Explain the use of req and res objects in Express JS
Last Updated :
17 Dec, 2023
Express JS is used to build RESTful APIs with Node.js. We have a ‘req‘ (request) object in Express JS which is used to represent the incoming HTTP request that consists of data like parameters, query strings, and also the request body. Along with this, we have ‘res‘ (response) which is used to send the HTTP response to the client which allows the modification of headers and consists of status codes, and resources.
Prerequisites
How are req and res Objects Useful?
Both the objects ‘req‘ and ‘res‘ are responsible for handling the HTTP requests and responses in the web applications. Below are some of the points which describe the benefits of these objects:
1. req (Request) Object:
- Accessing the Request Data: By using the req object, we can access the various components of the incoming HTTP request which consists of data, headers, parameters, etc.
- Middleware Interaction: Using the req object in Express.js, middleware functions can change and modify the request object by allowing various tasks of logging and authentication.
- Routing: By implementing the dynamic routes in the application, the req object can be used as it captures the URL parameters and also allows it to respond dynamically based on the client’s input.
2. res (Response) Object:
- Send Responses: The res object is used to send the HTP responses to the client which includes the resource, data, status codes, and headers.
- Error Handling: Using the res object we can send the error responses which is important for handling the errors and providing feedback to the client.
- Content Modification: We can set the custom response headers, status codes, and content through which the response control can be managed by us.
Steps to use req and res objects in Express
Step 1: In the first step, we will create the new folder as an http-objects by using the below command in the VScode terminal.
mkdir http-objects
cd http-objects
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
Step 4: Now create the below Project Structure of our project which includes the file as app.js.
Project Structure:
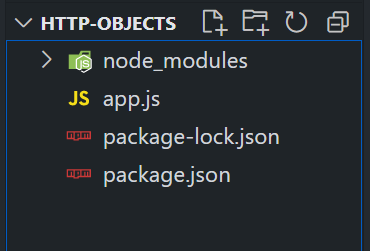
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Example: Write the following code in app.js file
Javascript
const express = require( "express" );
const app = express();
const port = 3000;
app.use((req, res, next) => {
console.log(`Incoming Request: ${req.method} ${req.url}`);
next();
});
app.get( "/favicon.ico" , (req, res) => {
res.status(204).end();
});
app.get( "/greet/:name" , (req, res) => {
const geekName = req.params.name;
const htmlResponse = `
<!DOCTYPE html>
<html lang= "en" >
<head>
<meta charset= "UTF-8" >
<meta name= "viewport" content= "width=device-width,
initial-scale=1.0" >
<title>Greeting Page</title>
</head>
<body>
<h1>Hello, ${geekName}!</h1>
</body>
</html>
`;
console.log(`Outgoing Response: ${res.statusCode} ${res.statusMessage}`);
res.send(htmlResponse);
});
app.listen(port, () => {
console.log(`Server is running at http:
});
|
Start the server by using the below command:
node app.js
Output:
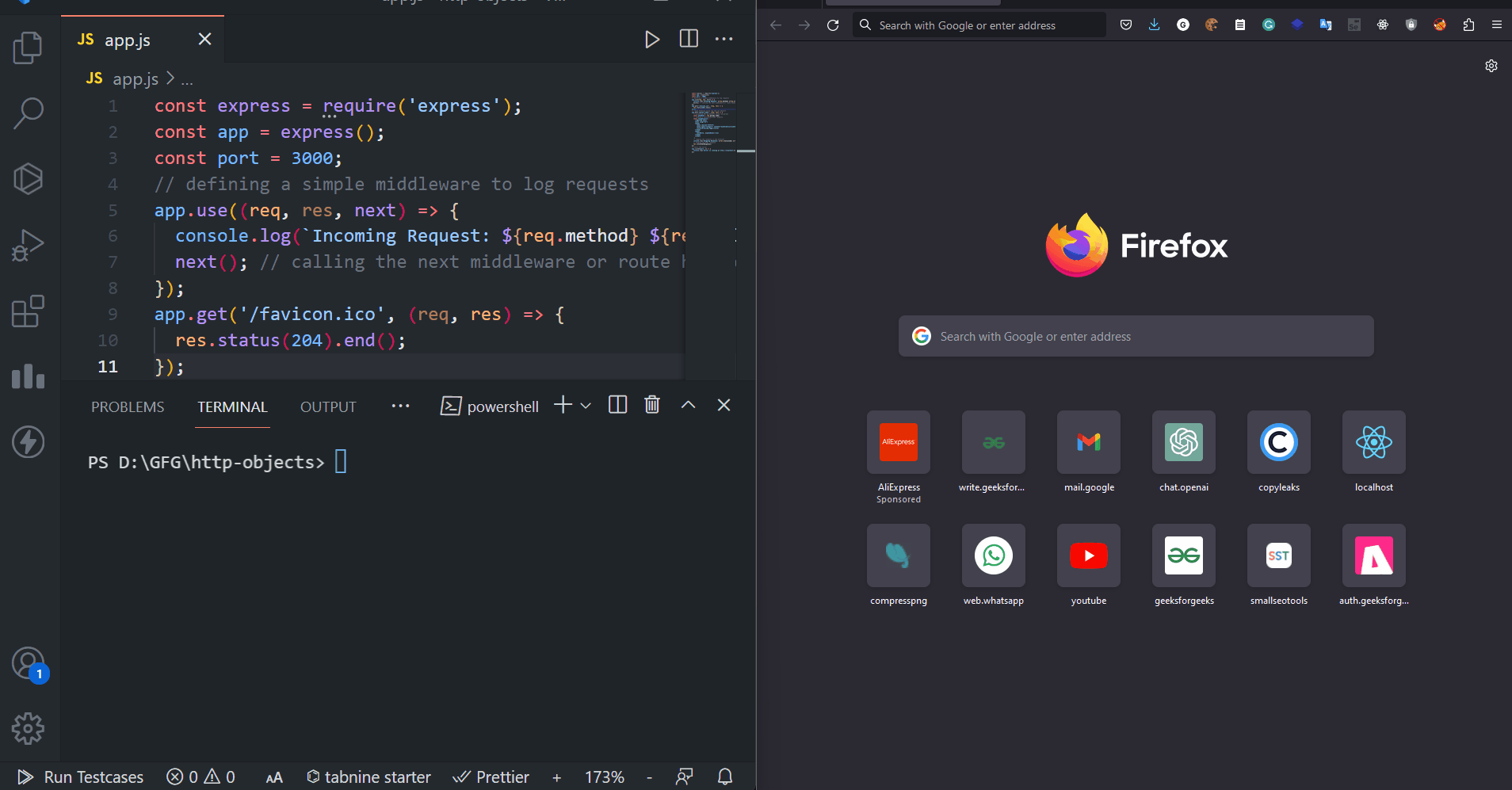
Share your thoughts in the comments
Please Login to comment...