AKTU 1st Year Sem 2 Solved Paper 2014-15 | COMP. SYSTEM & C PROGRAMMING | Sec B
Last Updated :
28 Jun, 2021
Paper download link: Paper | Sem 2 | 2014-15
B.Tech.
(SEM-II) THEORY EXAMINATION 2014-15
COMPUTER SYSTEM & PROGRAMMING IN C
Time: 3hrs
Total Marks: 100
Note:-
- Attempt all questions. Marks are indicated against each question.
- Assume suitable data wherever necessary.
2. Attempt any TWO parts: (10*2 = 20)
- What do you understand by ASCII value of a character? Can we use expressions including both integer data type and character data type? Justify your answer.
ASCII is American Standard Code for Information Interchange. ASCII is a character-encoding scheme and it was the first character encoding standard.ASCII uses 7 bits to represent a character. It has 128 code points, 0 through 127. It is a code for representing English characters as numbers, with each letter assigned a number from 0 to 127.
Yes, we can use expressions including both integer data type and character data type. In such expressions, type conversions occur and the character datatype gets converted into its integer value. This integer value is the ASCII value of the character.
Example:
#include <stdio.h>
int main()
{
int x = 10;
char y = 'a' ;
int z;
z = x + y;
printf ( "z = %d" , z);
return 0;
}
|
Output:
z = 107
- Write the difference between type conversion and type casting. What are the escape sequences characters?
In C programming language, there are 256 numbers of characters in character set. The entire character set is divided into 2 parts i.e. the ASCII characters set and the extended ASCII characters set. But apart from that, some other characters are also there which are not the part of any characters set, known as ESCAPE characters.
List of Escape Sequences
\a Alarm or Beep
\b Backspace
\f Form Feed
\n New Line
\r Carriage Return
\t Tab (Horizontal)
\v Vertical Tab
\\ Backslash
' Single Quote
\" Double Quote
\? Question Mark
\ooo octal number
\xhh hexadecimal number
\0 Null
Example:
#include <stdio.h>
int main( void )
{
printf ( "Hello Geeks\b\b\b\bF" );
return (0);
}
|
Output:
The output is dependent upon compiler.
- Convert the following number into:
(i) (11010.0110)2 = ()10
(11010.0110)2
= (26.375)10
(ii) (110101011.0110110)2 = ()8
(110101011.0110110)2
= (653.33)8
(iii) (2B6D)16 = ()2
(2B6D)16
= (10101101101101)2
(iv) (AB4F.C1)16 = ()10
(AB4F.C1)16
= (43855.75390625)10
(v) (54)6 = ()4
(54)6
= (202)4
3. Attempt any TWO parts: (10*2 = 20)
- Give the loop statement to print the following sequence of integers:
-6 -4 -2 0 2 4 6
#include <stdio.h>
int main()
{
int i = 0;
for (i = -6; i <= 6; i += 2)
printf ( "%d " , i);
return 0;
}
|
Output:
-6 -4 -2 0 2 4 6
- What are the main principles of recursion?
The process in which a function calls itself directly or indirectly is called recursion and the corresponding function is called as recursive function. Using recursive algorithm, certain problems can be solved quite easily. Examples of such problems are Towers of Hanoi (TOH), Inorder/Preorder/Postorder Tree Traversals, DFS of Graph, etc.
What is base condition in recursion?
In recursive program, the solution to base case is provided and solution of bigger problem is expressed in terms of smaller problems.
int fact(int n)
{
if (n < = 1) // base case
return 1;
else
return n*fact(n-1);
}
In the above example, base case for n < = 1 is defined and larger value of number can be solved by converting to smaller one till base case is reached.
- If int a=2, b=3, x=0; Find the value of x=(++a, b+=a)
Output: x = 6
Explanation:
- As a = 2, b = 3 and x = 0 intitially.
- Therefore,
x = (++a, b+=a)
= ((++a), b+=a)
= (b+=a)…..Now a = 3
= (b = b+a)
= (b = 3+3)
= (b = 6)
= (6)
x = 6
4. Attempt any TWO parts: (10*2 = 20)
- What are the different types of operators in C language and also write down the difference between the associativity and precedence of operators.
Operators are the foundation of any programming language. Thus the functionality of C/C++ programming language is incomplete without the use of operators. We can define operators as symbols that helps us to perform specific mathematical and logical computations on operands. In other words we can say that an operator operates the operands.
