Loops in Programming
Last Updated :
06 Apr, 2024
In the field of programming, Iteration Statements are helpful when we need a specific task in repetition. They’re essential as they reduce hours of work to seconds. In this article, we will explore the basics of loops, with the different types and best practices.
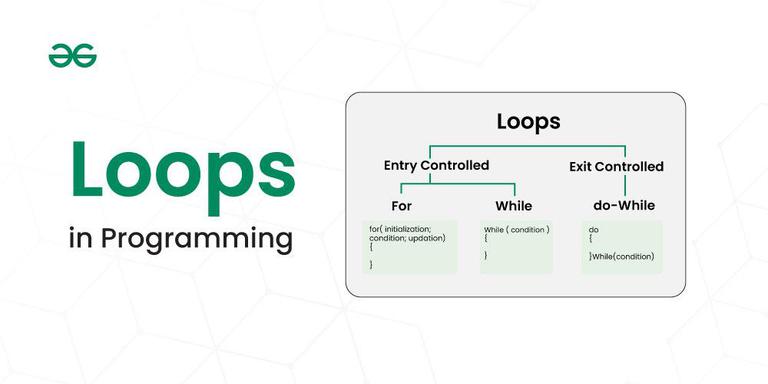
Loops in Programming
What are Loops in Programming?
Loops, also known as iterative statements, are used when we need to execute a block of code repetitively. Loops in programming are control flow structures that enable the repeated execution of a set of instructions or code block as long as a specified condition is met. Loops are fundamental to the concept of iteration in programming, enhancing code efficiency, readability and promoting the reuse of code logic.
Types of Loops in Programming:
In programming, loops are categorized into two main types based on the control mechanism: entry-controlled loops and exit-controlled loops.
1. Entry-Controlled loops:
In Entry controlled loops the test condition is checked before entering the main body of the loop. For Loop and While Loop is Entry-controlled loops.
Below are the examples of Entry-controlled loops:
C++
#include <iostream>
using namespace std;
int main()
{
// Entry-controlled for loop
int i;
for (i = 0; i < 5; i++) {
cout << i << " ";
}
cout << endl;
// Entry-controlled while loop
i = 0;
while (i < 5) {
cout << i << " ";
i++;
}
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Entry-controlled for loop
int i;
for (i = 0; i < 5; i++) {
System.out.print(i + " ");
}
System.out.println();
// Entry-controlled while loop
i = 0;
while (i < 5) {
System.out.print(i + " ");
i++;
}
}
}
Python3
# Entry-controlled for loop
for i in range(5):
print(i, end=" ")
print()
# Entry-controlled while loop
i = 0
while(i < 5):
print(i, end=" ")
i += 1
C#
// c# code for the above approach
using System;
class Program {
static void Main()
{
// Entry-controlled for loop
for (int i = 0; i < 5; i++) {
Console.Write(i + " ");
}
Console.WriteLine();
// Entry-controlled while loop
int j = 0;
while (j < 5) {
Console.Write(j + " ");
j++;
}
}
}
Javascript
let outputFor = '';
let outputWhile = '';
// Entry-controlled for loop
for (let i = 0; i < 5; i++) {
outputFor += i + ' ';
}
// Entry-controlled while loop
let j = 0;
while (j < 5) {
outputWhile += j + ' ';
j++;
}
console.log(outputFor);
console.log(outputWhile);
Output0 1 2 3 4
0 1 2 3 4
2. Exit-Controlled loops:
In Exit controlled loops the test condition is evaluated at the end of the loop body. The loop body will execute at least once, irrespective of whether the condition is true or false. Do-while Loop is an example of Exit Controlled loop.
Below are the examples of Exit-controlled loops:
C++
#include <iostream>
using namespace std;
int main()
{
// Exit controlled do-while loop
int i = 0;
do {
cout << i << " ";
i++;
} while (i < 5);
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Exit controlled do-while loop
int i = 0;
do {
System.out.print(i + " ");
i++;
} while (i < 5);
}
}
Python3
# Exit controlled do-while loop
i = 0
while True: # In Python, there's no direct equivalent of a do-while loop, so we use a while loop with a conditional break
print(i, end=" ") # Print the current value of i followed by a space
i += 1 # Increment i
if i >= 5: # Check if i is greater than or equal to 5
break # If so, exit the loop
C#
using System;
class Program
{
static void Main()
{
// Initialize the counter variable
int i = 0;
// Exit-controlled do-while loop
do
{
// Print the current value of i
Console.Write($"{i} ");
// Increment the counter
i++;
} while (i < 5);
// Return 0 to indicate successful completion
Environment.Exit(0);
}
}
Javascript
let i = 0;
let output = '';
do {
output += i + ' ';
i++;
} while (i < 5);
console.log(output);
For loop:
A for loop in programming is a control flow structure that iterates over a sequence of elements, such as a range of numbers, items in a list, or characters in a string. The loop is entry-controlled because it determines the number of iterations before entering the loop.
