Difference between While Loop and Do While Loop in Programming
Last Updated :
19 Apr, 2024
While loop and Do while loop concepts are fundamental to control flow in programming, allowing developers to create loops that repeat a block of code based on certain conditions. The choice between “while” and “do while” depends on the specific requirements of the program and the desired behavior of the loop. It is important for a beginner to know the key differences between both of them.
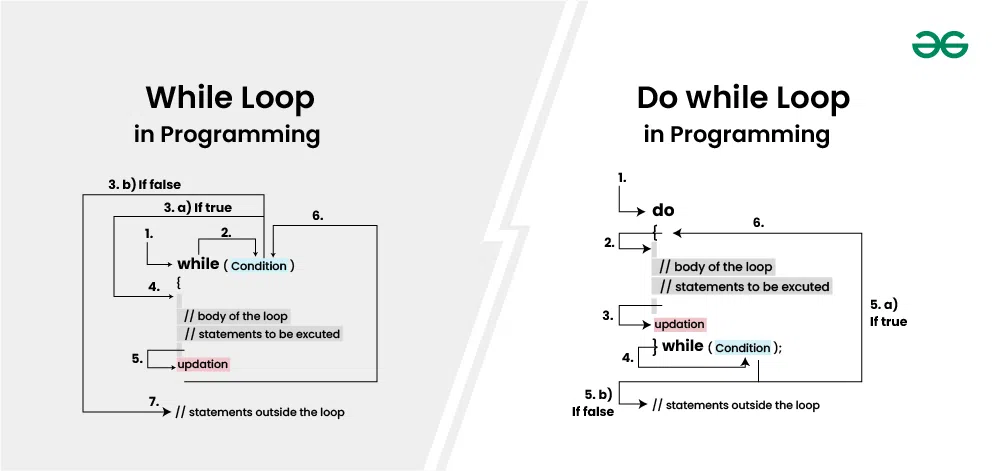
Difference between While Loop and Do While
- The condition is checked before the execution of the loop body.
- If the condition is initially false, the loop body may never be executed.
- The loop may execute zero or more times, depending on whether the condition is true or false initially.
Examples:
C++
#include <iostream>
using namespace std;
int main()
{
int count = 5;
while (count < 5) {
count += 1;
}
cout << "Final value of count = " << count << endl;
return 0;
}
C
#include <stdio.h>
int main()
{
int count = 5;
while (count < 5) {
count += 1;
}
printf("Final value of count = %d\n", count);
return 0;
}
Java
public class Main {
public static void main(String[] args) {
// Initialize count to 5
int count = 5;
// While loop: continue while count is less than 5
while (count < 5) {
count += 1; // Increment count
}
// Print the final value of count
System.out.println("Final value of count = " + count);
}
}
Python3
count = 5
while count < 5:
count += 1
print("Final value of count =", count)
C#
using System;
class Program
{
static void Main(string[] args)
{
// Initialize count to 5
int count = 5;
while (count < 5)
{
count += 1;
}
// Print the final value of count
Console.WriteLine("Final value of count = " + count);
}
}
Javascript
// Initialize count to 5
let count = 5;
// While loop: continue while count is less than 5
while (count < 5) {
count += 1; // Increment count
}
// Print the final value of count
console.log("Final value of count = " + count);
OutputFinal value of count = 5
- The loop body is executed at least once, regardless of the condition.
- After the first execution, the condition is checked.
- If the condition is true, the loop continues; otherwise, it exits.
- The loop is guaranteed to execute the code in the loop body at least once.
Examples:
C++
#include <iostream>
using namespace std;
int main()
{
int count = 5;
do {
count += 1;
} while (count < 5);
cout << "Final value of count = " << count;
return 0;
}
C
#include <stdio.h>
int main()
{
int count = 5;
do {
count += 1;
} while (count < 5);
printf("Final value of count = %d\n", count);
return 0;
}
Java
public class Main {
public static void main(String[] args) {
// Initialize count to 5
int count = 5;
// Do-while loop: increment count while it is less than 5
do {
count += 1; // Increment count
} while (count < 5);
// Print the final value of count
System.out.println("Final value of count = " + count);
}
}
Python3
count = 5
while True:
count += 1
if not count < 5:
break
print("Final value of count =", count)
C#
using System;
class Program
{
static void Main()
{
// Initialize count to 5
int count = 5;
// Do-while loop
do
{
// Increment count by 1
count += 1;
} while (count < 5); // Continue while count is less than 5
// Output final value of count
Console.WriteLine("Final value of count = " + count);
}
}
Javascript
// Initialize count to 5
let count = 5;
// Do-while loop: execute at least once and continue while count is less than 5
do {
count += 1; // Increment count
} while (count < 5);
// Print the final value of count
console.log("Final value of count = " + count);
OutputFinal value of count = 6
Difference between While Loop and Do-While Loop in Programming:
Key differences between a “while” loop and a “do-while” loop in general programming terms:
Feature | while Loop | do-while Loop |
---|
Syntax | while (condition) { } | do { } while (condition); |
---|
First Execution | Condition is checked before the loop block is executed. | Loop block is executed at least once before checking the condition. |
---|
Use Cases | Suitable when the loop block should be executed only if the condition is initially true. | Useful when the loop block must be executed at least once, regardless of the initial condition. |
---|
Initialization and Update | Initialization and update need to be handled explicitly before and inside the loop. | Initialization (if needed) is done before the loop; update (if needed) is placed inside the loop. |
---|
These differences highlight the distinct characteristics of “while” and “do-while” loops in terms of their initial conditions and the guaranteed execution of the loop body.
Share your thoughts in the comments
Please Login to comment...