Python While Else
Last Updated :
01 Feb, 2024
Python is easy to understand and a robust programming language that comes with lots of features. It offers various control flow statements that are slightly different from those in other programming languages. The “while-else” loop is one of these unique constructs. In this article, we will discuss the “while-else” loop in detail with examples.
What is Python While-Else Loop?
In Python, the while loop is used for iteration. It executes a block of code repeatedly until the condition becomes false and when we add an “else” statement just after the while loop it becomes a “While-Else Loop”. This else statement is executed only when the while loops are executed completely and the condition becomes false. If we break the while loop using the “break” statement then the else statement will not be executed.
Python While Else Syntax
while(Condition):
# Code to execute while the condition is true
else:
# Code to execute after the loop ends naturally
Example:
In this example, a ‘while’ loop iterates through numbers from 1 to 5, continuously adding each number to the ‘sum_result’. The ‘else’ block, executed when the loop condition becomes false, prints the message “Loop completed. Sum =” followed by the final sum of the numbers, which is 15.
Python3
num = 1
sum_result = 0
while num < = 5 :
sum_result + = num
num + = 1
else :
print ( "Loop completed. Sum =" , sum_result)
|
Output
Loop completed. Sum = 15
Flowchart of Python While Loop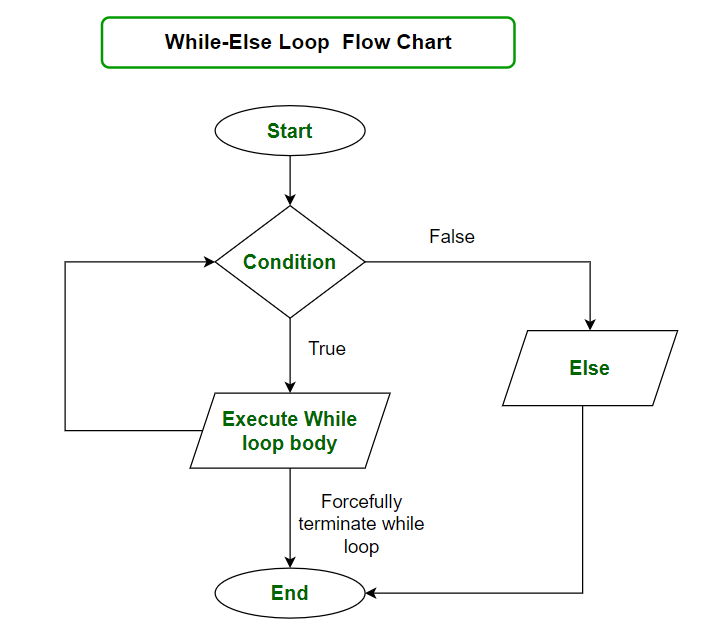
Flow Chart of While-Else Loop
Working of While-Else Loop
While Loop: This part works like any standard while loop. The code inside this block will continue to execute as long as the condition is True.
Else Clause: Once the false condition encounters in ‘while’ loop, control passes to the block of code inside the else. If the loop is terminated with break, the else block is not executed.
Infinite While-Else Loop in Python
In this example, we are understanding how we can write infinite Python While-else loops.
Python3
age = 30
while age > 19 :
print ( 'Infinite Loop' )
else :
print ( "Not a infinite loop" )
|
Control Statements in Python with Examples
Loop control statement changes the execution from their normal sequence and we can use them with Python While-else. Below are some of the ways by which we can use Python while else more effectively in Python:
Python While Else with a Break Statement
In the below code, we have created a list of numbers, after that we have written a while-else loop to search the target in the list ‘numbers’. The while loop is iterated over the list and check if the target is present in the list or not using if condition and in this example the target is not present so the while loop run completely using break statement and hence executed the else block.
Python3
numbers = [ 1 , 3 , 5 , 7 , 9 ]
target = 2
i = 0
while i < len (numbers):
if numbers[i] = = target:
print ( "Found the number!" )
break
i = i + 1
else :
print ( "Number not found." )
|
Nested While-Else loop in Python
In this example, a ‘while’ loop iterates through a list of numbers, and for each non-prime number, it finds the first composite number by checking divisibility, breaking out of the loop when found; if no composites are found, the ‘else’ block of the outer loop executes, printing a message.
Python3
nums = [ 3 , 5 , 7 , 4 , 11 , 13 ]
i = 0
while i < len (nums):
if nums[i] > 1 :
divisor = 2
while divisor < nums[i]:
if nums[i] % divisor = = 0 :
print (f "The first composite number in the list is: {nums[i]}" )
break
divisor + = 1
else :
i = i + 1
continue
break
else :
print ( "No composite numbers found in the list." )
|
Output
The first composite number in the list is: 4
Python While Else with Continue Statement
In this example, we have used continue statement in while-else loop. The below program print a table of 2 and inside the while loop we have write a condition which checks if the counter is even or not if the counter is even if statement is executed and hence “continue” is also executed and rest of the code inside the while loop is skipped. In the output, we can see that is continue statement will not affect the execution of else statement.
Python3
counter = 1
num = 2
while (counter < = 10 ):
if (counter % 2 = = 0 ):
counter + = 1
continue
print (f "{num} * {counter} = {num*counter}" )
counter + = 1
else :
print ( "Inside the else block" )
print ( "Outside the loop." )
|
Output
2 * 1 = 2
2 * 3 = 6
2 * 5 = 10
2 * 7 = 14
2 * 9 = 18
Inside the else block
Outside the loop.
Python While Else with a pass Statement
In this example, a ‘while’ loop is employed to search for the target value (5) within the first 10 integers. If the target value is found, it prints a corresponding message and breaks out of the loop; otherwise, the loop completes without finding the target using pass statement. The code outside the loop then executes, printing “Loop completed.” to indicate the end of the process.
Python3
counter = 0
target = 5
while counter < 10 :
if counter = = target:
print (f "Target value {target} found!" )
break
counter + = 1
else :
pass
print ( "Loop completed." )
|
Output
Target value 5 found!
Loop completed.
Python While Else With a User Input
In this example, we are using Python while else loop while taking the user input and demonstrating the right input.
Python3
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input ( "Enter a valid number: " )
if user_input.isdigit():
print (f "Valid input: {user_input}" )
break
else :
print ( "Invalid input. Please enter a numeric value." )
attempts + = 1
else :
print (f "Exceeded maximum attempts ({max_attempts}). Exiting program." )
|
Output:
Enter a valid number: 4
Valid input: 4
Share your thoughts in the comments
Please Login to comment...