against the usernames and passwords in real case. Depending upon the validation results, it will display the required result.
HTML Form (index.html):
Consider an HTML form (index.html) that sends a POST request to the servlet when the user enters the credentials.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Login Form</ title >
</ head >
< body >
< h2 >Login Form</ h2 >
< form action = "/your-web-app/login" method = "post" >
Username: < input type = "text" name = "username" >< br >
Password: < input type = "password" name = "password" >< br >
< input type = "submit" value = "Login" >
</ form >
</ body >
</ html >
|
Output:
- When a user submits the form with valid credentials (username=”user123″ and password=”pass123″), the servlet responds with a welcome message.
- When the credentials are incorrect, the servlet responds with an error message.
When to Choose a Framework
Choosing a framework is not a direct question which has a single answer. While considering the framework, you have to keep in mind several parameters. Based on different such parameters, below are the comparisons.
1. Flexibility
|
Spring Boot
|
Spring Boot lets you pick and choose the tools you need, making it flexible for different types of projects.
|
2. Simple:
|
Flask (for Python)
|
Flask is easy to understand and great for smaller projects or when you’re just starting.
|
3. Building a Big Web App
|
Django (for Python)
|
Django provides a lot of built-in features, making it efficient for larger projects.
|
4. Real-Time Web Apps
|
Node.js with Express
|
Node.js is great for real-time applications, and Express is like the power tool that makes it even faster.
|
5. Want Convention over Configuration
|
Java Spring MVC
|
Spring MVC follows conventions, so you spend less time deciding how things should be done.
|
6. Native Mobile Application
|
React Native (for JavaScript/React)
|
React Native lets you write code once and use it for different platforms like iOS and Android apps.
|
Share your thoughts in the comments
Please Login to comment...