While loop Syntax
Last Updated :
14 Feb, 2024
While loop is a fundamental control flow structure (or loop statement) in programming, enabling the execution of a block of code repeatedly as long as a specified condition remains true. Unlike the for loop, which is tailored for iterating a fixed number of times, the while loop excels in scenarios where the number of iterations is uncertain or dependent on dynamic conditions.
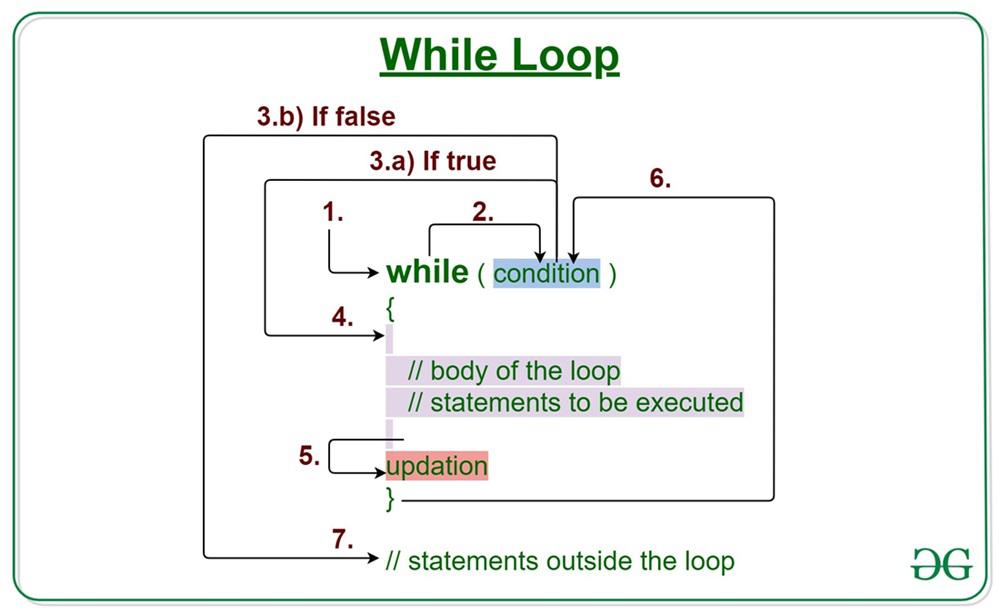
The syntax of While loop varies slightly depending on the programming language, but the basic structure is similar across many languages.
while (condition) {
// Code block to be executed repeatedly as long as the condition is true
}
Here’s a general overview of the Syntax of While loop:
- Condition: This is the condition that is evaluated before each iteration of the loop. If the condition is true, the loop body is executed; otherwise, the loop terminates.
- Loop Body: This is the block of code that gets executed if the condition is true.
- Update: Inside the loop body, there should be some logic to change the variables involved in the condition so that the loop eventually terminates. Otherwise, you risk creating an infinite loop.
While loop Syntax in C/C++:
Syntax:
while (condition) {
// Code block to be executed repeatedly as long as the condition is true
}
Explanation of the Syntax:
- In C and C++, the
while
loop executes a block of code repeatedly as long as the specified condition evaluates to true.
- The loop first evaluates the condition specified within the parentheses
()
.
- If the condition is true, the code block within the curly braces
{}
is executed.
- After executing the code block, the loop returns to the beginning and re-evaluates the condition.
- If the condition remains true, the process continues; otherwise, the loop terminates.
Implementation of While loop Syntax in C/C++:
C++
#include <iostream>
using namespace std;
int main()
{
int i = 0;
while (i < 10) {
cout << i << " " ;
i++;
}
return 0;
}
|
C
#include <stdio.h>
int main()
{
int i = 0;
while (i < 10) {
printf ( "%d " , i);
i++;
}
return 0;
}
|
Output
0 1 2 3 4 5 6 7 8 9
Java While loop Syntax:
Syntax:
while (condition) {
// Code block to be executed repeatedly as long as the condition is true
}
Explanation of the Syntax:
- In Java, the
while
loop operates similarly to C and C++.
- It first evaluates the condition specified within the parentheses
()
.
- If the condition is true, the code block within the curly braces
{}
is executed.
- After executing the code block, the loop returns to the beginning and re-evaluates the condition.
- If the condition remains true, the process continues; otherwise, the loop terminates.
Implementation of While loop Syntax in Java:
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int i = 0 ;
while (i < 10 ) {
System.out.print(i + " " );
i++;
}
}
}
|
Output
0 1 2 3 4 5 6 7 8 9
While loop Syntax in Python:
Syntax:
while condition:
# Code block to be executed repeatedly as long as the condition is true
Explanation of the Syntax:
- Python’s
while
loop follows a similar structure to other languages.
- It begins by evaluating the condition specified after the
while
keyword.
- If the condition is true, the code block indented below is executed.
- After executing the code block, the loop returns to the
while
statement and re-evaluates the condition.
- If the condition remains true, the process continues; otherwise, the loop ends.
Implementation of While loop Syntax in Python:
Python3
i = 0
while i < 10 :
print (i, end = " " )
i + = 1
|
Output
0 1 2 3 4 5 6 7 8 9
While loop Syntax in C#:
Syntax:
while (condition) {
// Code block to be executed repeatedly as long as the condition is true
}
Explanation of the Syntax:
- In C#, the
while
loop behaves similarly to C, C++, and Java.
- It starts by evaluating the condition specified within the parentheses
()
.
- If the condition is true, the code block within the curly braces
{}
is executed.
- After executing the code block, the loop returns to the beginning and re-evaluates the condition.
- If the condition remains true, the process continues; otherwise, the loop terminates.
Implementation of While loop Syntax in C#:
C#
using System;
public class GFG {
static public void Main()
{
int i = 0;
while (i < 10) {
Console.Write(i + " " );
i++;
}
}
}
|
Output
0 1 2 3 4 5 6 7 8 9
While loop Syntax in JavaScript:
Syntax:
while (condition) {
// Code block to be executed repeatedly as long as the condition is true
}
Explanation of the Syntax:
- JavaScript’s
while
loop follows the same pattern as other languages.
- It evaluates the condition specified within the parentheses
()
before each iteration.
- If the condition is true, the code block within the curly braces
{}
is executed.
- After executing the code block, the loop returns to the beginning and re-evaluates the condition.
- If the condition remains true, the loop continues; otherwise, it ends.
Implementation of While loop Syntax in JavaScript:
Javascript
let i = 0;
while (i < 10) {
console.log(i + " " );
i++;
}
|
Output
0
1
2
3
4
5
6
7
8
9
Share your thoughts in the comments
Please Login to comment...