For example, consider the below statement:
c = a + b;
Here, ‘+’ is the operator known as addition operator and ‘a’ and ‘b’ are operands. The addition operator tells the compiler to add both of the operands ‘a’ and ‘b’. C/C++ has many built-in operator types and they can be classified as:
- Arithmetic Operators: These are the operators used to perform arithmetic/mathematical operations on operands. Examples: (+, -, *, /, %, ++, –).
- Relational Operators: Relational operators are used for comparison of the values of two operands. For example: checking if one operand is equal to the other operand or not, an operand is greater than the other operand or not etc. Some of the relational operators are (==, >=, <= ). To learn about each of these operators in details go to this link.
- Logical Operators: Â Logical Operators are used to combine two or more conditions/constraints or to complement the evaluation of the original condition in consideration. The result of the operation of a logical operator is a boolean value either true or false. To learn about different logical operators in details please visit this link.
- Bitwise Operators: The Bitwise operators is used to perform bit-level operations on the operands. The operators are first converted to bit-level and then calculation is performed on the operands. The mathematical operations such as addition, subtraction, multiplication etc. can be performed at bit-level for faster processing. To learn bitwise operators in details, visit this link.
- Assignment Operators: Assignment operators are used to assign value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of variable on the left side otherwise the compiler will raise an error.
- Other Operators: Apart from the above operators there are some other operators available in C or C++ used to perform some specific task. Some of them are discussed here:
- sizeof operator: sizeof is a much used in the C/C++ programming language. It is a compile time unary operator which can be used to compute the size of its operand. The result of sizeof is of unsigned integral type which is usually denoted by size_t. Basically, sizeof operator is used to compute the size of the variable. To learn about sizeof operator in details you may visit this link.
- Comma Operator: The comma operator (represented by the token, ) is a binary operator that evaluates its first operand and discards the result, it then evaluates the second operand and returns this value (and type). The comma operator has the lowest precedence of any C operator. Comma acts as both operator and separator. To learn about comma in details visit this link.
- Conditional Operator: Conditional operator is of the form Expression1 ? Expression2 : Expression3 . Here, Expression1 is the condition to be evaluated. If the condition(Expression1) is True then we will execute and return the result of Expression2 otherwise if the condition(Expression1) is false then we will execute and return the result of Expression3. We may replace the use of if..else statements by conditional operators. To learn about conditional operators in details, visit this link.
-
- Describe call by value and call by reference with example.
There are different ways in which parameter data can be passed into and out of methods and functions. Let us assume that a function B() is called from another function A(). In this case A is called the “caller function” and B is called the “called function or callee function”. Also, the arguments which A sends to B are called actual arguments and the parameters of B are called formal arguments.
Important methods of Parameter Passing
- Pass By Value : This method uses in-mode semantics. Changes made to formal parameter do not get transmitted back to the caller. Any modifications to the formal parameter variable inside the called function or method affect only the separate storage location and will not be reflected in the actual parameter in the calling environment. This method is also called as call by value.
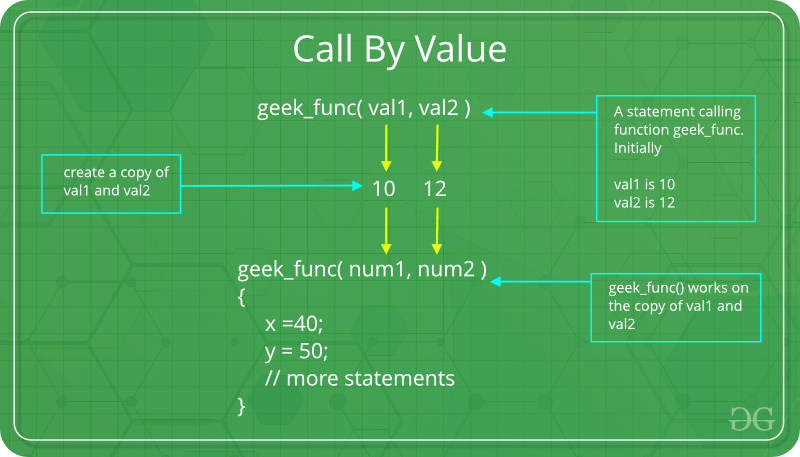
#include <stdio.h>
void func( int a, int b)
{
a += b;
printf ( "In func, a = %d b = %d\n" , a, b);
}
int main( void )
{
int x = 5, y = 7;
func(x, y);
printf ( "In main, x = %d y = %d\n" , x, y);
return 0;
}
|
Output:
In func, a = 12 b = 7
In main, x = 5 y = 7
Languages like C, C++, Java support this type of parameter passing. Java in fact is strictly call by value.
