Backend development involves working on the server side of web applications, where the logic, database interactions, and server management take place. It focuses on handling data, processing requests from clients, and generating appropriate responses.
In this Top Backend Development interview questions, We cover Interview questions from all important topics from basic to advanced such as JavaScript, Node.js, Express.js, SQL, MongoDB, Django, PHP, Java Spring, and API. No matter whether you are a fresher or an experienced professional we have got questions that will enhance your skills and help you shine in your backend development interview.
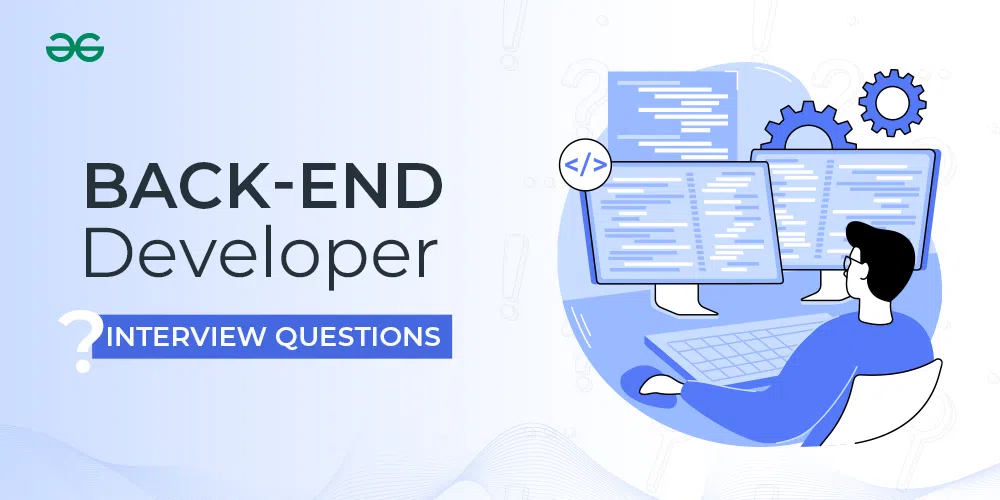
Backend Developer Interview Questions
Here, we have organized 85+ backend developer interview questions and answer based on different technologies, including:
Javascript Backend Interview Questions
In JavaScript, the ==
operator is used for equality comparison. It compares two values and returns true
if they are equal, and false
otherwise. However, it performs type coercion, which means it converts the operands to the same type before making the comparison.
Javascript
console.log(5 == '5'); // true, because '5' is converted to a number before comparison
console.log(5 == 5); // true, both values are of the same type and equal
console.log(5 == 6); // false, the values are not equal
In JavaScript, Function.prototype.bind()
is a method that allows you to create a new function with a specified this
value and optionally some initial arguments.
Syntax:
const newFunction = oldFunction.bind(thisArg, arg1, ag2, ..., argN)
Object.freeze()
:
Object.freeze()
is a method provided by JavaScript that freezes an object, making it immutable. This means that after calling Object.freeze()
on an object, you cannot add, delete, or modify any of its properties.- Even attempts to modify the properties of a frozen object will fail silently in non-strict mode and throw an error in strict mode.
Object.freeze()
operates on the object itself, making the object and its properties immutable.
const
:
const
is a keyword in JavaScript used to declare constants. When you declare a variable using const
, you cannot reassign it to a different value. However, this does not make the object itself immutable.- If the variable is an object, its properties can still be modified or reassigned, but you cannot assign a new object to the variable.
- In other words,
const
ensures that the variable reference cannot change, but it does not ensure immutability of the object itself.
In summary, Object.freeze()
is used to make an object and its properties immutable, while const
is used to create constants whose references cannot be reassigned, but it does not ensure immutability of the object itself.
IIFEs, which stands for Immediately Invoked Function Expressions, are JavaScript functions that are executed immediately after they are defined. They are commonly used to create a new scope and encapsulate code, preventing variable declarations from polluting the global scope.
Syntax:
(function (){
// Function Logic Here.
})();
.forEach()
:
- The
.forEach()
method is used to iterate over an array and execute a provided function once for each array element. - It does not mutate the original array and does not return a new array.
- The callback function passed to
.forEach()
can perform actions on each element of the array, but it does not produce a new array.
.map()
:
- The
.map()
method is used to iterate over an array and transform each element using a provided function. - It returns a new array with the results of calling the provided function on each element of the original array.
- The callback function passed to
.map()
should return a new value for each element, which will be included in the new array.
A cookie is an important tool as it allows you to store the user information as a name-value pair separated by a semi-colon in a string format. If we save a cookie in our browser then we can log in directly to the browser because it saves the user information.
- Create cookies: We can apply various operations on cookie-like create, delete, read, add an expiry date to it so that users can never be logged in after a specific time. A cookie is created by the document.cookie keyword.
- Read cookies: This function retrieves the cookie data stored in the browser. The cookie string is automatically encoded while sending it from the server to the browser.
- Clear cookies: Cookies expire, the date and time are specified in the “expires” attribute. As a result, the browser automatically deletes the cookies when the date and time exceed the expiration date (and time).
JavaScript closure is a feature that allows inner functions to access the outer scope of a function. Closure helps in binding a function to its outer boundary and is created automatically whenever a function is created.
