Assertions are checks or validations we can include in our API requests to ensure that the response from the server meets certain criteria. These criteria could be based on the response’s status code, headers, body content, or any other aspect of the response. Postman provides a variety of built-in assertion options that we can use to validate the responses you receive during API testing. These assertions help us to confirm that our APIs are behaving as expected.
Assertions are mainly the tests that we want to execute after writing of code. In this case, when our APIs are ready, we just want to make sure that they are perfectly working or not. For this Postman provides us a Test section where we can write a test for the request. Postman test uses Chai Assertion Library BDD syntax.
Why use Assertions in Postman?
Postman gives us a whole environment for checking and testing the APIs we develop. And assertions are a 2 way of checking on it. Like if we want to check about the behaviour API then this is the best way to test it.
The section in Postman where we are going to work:
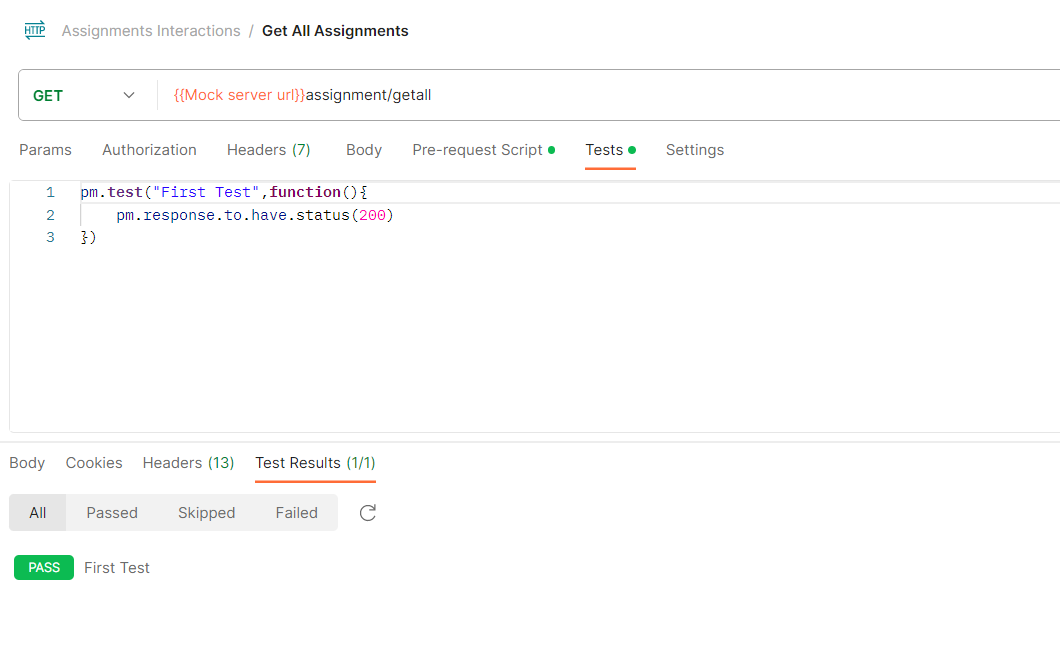
Test Section and its Result in Response Section
Like in the above API, we are testing whether this endpoint comes with a 200-status code or not. This might be the best way to figure out any error if we are working with many endpoints.
Types of Assertions in Postman
1. Checking the Status code or the status of the Request.
- For unauthorized users, it should give a 401-status code and for authorized it should give 200.
- If the Item is added to a database, then it should give 201.
- If any error is thrown, then a 500-status code is sent.
pm.test(“Content-Type is present”, function () {
pm.response.to.have.status(200);
});
2. Checking whether the response contains the data is in some constraints.
- If your API has filters, then the response should have only the data between those filters.
- After doing Login whether the response contains a JWT Token
3. Add Environment Variable.
- Accessing all the other APIs using one ID and the response contains that ID. So, Test can also add them into the environment variable.
var jsondata = pm.response.json();
pm.environment.set(“contactId”, jsondata._id);
4. Checking Headers and Cookies.
- If your response should have any particular Content-Type, then you can check it in tests.
- If the JWT token is passed in Cookies, then you can check it.
pm.test(“Content-Type is present”, function () {
pm.response.to.have.header(“Content-Type”,”application/json; charset=utf-8″);
});
How to Use Assertions in the Scripting Window?
Let’s say, for starters, we just want to ensure that whether the request is successful or not. We can know that by checking its status code. So the Expected outcome is that a Status Code should be 200.
pm.test(“First Test”,function(){
pm.response.to.have.status(200)
})
The name of the test is First Test and in the function, we checking its status which is expected to be 200. You can see the result in the Test Results section. If it shows Fails then it means that your response contains any other status code.