Shortcomings:
- Inefficiency in storage allocation
- For objects and arrays, the copy semantics are costly
- Pass by reference(aliasing) : This technique uses in/out-mode semantics. Changes made to formal parameter do get transmitted back to the caller through parameter passing. Any changes to the formal parameter are reflected in the actual parameter in the calling environment as formal parameter receives a reference (or pointer) to the actual data. This method is also called as <em>call by reference. This method is efficient in both time and space.
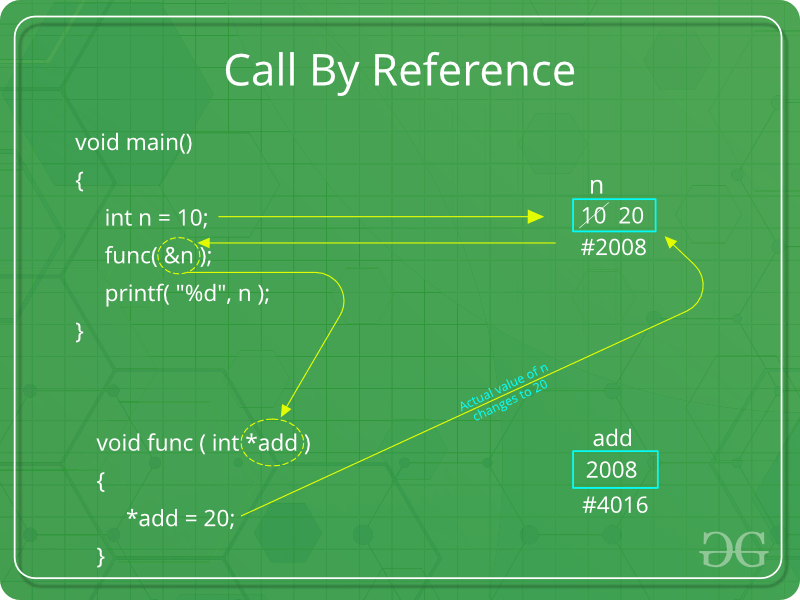
#include <stdio.h>
void swapnum( int * i, int * j)
{
int temp = *i;
*i = *j;
*j = temp;
}
int main( void )
{
int a = 10, b = 20;
swapnum(&a, &b);
printf ( "a is %d and b is %d\n" , a, b);
return 0;
}
|
Output:
a is 20 and b is 10
5. Attempt any TWO parts: (10*2 = 20)
- Write a program in C language to generate the Fibonacci series.
Program in C to generate the Fibonacci series:
#include <stdio.h>
int fib( int n)
{
if (n <= 1)
return n;
return fib(n - 1) + fib(n - 2);
}
int main()
{
int n = 9;
printf ( "%d" , fib(n));
getchar ();
return 0;
}
|
- What do you mean by dynamic memory allocation? Explain the following functions in detail: free and calloc
Dynamic Memory Allocation in C: It can be defined as a procedure in which the size of a data structure (like Array) is changed during the runtime.
C provides some functions to achieve these tasks. There are 4 library functions provided by C defined under <stdlib.h> header file to facilitate dynamic memory allocation in C programming. They are:
- malloc()
- calloc()
- free()
- realloc()
Lets see each of them in detail.
-
calloc()
“calloc” or “contiguous allocation” method is used to dynamically allocate the specified number of blocks of memory of the specified type. It initializes each block with a default value ‘0’.
Syntax:
ptr = (cast-type*)calloc(n, element-size);
For Example:
ptr = (float*) calloc(25, sizeof(float));
This statement allocates contiguous space in memory
for 25 elements each with the size of float.