Javascript
function foo(outer_arg) {
function inner(inner_arg) {
return outer_arg + inner_arg;
}
return inner;
}
let get_func_inner = foo(5);
console.log(get_func_inner(4));
console.log(get_func_inner(3));
Explanation:
The foo
function returns an inner function that adds its argument to the outer function’s argument. It creates a closure, preserving the outer function’s state. Output: 9, 8.
Arrow function {()=>} is concise way of writing JavaScript functions in shorter way. Arrow functions were introduced in the ES6 version. They make our code more structured and readable. Mostly in development phase mostly arrow function is used.
Syntax:
const gfg = () => {
console.log( "Hi Geek!" );
}
gfg() // output will be Hi Geek!
Exports:
- Exports are used to expose functionality from a module to other parts of the program.
- You can export variables, functions, classes, or any other JavaScript entity by using the
export
keyword. - There are different ways to export:
- Default Export:
export default myFunction;
- Named Export:
export const myVariable = 10;
- Named Exports from Expressions:
export { myFunction };
- You can have multiple exports in a single module.
Imports:
- Imports are used to bring functionality from other modules into the current module.
- You can import exported entities using the
import
keyword. - You can import default exports like this:
import myFunction from './module';
- You can import named exports like this:
import { myVariable } from './module';
- You can also import all named exports using the
* as
syntax: import * as module from './module';
JavaScript operators operate the operands, these are symbols that are used to manipulate a certain value or operand. Operators are used to performing specific mathematical and logical computations on operands.
Nodejs Backend Developer Interview Questions
Node.js is a JavaScript runtime environment that allows developers to run JavaScript code outside of a web browser. It uses the V8 JavaScript engine, which is the same engine used by Google Chrome, to execute JavaScript code on the server-side.
Node.js works by providing an event-driven, non-blocking I/O model that allows for highly efficient and scalable server-side applications. It utilizes asynchronous programming techniques to handle multiple requests simultaneously without blocking the execution of other code. Node.js is particularly well-suited for building real-time web applications, APIs, and microservices. It also comes with a vast ecosystem of libraries and frameworks that extend its capabilities for various use cases.
Modules are the blocks of reusable code consisting of Javascript functions and objects which communicate with external applications based on functionality. A package is often confused with modules, but it is simply a collection of modules (libraries).
To use async await in Nodejs:
- Define an asynchronous function with the
async
keyword. - Use the
await
keyword within the function to pause execution until promises are resolved. - Handle errors using
try/catch
blocks. - Call the asynchronous function and use
await
to wait for its result.
Example: After async/await
async function fun1(req, res){
let response = await request.get('http://localhost:3000');
if (response.err) { console.log('error');}
else { console.log('fetched response');
}
Streams are one of the fundamental concepts of Node.js. Streams are a type of data-handling methods and are used to read or write input into output sequentially. Streams are used to handle reading/writing files or exchanging information in an efficient way.
Accessing Streams:
const stream = require('stream');
const fs = require("fs");
const readablestream = fs.createReadStream("source.txt"); // the txt file in which data is available
const writeablestream = fs.createWriteStream("destination.txt"); // the txt to which we want to copy the data
readablestream.pipe(writeablestream);
readablestream.on("error" , (err)=>{
console.log(err); // this will handle the error due to source.txt(if any error will be place)
}
writeablestream.on("error" , (err)=>{
console.log(err);// this will handle the error due to source.txt(if any error will be place)
}
writeablestream.on("finish" , ()=>{
console.log("data from source.txt is successfully copied to destination.txt");
}
Node.js is a cross-platform, open-source back-end JavaScript runtime environment that uses the V8 engine to execute JavaScript code outside of a web browser. Exit codes in Node.js are a specific group of codes that finish off processes, which can include global objects as well.
A small program routine that substitutes for a longer program which is possible to be loaded later or that is remotely located.
Features of stub:
- Stubs can be either anonymous.
- Stubs can be wrapped into existing functions. When we wrap a stub into the existing function the original function is not called.
Clustering is the process through which we can use multiple cores of our central processing unit at the same time with the help of Node JS, which helps to increase the performance of the software and also reduces its time load.
We can install cluster modules through the given command.
npm i cluster
WASI also called as Web assembly system interface , it is like a bridge between operating system and web assembly , let me explain Web Assembly , see the browser only understand javascript so if any user sends a c++ or rust code on the browser so for translating that code web assembly is used , so web Assembly is like a translator which convert one language of code to the other.
so WASI provides a standard way to talk to the outside world like operating system so that so that it can ask to read the file or perform the other operations .
Asynchronous operation in Node.js is a non-blocking operation which means if we perform an asynchronous operation at a certain point in code then the code after that is executed and does not wait for this asynchronous operation to complete.
Syntax:
const calcTime = async () => {
const start = Date.now();
await someAsyncOperation();
const end = Date.now()
const duration = end - start;
}
A thread pool is a collection of worker threads that are used to execute asynchronous tasks concurrently in a multithreaded environment. Each task is assigned to a worker thread from the pool, allowing for efficient parallel execution of tasks without creating a new thread for each task.
In Node.js, the libuv
library handles the thread pool. libuv
is a cross-platform library responsible for asynchronous I/O operations, including file system operations, network operations, and timers. It manages the thread pool to handle these asynchronous tasks efficiently.
Expressjs Backend Developer Interview Questions
Express.js is a routing and Middleware framework for handling the different routing of the webpage and it works between the request and response cycle. Middleware gets executed after the server receives the request and before the controller actions send the response.
