What is Json Schema and How to Validate it with Postman Scripting?
Last Updated :
29 Jan, 2024
JSON stands for JavaScript Object Notation, is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate.
- It is often used to transmit data between a server and a web application.
- It supports data structures like arrays and objects and JSON documents that are rapidly executed on the server.
What is JSON Schema?
JSON Schema is a powerful tool for describing JSON data’s structure and validation rules. It provides a way to specify the expected format of JSON documents, ensuring that they meet certain criteria and adhere to a predefined schema. JSON Schema is particularly useful for validating API payloads and ensuring data consistency.
Key Concepts in JSON Schema:
- Properties: Define the properties that a JSON object should have.
- Types: Specify the data type expected for a particular property.
- Required: Indicate which properties are mandatory.
- Arrays: Define arrays and their constraints.
- Nested Objects: Describe nested JSON objects and their properties.
Why is JSON Schema Validation required?
JSON Schema Validation is essential for ensuring the correctness and quality of JSON data. It allows developers to define, validate, and document the structure and content of JSON data according to a set of predefined rules known as a schema. Let us understand its usage with following points:
- Consistency: It ensures that the JSON data follows the expected format, which is important when exchanging data between systems.
- Data Integrity: Validation helps to maintain the integrity of the data by preventing invalid or malicious data from being processed.
- Error Handling: It provides a mechanism for error handling by specifying which parts of the data are incorrect.
- Documentation: A JSON Schema serves as documentation for the data format which makes it easier for developers to understand and work with the data.
JSON Schema Validation
Postman supports the validation of JSON Schema using scripts. Let us discuss how to validate JSON schema in Postman:
Step 1: Install the Json schema library
You can install the library using the following command:
pip install jsonschema
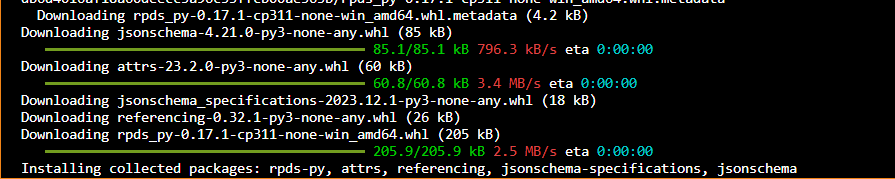
this is the output you will get after running this command.
Step 2: Create a JSON Schema
Create a simple JSON Schema file named schema.json that describes the structure we want our JSON data to have:
Javascript
{
"type" : "object" ,
"properties" : {
"name" : {
"type" : "string"
},
"age" : {
"type" : "integer" ,
"minimum" : 0
},
"email" : {
"type" : "string" ,
"format" : "email"
}
},
"required" : [ "name" , "age" ]
}
|
This schema defines an object with properties name (string), age (integer with a minimum value of 0), and an optional email (string with email format). The required keyword specifies that name and age are mandatory properties.
Step 3: Create a JSON Document
Create a JSON document named data.json that we want to validate against the schema:
Javascript
{
"name" : "Krishna Gupta" ,
"age" : 20,
"email" : "krishna.gupta@example.com"
}
|
Step 4: Validate JSON Document against Schema in Python
Write a Python script to validate the JSON document against the JSON schema using the jsonschema library:
Python
import json
from jsonschema import validate, ValidationError
with open ( "schema.json" ) as schema_file:
schema = json.load(schema_file)
with open ( "data.json" ) as data_file:
data = json.load(data_file)
try :
validate(instance = data, schema = schema)
print ( "Validation successful! The document is valid." )
except ValidationError as e:
print (f "Validation failed. Error: {e.message}" )
|
Step 5: Run the Python Script
Save the script in a file, for example, validate_json.py, and run it using the following command:
python validate_json.py

this is the output you will get after running this command.
Note:
- Ensure that the necessary npm packages are installed by running `npm install ajv` in the Postman Script’s sandbox.
- Adjust the script as needed based on your specific JSON Schema and API response structure.
- Remember to customize the script according to your specific requirements and JSON Schema.
Conclusion
In conclusion, JSON Schema serves as a robust framework for defining and validating the structure of JSON data. Its ability to articulate specific requirements ensures data integrity and consistency, especially in API development. By implementing JSON Schema validation within Postman, we enhance our testing processes, mitigating the risk of malformed responses and promoting adherence to predefined data standards.
Share your thoughts in the comments
Please Login to comment...