How to Enhance Node JS Performance through Clustering?
Last Updated :
30 Nov, 2023
In this article, we are going to learn about clustering through Node.js. Clustering is a process through which we can use multiple cores of a computer simultaneously. Like JavaScript, which is a single-threaded language, the code of JavaScript will run only on a single core if you have more than one core installed on your machine. So clustering is the process through which we can enhance the performance of our software using multiple cores of the machine at the same time.
Understand Clustering
Clustering is the process through which we can use multiple cores of our central processing unit at the same time with the help of Node JS, which helps to increase the performance of the software and also reduces its time load. Cluster is the module of JavaScript required to enable clustering in your program. First, we need to install this module in the required project. We can install cluster modules through the given command.
npm i cluster
Benefits of Clustering
Clustering provides the feature of improving system efficiency and enhancing system performance. Some of the main benefits of clustering are as follows:
- Clustering provides parallel processing, which means that more than one core can work together on a single source code. One part of the code will run on the master core, and the other part will be executed on the other cores present in the cluster.
- Clustering provides load balancing to machines and software, which means the load that should be on a single core will be divided into multiple cores, so the whole load will not be on a single core; it will be divided into multiple cores.
- Clustering provides resource utilization; it will help to utilize the other cores of the processor that are available to use, which leads to proper resource utilization.
- Clustering provides you with energy efficiency because the cores that are free or not working are being utilized, and so here we are saving energy.
Example Without Clustering
Example : Write the following code in App.js file
Javascript
const http = require( 'http' );
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end( 'Hello Geeks\n' );
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server Established at -> ${PORT}`);
});
|
Output:
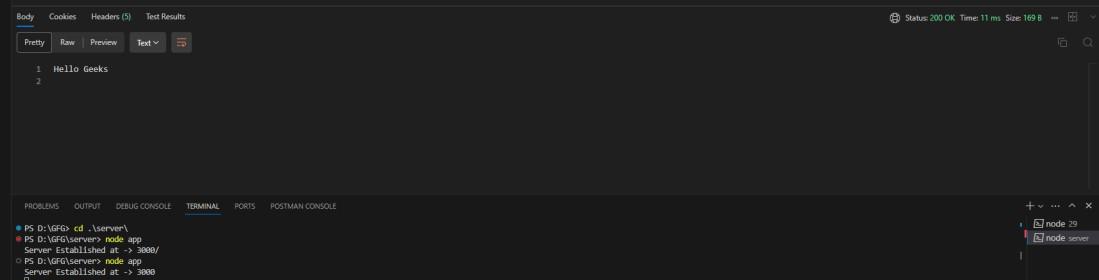
Example with Clustering
Example : Write the following code in App.js file
Javascript
const cluster = require( 'cluster' );
const http = require( 'http' );
const numCore = require( 'os' ).cpus().length;
if (cluster.isMaster) {
for (let i = 0; i < numCore; i++) {
cluster.fork();
}
cluster.on( 'exit' , (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died. Restarting...`);
cluster.fork();
});
} else {
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end( 'Hello Geeks\n' );
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Worker ${process.pid} established on PORT -> ${PORT}/`);
});
}
|
Output:
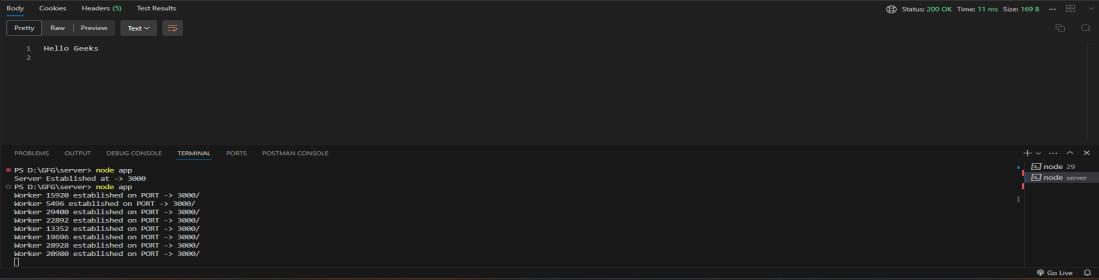
Provides utilization of multiple cores, which enhances performance.
|
Available cores are rest-free, so they will reduce performance.
|
Provides load balancing, which divides load among each core.
|
Does not provide load balancing, so it burdens only one core.
|
Resource utilization helps to work all core that reduce time to process.
|
Resource utilization does not exist so it will take much time to execute process.
|
It will take less energy because no core will be free.
|
It will take more energy because cores will be available in rest.
|
Conclusion
Node JS clustering improves multiple factors of applications, like allowing developers to use more cores at the same time, which will improve the efficiency of the program, reduce the time taken for execution, reduce the energy consumption of the program, and also balance the load among each processor, which helps to fix the resource utilization of the machine.
Share your thoughts in the comments
Please Login to comment...