The basic syntax for the middleware functions are as follows –
app.get(path, (req, res, next) => {}, (req, res) => {})
In Express.js, request and response objects are fundamental to handling HTTP requests and generating HTTP responses. Here’s how Express.js handles these objects:
- Request Object (req):
- The request object (
req
) represents the HTTP request received from the client. It contains information about the request, such as the HTTP method, URL, headers, query parameters, request body, and more. - Express populates the request object with this information and passes it to route handlers and middleware functions.
- Response Object (res):
- The response object (
res
) represents the HTTP response that Express sends back to the client. It allows developers to send back data, set HTTP headers, and control the response status code. - Express provides methods on the response object to send different types of responses, such as sending JSON data (
res.json()
), sending HTML (res.send()
), redirecting the client to a different URL (res.redirect()
), setting HTTP headers (res.setHeader()
), and more.
Node.js server code sets up a RESTful API for managing data. It provides endpoints for performing CRUD (Create, Read, Update, Delete) operations on a collection of records. The server uses the Express.js framework to handle HTTP requests.
24. What is the difference between a traditional server and an Express.js server?
Traditional server
| Express.js server
|
---|
Typically, traditional servers are built using various server-side technologies and frameworks, such as Java with Spring, Python with Django, Ruby on Rails, ASP.NET, etc. | Express.js is a minimal and flexible Node.js web application framework. It is specifically designed for building web applications and APIs using JavaScript on the server side. |
Traditional servers may use a synchronous or asynchronous programming model, depending on the underlying technology. | Express.js is built on top of Node.js, which uses an event-driven, non-blocking I/O model. Asynchronous programming is fundamental to Node.js and, by extension, to Express.js. |
Frameworks like Spring or Django often come with built-in middleware and routing systems. | Express.js also provides middleware and routing, but it has a more lightweight and flexible approach. Middleware functions can be added to the request-response cycle to perform specific tasks. |
Different server-side frameworks have their own communities, ecosystems, and plugins. The size and activity of these communities can vary depending on the framework. | Express.js has a large and active community. It is one of the most popular Node.js frameworks, and as such, it benefits from a rich ecosystem of middleware, plugins, and extensions. |
A JSON web token(JWT) is JSON Object which is used to securely transfer information over the web(between two parties). It can be used for an authentication system and can also be used for information exchange. The token is mainly composed of header, payload, signature.
Publisher Subscriber basically known as Pub-Sub is an asynchronous message-passing system that solves the drawback above. The sender is called the publisher whereas the receiver is called the subscriber. The main advantage of pub-sub is that it decouples the subsystem which means all the components can work independently.
POST parameter can be received from a form using express.urlencoded() middleware and the req.body Object. The express.urlencoded() middleware helps to parse the data that is coming from the client-side.
Example: Here, is the code to get post a query in Express.js
Javascript
const express = require('express');
const app = express();
// Parse incoming request bodies with urlencoded payloads
app.use(express.urlencoded({ extended: true }));
// POST route to handle form submission
app.post('/submit', (req, res) => {
// Extract data from the request body
const { name, email } = req.body;
// Process the data (in this example, just logging it)
console.log('Received form submission:');
console.log('Name:', name);
console.log('Email:', email);
// Respond with a success message
res.send('Form submitted successfully');
});
// Start the server
const port = process.env.PORT || 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Explanation:
- We use
app.use(express.urlencoded({ extended: true }))
to enable the express.urlencoded()
middleware, which parses incoming request bodies with application/x-www-form-urlencoded
format. - We define a POST route
/submit
to handle form submissions. Inside the route handler, we extract the name
and email
parameters from the req.body
object, which is populated by the express.urlencoded()
middleware. - We process the received data (in this example, just logging it to the console).
- We respond with a success message using
res.send()
.
Scaffolding is creating the skeleton structure of application. It allows users to create own public directories, routes, views etc. Once the structure for app is built, user can start building it.
Syntax:
npm install express --save
Error handling in Express.js refers to the process of capturing and responding to errors that occur during the execution of an Express application. In Express, you can handle errors using middleware functions, which are functions that have access to the request and response objects, as well as the next middleware function in the application’s request-response cycle.
Template Engine : A template engine basically helps us to use the static template files with minimal code. At runtime, the template engine replaces all the variables with actual values at the client-side.
To use templating with Express.js in Node.js:
- Install a template engine like EJS (
npm install ejs
). - Set up Express to use the template engine (
app.set('view engine', 'ejs')
). - Create EJS templates in the
views
directory. - Render EJS templates in Express routes using
res.render()
. - Pass dynamic data to the templates.
- Start the Express server.
SQL Backend Developer Interview Questions
31. What is the difference between LEFT JOIN with WHERE clause & LEFT JOIN?
LEFT JOIN with WHERE Clause:
SELECT *
FROM table1
LEFT JOIN table2 ON table1.column = table2.column
WHERE table2.column IS NULL;
Explanation:
This query performs a LEFT JOIN between table1 and table2 based on the specified column.
The WHERE clause filters the results to only include rows where there is no match in table2 (i.e., table2.column IS NULL).
It effectively retrieves records from table1 and the matching records from table2, where no match is found, the columns from table2 will be NUL.
Regular LEFT JOIN:
SELECT *
FROM table1
LEFT JOIN table2 ON table1.column = table2.column;
Explanation:
This query performs a standard LEFT JOIN between table1 and table2 based on the specified column.
