The do-while loop is a control flow statement found in many programming languages. It is similar to the while loop, but with one key difference: the condition is evaluated after the execution of the loop’s body, ensuring that the loop’s body is executed at least once.
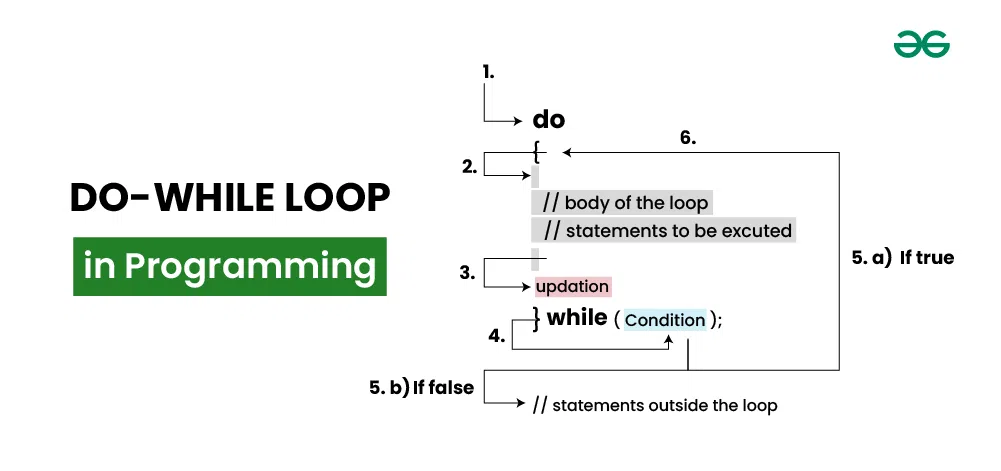
Do-While loop in Programming
What is Do-While Loop:
A do-while loop is a control flow statement (or loop statement) commonly found in many programming languages. It is similar to the while loop but with one crucial difference: the condition is evaluated after the execution of the loop’s body. This ensures that the loop’s body is executed at least once, even if the condition is initially false.
Do-While Loop Syntax:
The syntax of a do-while loop is as follows:
do {
// Statements to be executed
} while (condition);
- The block of statements within the
do
block is executed unconditionally for the first time. - After executing the block, the condition specified after the
while
keyword is evaluated. - If the condition evaluates to
true
, the loop body is executed again. If the condition evaluates to false
, the loop terminates, and program execution moves to the next statement after the loop.
Here’s a breakdown of each component of the syntax:
do
: This keyword initiates the do-while loop and precedes the block of statements to be executed.{}
: These braces enclose the block of statements to be executed within the loop.while
: This keyword specifies the condition to be evaluated after the execution of the loop body.condition
: This is the expression that determines whether the loop should continue iterating. If the condition evaluates to true
, the loop continues; if it evaluates to false
, the loop terminates.
How does Do-While Loop work?
The do-while loop is a control flow construct used in programming to execute a block of code repeatedly until a specified condition becomes false. Here’s how the do-while loop works:
- Initialization: The loop starts with the execution of the block of code inside the
do
statement. Unlike other loop types, the do-while loop guarantees that the block of code will be executed at least once, regardless of the condition. - Condition Evaluation: After executing the block of code inside the
do
statement, the condition specified in the while
statement is evaluated. This condition determines whether the loop should continue iterating or terminate. If the condition evaluates to true
, the loop continues to execute; if it evaluates to false
, the loop terminates. - Iterative Execution: If the condition evaluates to
true
, the loop body is executed again, and the process repeats. After each iteration, the condition is evaluated again to determine whether the loop should continue. - Termination: The loop terminates when the condition specified in the
while
statement evaluates to false
. Once the condition becomes false
, control exits the loop, and program execution proceeds to the next statement following the loop.
Do-While Loop in Different Programming Languages:
Do-While loops are fundamental constructs in programming and are supported by virtually all programming languages. While the syntax and specific details may vary slightly between languages, the general concept remains the same. Here’s how Do-While loops are implemented in different programming languages:
1. Do-While loop in Python:
In Python, there is no native do-while
loop construct. However, you can achieve similar functionality using a while True
loop and a conditional break
statement to exit the loop when the desired condition is met.
Python3
# Initialize a variable
count = 0
# Execute the loop body at least once
while True:
print(count) # Print the current value of count
count += 1 # Increment count by 1
if count >= 5:
break # Exit loop if count is equal to or greater than 5
Explanation: In Python, we initiate a while True
loop, ensuring that the loop body executes at least once. Inside the loop, we print the current value of count
and then increment it by 1
. The loop continues until the condition count >= 5
becomes True
, at which point we use a break
statement to exit the loop.
2. Do-While loop in JavaScript:
JavaScript’s do-while
loop behaves similarly to other languages. It executes a block of code at least once and then evaluates the loop condition. If the condition is true, the loop continues; otherwise, it terminates.
Javascript
let count = 0;
do {
console.log(count); // Print the current value of count
count++; // Increment count by 1
} while (count < 5); // Continue looping as long as count is less than 5
Explanation: In JavaScript, the do-while
loop prints the value of count
and increments it by 1
on each iteration. The loop body executes at least once, ensuring that the condition count < 5
is evaluated after the first execution. If count
is less than 5
, the loop continues executing.