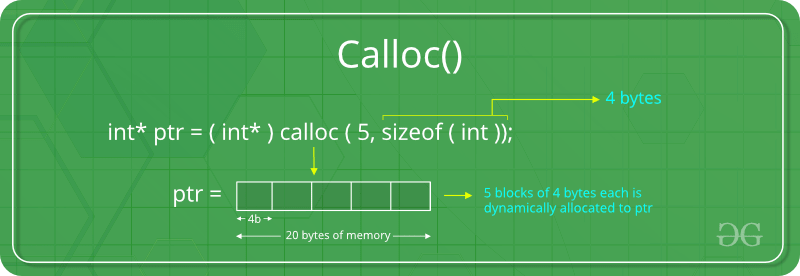
If the space is insufficient, allocation fails and returns a NULL pointer.
Example:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int * ptr;
int n, i, sum = 0;
n = 5;
printf ( "Enter number of elements: %d\n" , n);
ptr = ( int *) calloc (n, sizeof ( int ));
if (ptr == NULL) {
printf ( "Memory not allocated.\n" );
exit (0);
}
else {
printf ( "Memory successfully allocated using calloc.\n" );
for (i = 0; i < n; ++i) {
ptr[i] = i + 1;
}
printf ( "The elements of the array are: " );
for (i = 0; i < n; ++i) {
printf ( "%d, " , ptr[i]);
}
}
return 0;
}
|
Output:
Enter number of elements: 5
Memory successfully allocated using calloc.
The elements of the array are: 1, 2, 3, 4, 5,
-
free()
“free” method is used to dynamically de-allocate the memory. The memory allocated using functions malloc() and calloc() are not de-allocated on their own. Hence the free() method is used, whenever the dynamic memory allocation takes place. It helps to reduce wastage of memory by freeing it.
Syntax:
free(ptr);
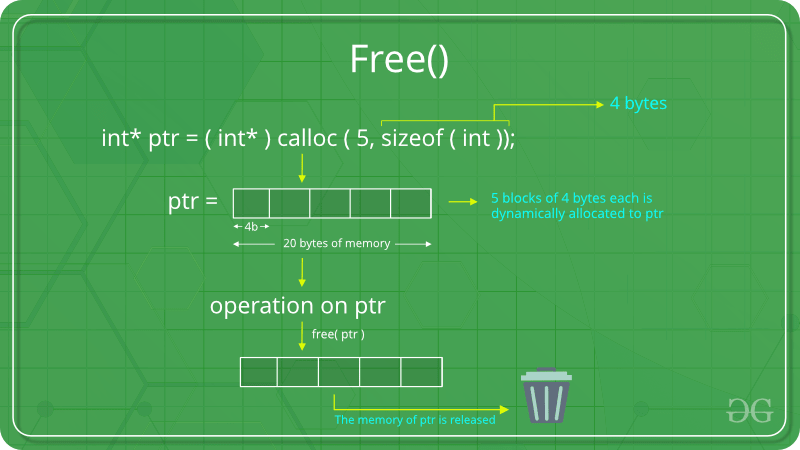
Example:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int *ptr, *ptr1;
int n, i, sum = 0;
n = 5;
printf ( "Enter number of elements: %d\n" , n);
ptr = ( int *) malloc (n * sizeof ( int ));
ptr1 = ( int *) calloc (n, sizeof ( int ));
if (ptr == NULL || ptr1 == NULL) {
printf ( "Memory not allocated.\n" );
exit (0);
}
else {
printf ( "Memory successfully allocated using malloc.\n" );
free (ptr);
printf ( "Malloc Memory successfully freed.\n" );
printf ( "\nMemory successfully allocated using calloc.\n" );
free (ptr1);
printf ( "Calloc Memory successfully freed.\n" );
}
return 0;
}
|
Output:
Enter number of elements: 5
Memory successfully allocated using malloc.
Malloc Memory successfully freed.
Memory successfully allocated using calloc.
Calloc Memory successfully freed.
- Write a program to add two matrices of dimension 3*3 and store the result in another matrix
#include <stdio.h>
#define N 3
void add( int A[][N], int B[][N], int C[][N])
{
int i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
C[i][j] = A[i][j] + B[i][j];
}
int main()
{
int A[N][N] = { { 1, 1, 1 },
{ 2, 2, 2 },
{ 3, 3, 3 } };
int B[N][N] = { { 1, 1, 1 },
{ 2, 2, 2 },
{ 3, 3, 3 } };
int C[N][N];
int i, j;
add(A, B, C);
printf ( "Result matrix is \n" );
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
printf ( "%d " , C[i][j]);
printf ( "\n" );
}
return 0;
}
|
Output:
Result matrix is
2 2 2
4 4 4
6 6 6
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...