It retrieves all records from table1, and for each record, it includes matching records from table2. If no match is found in table2, the columns from table2 will be NULL.
Summary:
The primary difference lies in the WHERE clause of the first query. The LEFT JOIN with a WHERE clause specifically filters the results to include only the rows where no match is found in the right table (table2). The regular LEFT JOIN, on the other hand, retrieves all records from the left table (table1) along with matching records from the right table (table2), including NULLs where no match is found.
In summary, the LEFT JOIN with a WHERE clause is often used when you want to filter out rows with matches in the right table, effectively isolating the unmatched rows from the left table.
32.How do you prevent SQL Server from giving you informational messages during and after a SQL statement execution?
In SQL Server, informational messages, often called “info” or “print” messages, can be generated during and after the execution of a SQL statement. These messages might include details such as the number of rows affected or certain warnings. If you want to suppress these informational messages, you can use the SET command with the NOCOUNT option.
Here’s an example of how to use it:
SET NOCOUNT ON;
-- Your SQL statements go here
SET NOCOUNT OFF;
Explanation:
- SET NOCOUNT ON;: This setting turns off the “row affected” informational messages for subsequent statements in the same batch.
- SET NOCOUNT OFF;: This setting turns the informational messages back on.
By setting NOCOUNT ON, you prevent SQL Server from returning the “X rows affected” message for each statement in your batch. This can be useful in situations where you want to reduce the amount of information returned by the server, especially in scenarios where large volumes of data are processed.
Remember to turn NOCOUNT back to OFF if you want to receive informational messages for subsequent statements.
Cursor is a Temporary Memory or Temporary Work Station. It is Allocated by Database Server at the Time of Performing DML(Data Manipulation Language) operations on the Table by the User. Cursors are used to store Database Tables.
There are 2 types of Cursors: Implicit Cursors, and Explicit Cursors. These are explained as following below.
- Implicit Cursors: Implicit Cursors are also known as Default Cursors of SQL SERVER. These Cursors are allocated by SQL SERVER when the user performs DML operations.
- Explicit Cursors: Explicit Cursors are Created by Users whenever the user requires them. Explicit Cursors are used for Fetching data from Table in Row-By-Row Manner.
34. How SQL Server executes a statement with nested subqueries?
When SQL Server processes a statement with nested subqueries, the execution process involves evaluating each subquery from the innermost to the outermost level. The general steps for executing a statement with nested subqueries are as follows:
- Parsing and Compilation:
The SQL Server query processor parses the SQL statement and compiles it into an execution plan. - Innermost Subquery Execution:
SQL Server begins executing the innermost subquery first.
The result of the innermost subquery is typically a set of values or a single value that will be used in the next level of the query. - Intermediate Result Storage:
If the result of the innermost subquery is a set of values, SQL Server may store these values in temporary structures or tables in memory or on disk. - Propagation to the Next Level:
The result or intermediate results obtained from the innermost subquery are then used in the next level of the query, which could be a higher-level subquery or the main outer query. - Execution of Higher-Level Subqueries or Main Query:
The process repeats for higher-level subqueries or the main outer query, using the intermediate results obtained from the lower levels. - Combination of Results:
As the execution progresses from the innermost to the outermost levels, the results from each level are combined to produce the final result set. - Query Optimization:
SQL Server’s query optimizer may perform various optimization techniques, such as reordering joins or selecting the most efficient indexes, to enhance the overall query performance.
It’s important to note that SQL Server’s query optimizer aims to find the most efficient execution plan for the entire query, taking into account the structure of the query, the available indexes, and the distribution of data in the tables involved. The goal is to minimize the time and resources required to execute the query.
Developers and database administrators can use tools like SQL Server Management Studio (SSMS) to analyze and optimize query execution plans, ensuring that nested subqueries are efficiently processed. Additionally, appropriate indexing and schema design can significantly impact the performance of queries with nested subqueries.
35. What is a deadlock and what is a live lock? How will you go about resolving deadlocks?
- Deadlock:A deadlock in database systems occurs when two or more transactions are blocked indefinitely, each waiting for the other to release a resource, resulting in a state where no progress can be made. It’s a situation where transactions are essentially stuck and cannot proceed.
- Live Lock: A live lock is a scenario where two or more transactions are actively trying to resolve a conflict, but their efforts prevent the conflict resolution from progressing. In other words, the transactions are not blocked, but they are caught in a loop of trying to resolve the situation without success.
- Deadlock Prevention: Design the system in a way that makes it impossible for a deadlock to occur. This involves careful resource allocation and transaction scheduling.
- Deadlock Detection and Resolution: Implement a deadlock detection mechanism that identifies when a deadlock has occurred.
Use techniques like timeout mechanisms or periodic checks to detect deadlocks.
Once a deadlock is detected, choose one of the transactions as a “victim” and roll back its changes, allowing the other transactions to proceed.- Transaction Timeout:
Set a maximum time limit for transactions. If a transaction cannot complete within the specified time, it will be automatically rolled back, preventing indefinite blocking. - Resource Allocation Order:
Define a standard order for acquiring resources, and ensure that transactions request resources in the same order. This reduces the chances of circular waits. - Lock Hierarchy:
Establish a hierarchy for resource locking. A transaction can only request resources at a lower level in the hierarchy than the ones it currently holds. This helps prevent circular waits. - Avoidance Algorithms:
Use resource allocation algorithms that predict whether a particular resource allocation will lead to a deadlock. These algorithms can help make decisions on resource requests based on the likelihood of deadlock.
