Do While loop Syntax
Last Updated :
14 Feb, 2024
Do while loop is a control flow statement found in many programming languages. It is similar to the while loop, but with one key difference: the condition is evaluated after the execution of the loop’s body, ensuring that the loop’s body is executed at least once.
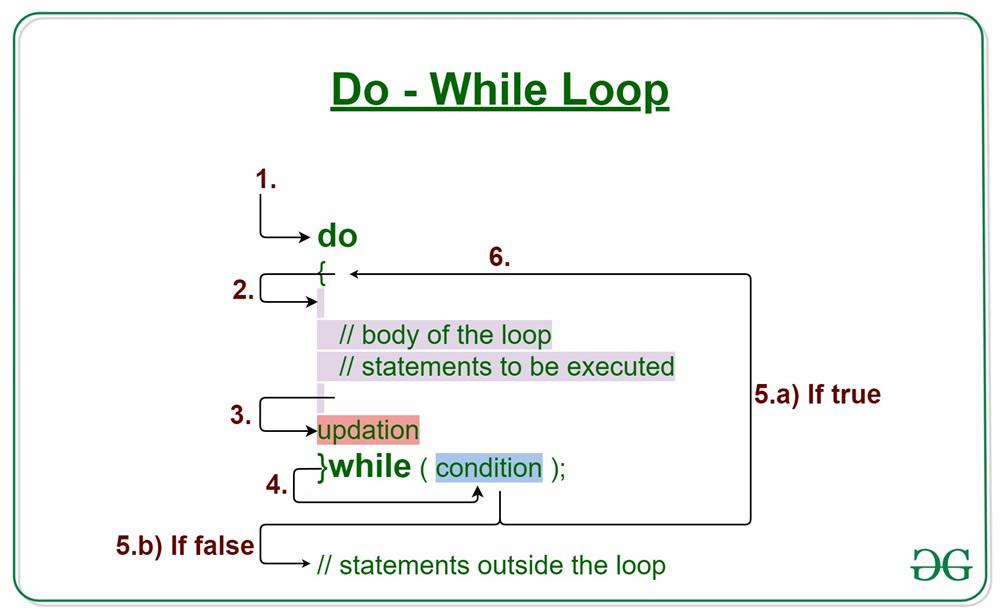
The syntax for a do while loop varies slightly depending on the programming language you’re using, but the general structure is similar across many languages.
do {
// Code block to be executed at least once
// This block will execute at least once
} while (condition);
Here’s a basic outline:
- Initialization: Initialize any loop control variables or other necessary variables before entering the loop.
- Do While Loop Structure: The loop consists of two main parts: the “do” block and the “while” condition.
- The “do” block contains the code that will be executed at least once, regardless of the condition.
- The “while” condition specifies the condition that must be true for the loop to continue iterating. If the condition evaluates to true, the loop will execute again. If it evaluates to false, the loop will terminate.
- Loop Execution: The loop executes the code inside the “do” block, then evaluates the “while” condition.
- If the condition is true, the loop repeats, executing the code block again.
- If the condition is false, the loop terminates, and control passes to the code following the loop.
Do While loop Syntax in C/C++:
Syntax:
do {
// Code block to be executed at least once
} while (condition);
Explanation of the Syntax:
- In C and C++, the do while loop executes a block of code once before checking the condition.
- The code block enclosed within the curly braces
{}
is executed at least once, regardless of the condition.
- After executing the code block, the loop checks the specified condition inside the parentheses
()
.
- If the condition evaluates to true, the loop repeats; otherwise, it terminates.
Implementation of Do While Loop Syntax in C/C++:
C++
#include <iostream>
using namespace std;
int main()
{
int i = 0;
do {
cout << i << " " ;
i++;
} while (i < 10);
return 0;
}
|
C
#include <stdio.h>
int main()
{
int i = 0;
do {
printf ( "%d " , i);
i++;
} while (i < 10);
return 0;
}
|
Output
0 1 2 3 4 5 6 7 8 9
Java Do While loop Syntax:
Syntax:
do {
// Code block to be executed at least once
} while (condition);
Explanation of the Syntax:
- In Java, the do while loop works similarly to C and C++.
- The code block within the curly braces
{}
is executed at least once.
- After executing the code block, the loop evaluates the condition specified in the parentheses
()
.
- If the condition is true, the loop repeats; otherwise, it exits.
Implementation of Do While Loop Syntax in Java:
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int i = 0 ;
do {
System.out.print(i + " " );
i++;
} while (i < 10 );
}
}
|
Output
0 1 2 3 4 5 6 7 8 9
Do While loop Syntax in Python:
Python doesn’t have a built-in do while loop, but a similar behavior can be achieved using a while loop with a condition check after the code block.
Syntax:
while True:
# Code block to be executed at least once
if not condition:
break
Explanation of the Syntax:
- Python doesn’t have a built-in do while loop construct.
- However, a similar behavior can be achieved using a while loop with a conditional break statement.
- The loop is initiated with a condition that is always true (
while True:
).
- Inside the loop, the code block is executed at least once.
- After executing the code block, an
if
statement checks the condition.
- If the condition is not met (
not condition
), the loop is terminated using the break
statement.
Implementation of Do While Loop Syntax in Python:
Python3
i = 0
while i < 10 :
print (i, end = " " )
i + = 1
|
Output
0 1 2 3 4 5 6 7 8 9
Do While loop Syntax in C#:
Syntax:
do {
// Code block to be executed at least once
} while (condition);
Explanation of the Syntax:
- In C#, the do while loop operates similarly to C, C++, and Java.
- The code block enclosed within the curly braces
{}
is executed at least once.
- After executing the code block, the loop evaluates the condition specified in the parentheses
()
.
- If the condition is true, the loop continues; otherwise, it terminates.
Implementation of Do While Loop Syntax in C#:
C#
using System;
public class GFG {
static public void Main()
{
int i = 0;
do {
Console.Write(i + " " );
i++;
} while (i < 10);
}
}
|
Output
0 1 2 3 4 5 6 7 8 9
Do While loop Syntax in JavaScript:
Syntax:
do {
// Code block to be executed at least once
} while (condition);
Explanation of the Syntax:
- In JavaScript, the do while loop functions similarly to other languages.
- The code block within the curly braces
{}
is executed at least once.
- After executing the code block, the loop evaluates the condition specified in the parentheses
()
.
- If the condition is true, the loop repeats; otherwise, it exits.
Implementation of Do While Loop Syntax in JavaScript:
Javascript
let i = 0;
do {
console.log(i + " " );
i++;
} while (i < 10);
|
Output
0
1
2
3
4
5
6
7
8
9
Share your thoughts in the comments
Please Login to comment...