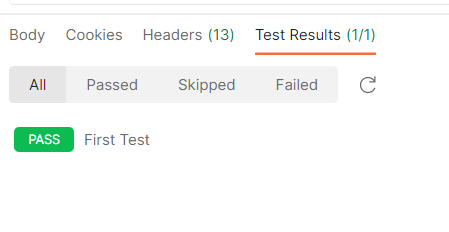
You can write as many tests as you want in the Tests section one after other as per your requirements. Let’s see one other example of a Test, it will test whether the response time is less than 200ms.
pm.test(“Response time is Less than 200ms”,function(){
//We are expecting responseTime to be less than 200
pm.expect(pm.response.responseTime).to.be.lessThan(200)
})
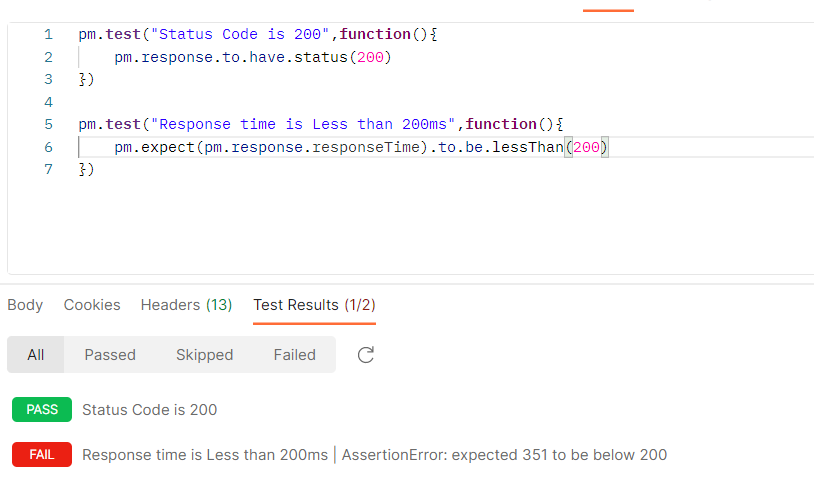
You can see that I get Two results in which the first one is Passed but the second Fails, so by this I can work on my API to reduce the response time. By this, Postman can help us to figure out the real-time problems that we could face in our application.
Using Chai Assertions in Postman
Chai is a JavaScript assertion library and it is used for creating expressive and flexible assertions in test scripts. It provides a wide range of assertion styles and methods to make our test assertions more readable and comprehensible.
We can use Chai assertions to create a chain, Example we want to verify that in our json response is there a property name “location” and if yes then is it an “India”, for this I can create a chain like
pm.expect(pm.response.json()).to.have.property(“location”).that.is.a(“India”);
Similarly, there are functions like “property” such as lengthOf(), include(), a() and many more let’s look at some of them with advanced assertions.
Advanced Assertions
We have already seen different assertions and they are the common assertions that are mainly used for testing, but now let’s look at some advanced assertions.
In Postman we are keen to test our response and we can get the Json response by pm.response.json(), using this the Json Data is returned and now we can validate any property or any object.
Let our response looks something like:
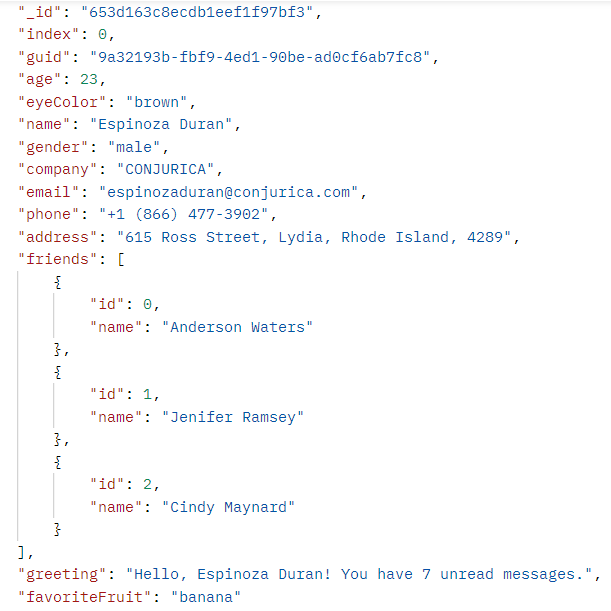
Response to test
1. Now, let’s start with checking whether the email is proper or not with the help of Regex Pattern
pm.test(“Regular Expression Matching”, function () {
const responseText = pm.response.json();
const regexPattern = /^[a-zA-Z0-9+_.-]+@[a-zA-Z0-9.-]+$/; // A pattern for a format like “ABC-123”
pm.expect(responseText.email).to.match(regexPattern);
});
2. Next, we are going to check if is there any greeting is there, we can check this by looking at the string that whether it contains the word “unread“.
pm.test(“Any Greeting”, function () {
const responseText = pm.response.json();
pm.expect(responseText.greeting).to.include(“unread”);
});
3. We have checked whether the email is valid or not, and now we will check whether the domain of mail is the same as the name of the company.
pm.test(“Email Domain and Company Name is Same”, function () {
const responseText = pm.response.json();
const email = responseText.email;
//Extracting the domain
const domain = email.split(‘@’)[1].split(‘.’)[0];
pm.expect(domain).to.equal(responseText.company.toLowerCase())
});
Conclusion
So, Assertions can be used for many reasons, and by using them we can assure ourselves regarding the different parameters of API. As discussed there are so many test cases and to ensure all is not always important but yes at different APIs we might need some important tests like checking status codes and checking responses. Also, we could write some common test cases into the collections which will be applied to all of the tests in those collections.
Share your thoughts in the comments
Please Login to comment...