Resolving Live Locks:
Identification:
Monitor the system for signs of live locks. This may involve analyzing system logs or using tools for performance monitoring.
- Adjust Transaction Logic: Review the logic of transactions involved and make adjustments to prevent continuous conflicts. This might involve using retries with backoff strategies.
- Use Randomized Delays: Introduce randomized delays in transactions to break the cycles that cause live locks.
- Concurrency Control Mechanisms: Review and optimize the concurrency control mechanisms in use, such as locking and isolation levels, to minimize the chances of live locks.
- Logging and Monitoring: Implement comprehensive logging and monitoring to capture information about live locks and facilitate analysis.
- Correct Underlying Issues: Identify and address any underlying issues in the application or database schema that contribute to live locks.
It’s important to note that both deadlocks and live locks are complex issues, and their resolution often involves a combination of careful system design, configuration, and monitoring. The chosen approach may vary based on the specific characteristics of the application and the database system in use.
Collations in SQL Server provide sorting rules, case, and accent sensitivity properties to data. A collation defines bit patterns that represent each character in metadata of database. SQL Server supports storing objects that have different collations in database. The options associated with collation are mentioned below :
- Case-sensitive (_CS)
- Accent-sensitive (_AS)
- Kana-sensitive (_KS)
- Width-sensitive (_WS)
- Variation-selector-sensitive (_VSS)a
- Binary (_BIN)
- Binary-code point (_BIN2)
37. Where is the MyISAM table stored?
In MySQL, the MyISAM storage engine stores tables in files on the file system. Each MyISAM table is represented by three files with the same base name but different extensions. These files are typically stored in the database directory associated with the MySQL server.
- .frm File: The .frm file is a binary file that stores the table definition, including the column names, types, and other metadata.
It is located in the MySQL database directory and is essential for MySQL to understand the structure of the table. - .MYD File (MyISAM Data): The .MYD file contains the actual data of the MyISAM table.
Each MyISAM table has its corresponding .MYD file, which holds the rows of the table.
The data is stored in a non-compressed format, and the file is organized as a heap structure. - .MYI File (MyISAM Index): The .MYI file contains the indexes for the MyISAM table.
Each index created on the table is stored in this file.
The index file is a B-tree (balanced tree) structure that allows for efficient lookups based on indexed columns.
Location of MyISAM Table Files:
- The default location for storing MyISAM table files is in the MySQL data directory.
- The MySQL data directory is specified in the MySQL configuration file (my.cnf or my.ini).
- Common default locations include /var/lib/mysql/ on Unix/Linux systems and C:\ProgramData\MySQL\MySQL Server X.Y\data\ on Windows.
File-Level Locking:
- MyISAM uses table-level locking, meaning that when a write operation (like an UPDATE or DELETE) is performed, the entire table is locked.
- This can lead to contention in scenarios with concurrent write operations on the same table.
Maintenance and Optimization:
- MyISAM tables may require periodic maintenance and optimization to reclaim disk space and improve performance.
- The OPTIMIZE TABLE command can be used to defragment and optimize MyISAM tables.
Considerations:
While MyISAM has been widely used, especially in earlier versions of MySQL, it lacks some features offered by the InnoDB storage engine, such as support for transactions and foreign keys.
InnoDB is the default storage engine in recent MySQL versions, and it is often recommended for new projects due to its reliability and additional features.
It’s crucial to note that the choice of storage engine depends on the specific requirements of your application, and InnoDB is often preferred for its support of ACID transactions and better handling of concurrency. If you have a choice, consider evaluating whether InnoDB is a better fit for your use case.
MongoDB Backend Developer Interview Questions
BSON stands for Binary JSON. It is a binary file format that is used to store serialized JSON documents in a binary-encoded format.The MongoDB database had several scalar data formats that were of special interest only for MongoDB, hence they developed the BSON data format to be used while transferring files over the network.
Replication – is the method of duplication of data across multiple servers. For example, we have an application and it reads and writes data to a database and says this server A has a name and balance which will be copied/replicate to two other servers in two different locations.
Sharding – is a method for allocating data across multiple machines. MongoDB used sharding to help deployment with very big data sets and large throughput the operation. By sharding, you combine more devices to carry data extension and the needs of read and write operations.
MongoDB provides you read operations to retrieve embedded/nested documents from the collection or query a collection for a embedded/nested document. You can perform read operations using the db.collection.find() method.
41. Can you create an index on an array field in MongoDB? If yes, what happens in this case?
Yes , You can create an index on a field containing an array value to improve performance for queries on that field. When you create an index on a field containing an array value, MongoDB stores that index as a multikey index.
To create an index, use the db.collection.createIndex() method.
Syntax:
db.<collection>.createIndex( { <field>: <sortOrder> } )
The example uses a students collection that contains these documents:
Javascript
db.students.insertMany( [
{
"name": "Andre Robinson",
"test_scores": [ 88, 97 ]
},
{
"name": "Wei Zhang",
"test_scores": [ 62, 73 ]
},
{
"name": "Jacob Meyer",
"test_scores": [ 92, 89 ]
}
] )
You regularly run a query that returns students with at least one test_score greater than 90. You can create an index on the test_scores field to improve performance for this query.