3. Do-While loop in Java:
In Java, the do-while
loop is used to execute a block of code at least once before checking the loop condition. It guarantees that the loop body is executed at least once, even if the condition is initially false
.
Java
public class Main {
public static void main(String[] args) {
int count = 0;
do {
System.out.println(count); // Print the current value of count
count++; // Increment count by 1
} while (count < 5); // Continue looping as long as count is less than 5
}
}
Explanation: Here, we initialize a variable count
and use a do-while
loop to print its value and increment it by 1
on each iteration. The loop continues as long as count
is less than 5
. Even if count
is initially 5
or greater, the loop body executes at least once before the condition is checked.
4. Do-While loop in C:
In C, the do-while
loop is similar to other languages, executing a block of code at least once before evaluating the loop condition. The loop continues as long as the condition remains true.
C
#include <stdio.h>
int main() {
int count = 0;
do {
printf("%d\n", count); // Print the current value of count
count++; // Increment count by 1
} while (count < 5); // Continue looping as long as count is less than 5
return 0;
}
Explanation: In this C example, the do-while
loop prints the value of count
and increments it by 1
on each iteration. Similar to other languages, the loop body executes at least once, and the loop continues as long as the condition count < 5
remains true.
5. Do-While loop in C++:
Similar to Java, C++ also supports the do-while
loop, which guarantees that the loop body is executed at least once. The loop condition is evaluated after the first execution of the loop body.
C++
#include <iostream>
using namespace std;
int main() {
int count = 0;
do {
cout << count << endl; // Print the current value of count
count++; // Increment count by 1
} while (count < 5); // Continue looping as long as count is less than 5
return 0;
}
Explanation: Here, we initialize a variable count
and use a do-while
loop to print its value and increment it by 1
on each iteration. The loop continues as long as count
is less than 5
. Similar to Java, the loop body executes at least once before the condition is checked.
6. Do-While loop in PHP:
PHP’s do-while
loop is similar to other languages, executing a block of code at least once before evaluating the loop condition. It continues looping as long as the condition remains true.
PHP
<?php
$count = 0;
do {
echo $count . "\n"; // Print the current value of count
$count++; // Increment count by 1
} while ($count < 5); // Continue looping as long as count is less than 5
?>
Explanation: In this PHP example, the do-while
loop prints the value of count
and increments it by 1
on each iteration. The loop body executes at least once, ensuring that the condition $count < 5
is evaluated after the first execution. If $count
is less than 5
, the loop continues executing.
7. Do-While loop in C#:
In C#, the do-while
loop is similar to Java and C++. It executes a block of code at least once before evaluating the loop condition. The loop continues as long as the condition remains true
.
C#
using System;
class MainClass {
public static void Main (string[] args) {
int count = 0;
do {
Console.WriteLine(count); // Print the current value of count
count++; // Increment count by 1
} while (count < 5); // Continue looping as long as count is less than 5
}
}
Explanation: In this C# example, the do-while
loop prints the value of count
and increments it by 1
on each iteration. The loop body executes at least once, even if the condition count < 5
is initially false. After the first execution, the condition is evaluated, and if count
is less than 5
, the loop continues executing.
These examples demonstrate how do-while loops are implemented and used in various programming languages. They guarantee the execution of the loop body at least once and then continue looping based on the specified condition.
Do-While Use Cases:
The do-while
loop, which guarantees the execution of its block of code at least once, is beneficial in various scenarios. Here are some common use cases:
1. Input Validation:
- When validating user input, especially in interactive programs, it’s often necessary to prompt the user for input at least once, regardless of the initial validity of the input.
- The
do-while
loop ensures that the input validation logic is executed at least once before checking if the input meets the required criteria.
2. Menu-Driven Programs:
- In menu-driven applications where users make selections from a list of options, it’s essential to display the menu to the user at least once.
- The
do-while
loop allows you to display the menu and prompt the user for input repeatedly until they choose to exit the menu.
3. File Processing:
- When reading or processing data from files, especially where the file may be empty or contain incomplete data, the
do-while
loop ensures that the file processing logic is executed at least once. - This allows you to handle edge cases gracefully and avoid errors due to unexpected file contents.
Do-While Loop vs Other Loops:
Here’s a comparison table between the do-while loop and other common loop structures:
Aspect | Do-While Loop | While Loop | For Loop |
---|
Execution | Body executes at least once | Condition checked before execution | Initialization, condition check, iteration |
---|
Syntax | do { } while(condition); | while(condition) { } | for(initialization; condition; iteration) |
---|
Control Flow | Always executes at least once | May not execute if condition is false | May not execute if condition is false |
---|
Condition Evaluation | After the loop body | Before entering the loop body | Before entering the loop body |
---|
Loop Control Variables | May need to declare outside the loop | Typically declared outside the loop | Declared within the loop |
---|
Initialization | Not explicitly initialized in the loop | Not explicitly initialized in the loop | Initialized in the loop declaration |
---|
Use Cases | Input validation, game loops, error handling | Condition-based looping | Counter-based looping |
---|
Flexibility | Provides flexibility for executing code | Flexibility in defining condition | Flexibility in controlling loop parameters |
---|
In conclusion, the while loop emerges as a potent tool in the arsenal of any programmer, providing unmatched versatility and precision in controlling iteration. Mastering the intricacies of the while loop empowers developers to craft elegant, efficient, and robust solutions to a myriad of programming challenges.
Share your thoughts in the comments
Please Login to comment...