What is a Syntax Error and How to Solve it?
Last Updated :
12 Apr, 2024
Syntax error is an error in the syntax of a sequence of characters that is intended to be written in a particular programming language. It’s like a grammatical error in a programming language. These errors occur when the code does not conform to the rules and grammar of the language. Syntax errors are detected at compile-time in compiled languages, and during program execution in interpreted languages.
What is Syntax Error?
Syntax Error is defined as a fundamental mistake created in programming when the code doesn’t follow the syntax rules of the programming language.
Language syntax of programming languages can be defined as a set of rules and structures that determine how the code should be written to be correctly translated and executed by the compilers or interpreters.
Types of Syntax Error:
- Missing Parentheses or Brackets: Forgetting to include closing parentheses
)
, square brackets ]
, or curly braces {}
can lead to syntax errors, especially in expressions, function calls, or data structures. - Missing Semicolons: In languages that use semicolons to terminate statements (e.g., C, Java, JavaScript), omitting a semicolon at the end of a statement can result in a syntax error.
- Mismatched Quotes: Forgetting to close quotation marks
'
or "
around strings can lead to syntax errors, as the interpreter/compiler will interpret everything until the next matching quote as part of the string. - Incorrect Indentation: In languages like Python, incorrect indentation can cause syntax errors, especially within control structures like loops, conditional statements, or function definitions.
- Misspelled Keywords or Identifiers: Misspelling keywords, variable names, function names, or other identifiers can result in syntax errors. The interpreter/compiler won’t recognize these misspelled names, leading to errors.
Common Syntax Errors:
- Violation of Language Rules: Syntax errors take place when a programmer writes code that violates the syntax rules of the computer language that is established. These rules dictate the proper use of parentheses, brackets, semicolons, quotation marks, and other punctuation marks and the structure and organization of the expressions and statements.
- Compiler or Interpreter Detection: When you try to compile or execute code having syntax errors, the compiler or interpreter goes through the code and lists down any breaches of the rules of the language’s syntax. Then, it produces an error message which pinpoints the exact place and nature of the errors.
- Prevents Execution: Unlike runtime errors that happen while the program runs, syntax errors do not allow the program to run at all. This is because the compiler or interpreter cannot interpret the instructions given in the code because of their faulty structure or grammar.
- Common Causes: There are syntax errors that come about due to various mistakes made by the programmer including misspelled keywords, missing or misplaced punctuation, incorrect indentation, mismatching of parentheses or brackets, and typographical errors. These mistakes are simple errors, but sometimes they can produce prominent effects when they are not corrected.
- Error Messages: When a grammar mistake is caught, the compiler or interpreter usually signals it by generating an error message that tells about the nature of the mistake and suggests fixing it like where it is located in the code and sometimes how it should be changed. Making out the information and interpreting the error message is the key to successful code debugging.
How to Identify Syntax Errors?
Normally compiler or interpreter detects syntax errors during program execution. The error message not only shows the place where the error happened but also provides some clues about how the problem originated.
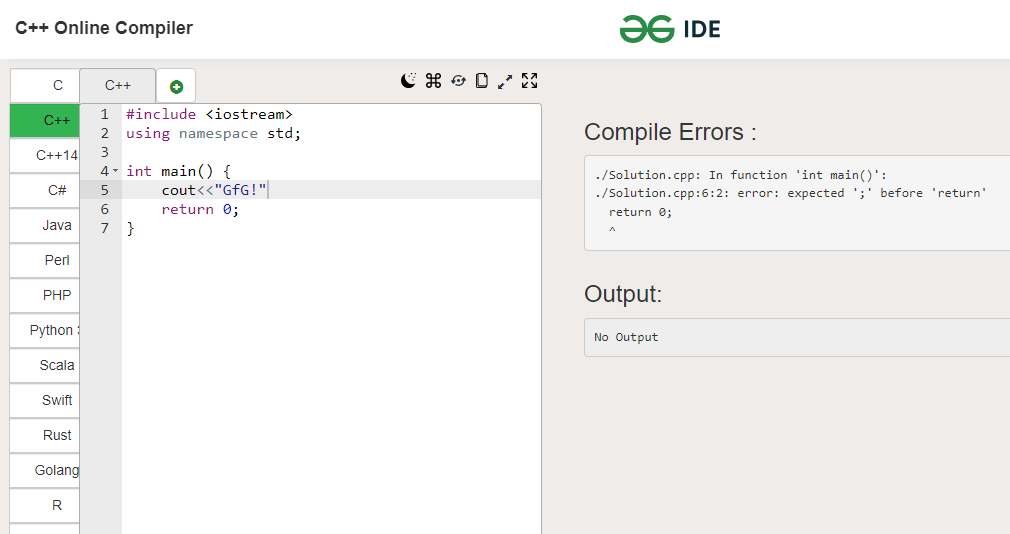
Syntax Error in C++:
Here is the example of syntax error in C++:
C++
#include <iostream>
using namespace std;
int main()
{
int x = 10
cout << "The value of x is: " << x << endl;
}
Syntax Error in Java:
Here is the example of syntax error in Java:
Java
public class SyntaxErrorExample {
public static void main(String[] args) {
int x = 10
System.out.println("The value of x is: " + x);
}
}
Syntax Error in Python:
Here is the example of syntax error in Python:
Python3
def syntax_error_example():
if x == 5
print("x is equal to 5")
Syntax Error in Javascript:
Here is the example of syntax error in Javascript:
JavaScript
How to Solve Syntax Errors?