Procedure: The following operation creates an ascending multikey index on the test_scores field of the students collection:
db.students.createIndex( { test_scores: 1 } )
Because test_scores contains an array value, MongoDB stores this index as a multikey index.
42. What is Shard Key in MongoDB and how does it affect development process?
Shard key, which is a unique identifier that is used to map the data to its corresponding shard. When a query is received, the system uses the shard key to determine which shard contains the required data and then sends the query to the appropriate server or node.
Every document in the collection has an “_id” field that is used to uniquely identify the document in a particular collection it acts as the primary key for the documents in the collection. “_id” field can be used in any format and the default format is ObjectId of the document.
Format of ObjectId:
ObjectId(<hexadecimal>)
44. What is a Covered Query in MongoDB?
A covered query in MongoDB is a type of query where all the fields needed for the query are covered by an index. In other words, MongoDB can fulfill the query entirely using the index without needing to examine the actual documents in the collection. This is advantageous in terms of performance because it reduces the number of document reads necessary to satisfy the query.
For a query to be considered covered, the following conditions must be met:
- Projection: The query must include a projection that only selects fields covered by the index. A projection specifies which fields to include or exclude in the query results.
- Index: The fields specified in the query’s filter criteria must be part of an index. The fields specified in the projection must be part of the same index.
- No Additional Document Fields: The query should not include additional fields or expressions that are not covered by the index. If additional fields are needed, the index might not cover the entire query.
Example:
Consider a collection named employees with the following documents:
{ "_id": 1, "name": "Alice", "age": 30, "department": "HR" }
{ "_id": 2, "name": "Bob", "age": 35, "department": "IT" }
{ "_id": 3, "name": "Charlie", "age": 28, "department": "HR" }
Let’s say you have an index on the “department” field:
db.employees.createIndex({ "department": 1 });
Now, if you perform a query like this:
db.employees.find({ "department": "HR" }, { "_id": 0, "name": 1 });
This query is considered covered because:
- The filter criteria ({ “department”: “HR” }) matches the indexed field.
- The projection ({ “_id”: 0, “name”: 1 }) includes only the “name” field, which is also part of the index.
As a result, MongoDB can satisfy the query by scanning the index without needing to access the actual documents.
Keep in mind that covered queries are beneficial for performance because they minimize the amount of data that needs to be read from disk. However, not all queries can be covered, and the decision to create specific indexes should be based on the most common and performance-critical queries in your application.
In MongoDB, a relationship represents how different types of documents are logically related to each other. Relationships like one-to-one, one-to-many, etc., can be represented by using two different models:
- Embedded document model
- Reference model
The CAP theorem, originally introduced as the CAP principle, can be used to explain some of the competing requirements in a distributed system with replication. It is a tool used to make system designers aware of the trade-offs while designing networked shared-data systems.
MongoDB uses indexing in order to make the query processing more efficient. If there is no indexing, then the MongoDB must scan every document in the collection and retrieve only those documents that match the query. Indexes are special data structures that stores some information related to the documents such that it becomes easy for MongoDB to find the right data file.
Django Backend Developer Interview Questions
Django is a Python-based web framework which allows you to quickly create web application without all of the installation or dependency problems that you normally will find with other frameworks. Django is based on MVT (Model-View-Template) architecture. MVT is a software design pattern for developing a web application.
Templates are the third and most important part of Django’s MVT Structure. A template in Django is basically written in HTML, CSS, and Javascript in a .html file. Django framework efficiently handles and generates dynamic HTML web pages that are visible to the end-user.
Django lets us interact with its database models, i.e. add, delete, modify, and query objects, using a database-abstraction API called ORM(Object Relational Mapper).Django’s Object-Relational Mapping (ORM) system, a fundamental component that bridges the gap between the database and the application’s code.
51. How to get a particular item in the Model?
In Django, you can retrieve a particular item (a specific record or instance) from a model using the model’s manager and a query.
Assuming you have a Django model named YourModel defined in an app named your_app:
- Import the Model: Make sure to import your model in the Python file where you need to retrieve the item.
from your_app.models import YourModel
- Use the Model’s Manager: Every Django model comes with a default manager called objects. You can use this manager to perform queries on the model.
- Perform the Query: Use a query to filter the items based on the criteria you want. For example, if you want to retrieve an item by its primary key (id field), you can use the get method.
*Remember to replace YourModel with the actual name of your model and adjust the query criteria based on the fields of your model that you want to use for retrieval. If you need to retrieve multiple items based on certain conditions, you can use the filter method instead of get.
In Django, select_related and prefetch_related are designed to stop the deluge of database queries that are caused by accessing related objects.
- select_related() “follows” foreign-key relationships, selecting additional related-object data when it executes its query.
- prefetch_related() does a separate lookup for each relationship and does the “joining” in Python.
A QuerySet is a collection of database queries to retrieve data from your database. It represents a set of records from a database table or a result of a database query. Query sets are lazy, meaning they are not evaluated until you explicitly request the data, which makes them highly efficient.
Django Field Choices. According to documentation Field Choices are a sequence consisting itself of iterables of exactly two items (e.g. [(A, B), (A, B) …]) to use as choices for some field.Choices limits the input from the user to the particular values specified in models.py. If choices are given, they’re enforced by model validation and the default form widget will be a select box with these choices instead of the standard text field.
In Django, views are Python functions which take a URL request as parameter and return an HTTP response or throw an exception like 404. Each view needs to be mapped to a corresponding URL pattern. This is done via a Python module called URLConf(URL configuration).
Here’s a sample code for books/urls.py:
Python
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('books.urls')),
]
Django Views are one of the vital participants of MVT Structure of Django. As per Django Documentation, A view function is a Python function that takes a Web request and returns a Web response. This response can be the HTML contents of a Web page, or a redirect, or a 404 error, or an XML document, or an image, anything that a web browser can display.
A Django model is the built-in feature that Django uses to create tables, their fields, and various constraints. In short, Django Models is the SQL Database one uses with Django. SQL (Structured Query Language) is complex and involves a lot of different queries for creating, deleting, updating, or any other stuff related to a database.
Example: Below is an example of Django models.
Python
from django.db import models
# Create your models here.
class GeeksModel(models.Model):
title = models.CharField(max_length = 200)
description = models.TextField()
PHP Backend Developer Interview Questions
The error_reporting() function sets the error_reporting directive at runtime. PHP has many levels of errors, using this function sets that level for the duration (runtime) of your script. If the optional error_level is not set, error_reporting() will just return the current error reporting level.
The explode() function is an inbuilt function in PHP used to split a string into different strings. The explode() function splits a string based on a string delimiter, i.e. it splits the string wherever the delimiter character occurs. This function returns an array containing the strings formed by splitting the original string.
Syntax:
array explode(separator, OriginalString, NoOfElements)
The array_walk() function is an inbuilt function in PHP. The array_walk() function walks through the entire array regardless of pointer position and applies a callback function or user-defined function to every element of the array. The array element’s keys and values are parameters in the callback function.
Syntax:
boolean array_walk($array, myFunction, $extraParam)
A web browser may be the client, and an application on a computer that hosts a website may be the server. A client (browser) submits an HTTP request to the server; then the server returns a response to the client. The response contains status information about the request and may also contain the requested content.
- GET: Requests data from a specified resource.
- POST: Submits data to be processed to a specified resource.
- == Operator: This operator is used to check the given values are equal or not. If yes, it returns true, otherwise it returns false.
Syntax:
operand1 == operand2
- === Operator: This operator is used to check the given values and its data type are equal or not. If yes, then it returns true, otherwise it returns false.
Syntax:
operand1 === operand2
The Model-View-Controller (MVC) framework is an architectural/design pattern that separates an application into three main logical components Model, View, and Controller. Each architectural component is built to handle specific development aspects of an application. It isolates the business logic and presentation layer from each other.
PHP exit() Function: In PHP, the exit() function prints a message and exits the application. It’s often used to print a different message in the event of a mistake. Use exit() when there is not an error and have to stop the execution.
Syntax:
exit("Message goes here");
or
exit();
Example:
exit("This request is processed");
PHP die() Function: In PHP, die() is the same as exit(). A program’s result will be an empty screen. Use die() when there is an error and have to stop the execution.
Syntax:
die("Message goes here");
or
die();
Example:
die('Oops! Something went wrong');
The stdClass is the empty class in PHP which is used to cast other types to object. It is similar to Java or Python object. The stdClass is not the base class of the objects. If an object is converted to object, it is not modified. But, if object type is converted/type-casted an instance of stdClass is created, if it is not NULL. If it is NULL, the new instance will be empty.
When you don’t want to have more than a single instance of a given class, then the Singleton Design Pattern is used and hence the name is – Singleton. Singleton is the design patterns in PHP OOPs concept that is a special kind of class that can be instantiated only once. If the object of that class is already instantiated then, instead of creating a new one, it gets returned.
Spring Boot is built on the top of the spring and contains all the features of spring. And is becoming a favorite of developers these days because of its rapid production-ready environment which enables the developers to directly focus on the logic instead of struggling with the configuration and setup.
Java Spring Backend Developer Interview Questions
- Modularity: Lightweight and modular design.
- Inversion of Control (IoC): Manages object lifecycle and reduces coupling.
- Aspect-Oriented Programming (AOP): Modularizes cross-cutting concerns.
- Dependency Injection (DI): Promotes loose coupling and testability.
- Transaction Management: Simplifies database transactions.
- Integration: Seamlessly integrates with existing technologies.
- Enterprise Features: Provides support for security, caching, messaging, etc.
- Testability and Maintainability: Promotes unit testing and modular design.
A Spring Boot Multi-Module Project is a project structure where you organize your codebase into multiple modules, each representing a different functional or logical unit of your application. Spring Boot, a popular Java framework, provides support for creating and managing multi-module projects.
In a multi-module project, you typically have a parent module (also known as the aggregator module) and one or more child modules. The parent module coordinates the build process and manages the dependencies between the child modules.
Dependency Injection is the main functionality provided by Spring IOC(Inversion of Control). The Spring-Core module is responsible for injecting dependencies through either Constructor or Setter methods.The design principle of Inversion of Control emphasizes keeping the Java classes independent of each other and the container frees them from object creation and maintenance.
The BeanFactory Interface
This is the root interface for accessing a Spring bean container. It is the actual container that instantiates, configures, and manages a number of beans. These beans collaborate with one another and thus have dependencies between themselves.