Fixing syntax errors is all about manually going through the code and correctly finding and fixing the problems. Here are some steps to help you tackle syntax errors effectively:
- Review the Error Message: The first step is to pay a lot of attention to the error message displayed by the compiler or interpreter. Sometimes it provides the necessary clue to the position and structure of the grammar error.
- Check the Code Surrounding the Error: Study the code lines that are around the error point. The problem may conceivably be not where the error is marked but in another section of the code.
- Look for Common Mistakes: Syntax errors may arise from common mistakes like leaving punctuation out, not matching closing and opening brackets or parentheses, and typing the wrong word. Gaze over these elements of your code.
- Use Debugging Tools: The majority of integrated development environments (IDEs) and editors provide debugging tools to identify and fix syntax errors swiftly. Using these tools will help us speed up the debugging process.
- Consult Documentation and Resources: If you cannot remember the correct syntax for a particular construct, the official documentation or other reputable sources could be consulted to get guidance. The main principle here is to know about the language syntax rules to avoid syntax errors in the future.
Tips to Avoid Syntax Errors:
- Consistent Indentation: Maintain consistent indentation throughout your code to improve readability and prevent syntax errors, especially in languages that rely on indentation for structure like Python.
- Use Code Editors with Syntax Highlighting: Utilize code editors with syntax highlighting features to visually identify syntax errors as you write code.
- Follow Language Syntax Rules: Familiarize yourself with the syntax rules of the programming language you’re using and adhere to them strictly.
- Test Code Frequently: Test your code frequently as you write it to catch syntax errors early and address them promptly.
- Break Code into Smaller Parts: Break down complex code into smaller, manageable parts to make it easier to identify and fix syntax errors.
Real world Syntax Error Problems:
- Configuration Files: Syntax errors can occur in configuration files (e.g., XML, JSON, YAML) used by applications. For instance, a missing closing tag in an XML file or a misplaced comma in a JSON file can lead to syntax errors.
- Markup Languages: In markup languages like HTML or Markdown, syntax errors can occur due to missing or mismatched tags. For example, forgetting to close a
<div>
tag or using incorrect indentation in Markdown can result in syntax errors. - SQL Queries: Syntax errors are common in SQL queries, especially when writing complex statements. Errors can occur due to missing commas, incorrect table aliases, or improper placement of keywords like SELECT, FROM, WHERE, etc.
- Regular Expressions: Writing regular expressions with incorrect syntax can lead to errors. Common mistakes include missing escape characters, mismatched parentheses, or invalid quantifiers.
- Command Line Syntax: Incorrect usage of command-line tools and utilities can result in syntax errors. For example, providing the wrong option or argument format when executing commands can lead to errors.
Conclusion:
In conclusion, a syntax error is a mistake in the code’s structure that makes it impossible for the program to be compiled or run. A syntax error is like a grammatical mistake in programming languages. It occurs when code violates the rules of the language’s syntax, making it impossible for the program to run. These errors are usually easy to spot and fix because they’re caught by the compiler or interpreter during the compilation or interpretation process.
Share your thoughts in the comments
Please Login to comment...