Java
ClassPathResource resource = new ClassPathResource("beans.xml");
XmlBeanFactory factory = new XmlBeanFactory(resource);
The ApplicationContext Interface
This interface is designed on top of the BeanFactory interface. The ApplicationContext interface is the advanced container that enhances BeanFactory functionality in a more framework-oriented style.
Java
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Bean life cycle is managed by the spring container. When we run the program then, first of all, the spring container gets started. After that, the container creates the instance of a bean as per the request, and then dependencies are injected. And finally, the bean is destroyed when the spring container is closed.
The context path is a prefix to the URL path used to identify and differentiate between different context(s). In Spring Boot, by default, the applications are accessed by context path “/”. That means we can access the application directly at http://localhost:PORT/. For example
http://localhost:8080/
- @Around: This is the most effective advice among all other advice. The first parameter is of type ProceedingJoinPoint. Code should contain proceed() on the ProceedingJoinPoint and it causes the underlying lines of code to execute.
- @Before: This advice will run as a first step if there is no @Around advice. If @Around is there, it will run after the beginning portion of @Around.
- @After: This advice will run as a step after @Before advice if there is no @Around advice. If @Around is there, it will run after the ending portion of @Around.
- @AfterReturning: This advice will run as a step after @After advice. Usually, this is the place , where we need to inform about the successful resultant of the method.
The Spring container detects those dependencies specified in the configuration file and @ the relationship between the beans. This is referred to as autowiring in Spring. An autowired application requires fewer lines of code comparatively but at the same time, it provides very little flexibility to the programmer.
Spring Security is a framework that allows a programmer to use JEE components to set security limitations on Spring-framework-based Web applications. In a nutshell, it’s a library that can be utilized and customized to suit the demands of the programmer.
Spring IoC Container is the core of Spring Framework. It creates the objects, configures and assembles their dependencies, manages their entire life cycle. The Container uses Dependency Injection(DI) to manage the components that make up the application.
API Backend Developer Interview Questions
API is an abbreviation for Application Programming Interface which is a collection of communication protocols and subroutines used by various programs to communicate between them. A programmer can make use of various API tools to make their program easier and simpler.
Representational State Transfer (REST) is an architectural style that defines a set of constraints to be used for creating web services. REST API is a way of accessing web services in a simple and flexible way without having any processing. REST technology is generally preferred to the more robust Simple Object Access Protocol (SOAP) technology.
URI stands for Uniform Resource Identifier. It is a technical term that used for the names of all resources Connected to the World Wide Web. URIs established the protocols over the internet to conduct the connection between among resources.
RESTful web services are generally highly scalable, light, and maintainable and are used to create APIs for web-based applications. It exposes API from an application in a secure and stateless manner to the client. The protocol for REST is HTTP.
REST is a software architectural style that defines the set of rules to be used for creating web services. Web services which follow the REST architectural style are known as RESTful web services. There are six architectural constraints which makes any web service are listed below:
- Uniform Interface
- Stateless
- Cacheable
- Client-Server
- Layered System
- Code on Demand
HTTP PUT Request
HTTP PUT is a request method supported by HTTP used by the World Wide Web. The PUT method requests that the enclosed entity be stored under the supplied URI.
Python
import requests
# Making a PUT request
r = requests.put('https://httpbin.org/put', data={'key':'value'})
#check status code for response received
# success code - 200
print(r)
# print content of request
print(r.content)
HTTP POST Request
HTTP POST is a request method supported by HTTP used by the World Wide Web. By design, the POST request method requests that a web server accepts the data enclosed in the body of the request message, most likely for storing it.
Python
import requests
# Making a POST request
r = requests.post('https://httpbin.org/post', data={'key':'value'})
#check status code for response received
# success code - 200
print(r)
# print content of request
print(r.json())
HTTP status codes are a set of standardized three-digit numbers used by web servers to communicate the outcome of an HTTP request made by a client. They provide information about whether the request was successful, encountered an error, or requires further action by the client.
- 1xx – Informational: Request received, continuing process.
- 2xx – Success: The action was successfully received, understood, and accepted.
- 3xx – Redirection: Further action needs to be taken in order to complete the request.
- 4xx – Client Error: The request contains bad syntax or cannot be fulfilled.
- 5xx – Server Error: The server failed to fulfill an apparently valid request.
Each status code has a specific meaning, allowing both clients and servers to understand the outcome of a request and take appropriate action. For example, status code 200 indicates a successful request, while status code 404 indicates that the requested resource was not found on the server.
There are three types of security mechanism for an API –
- HTTP Basic Authentication: In this mechanism HTTP User Agent provides a Username and Password. Since this method depends only on HTTP Header and entire authentication data is transmitted on insecure lines
- API Keys: API Keys came into picture due to slow speed and highly vulnerable nature of HTTP Basic Authentication.
- OAuth: OAuth is not only a method of Authentication or Authorization, but it’s also a mixture of both the methods. Whenever an API is called using OAuth credential, user logs into the system, generating a token.
The Cache-Control header is a general header, that specifies the caching policies of server responses as well as client requests. Basically, it gives information about the manner in which a particular resource is cached, location of the cached resource, and its maximum age attained before getting expired i.e. time to live.
Syntax:
Cache-Control: <directive> [, <directive>]*
The process of connecting two or more software applications or processes using APIs is referred to as API integration. This allows the systems to exchange data and functionality, allowing them to work in unison. APIs are a collection of protocols, routines, and tools used to create software and applications.
Share your thoughts in the comments
Please Login to comment...