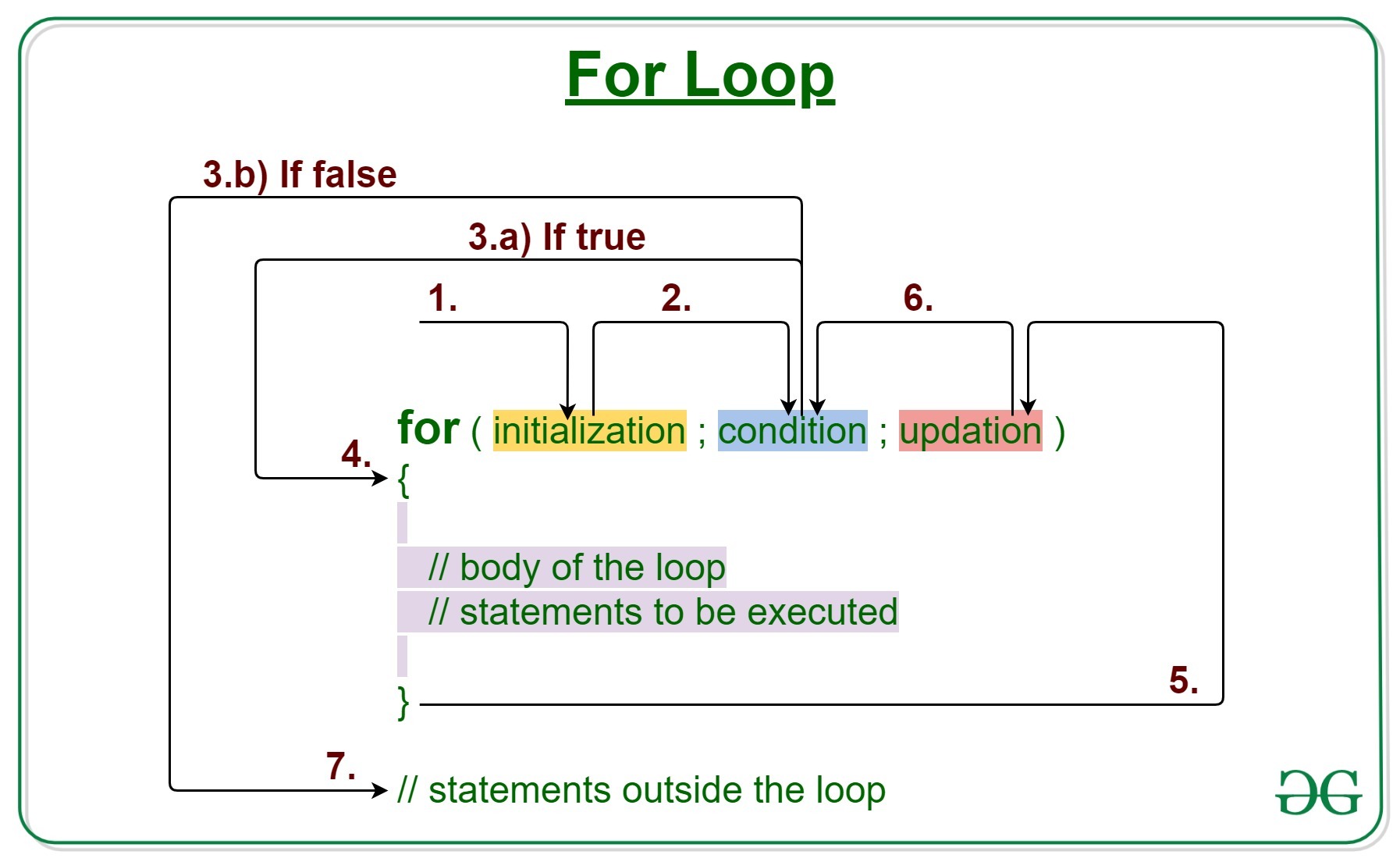
for-loop
The basic syntax for a for loop often includes a variable that takes on each value in the sequence, and the loop body contains the code to be executed during each iteration.
C++
#include <iostream>
using namespace std;
int main()
{
for (int i = 0; i < 5; i++) {
cout << i << " ";
}
cout << endl;
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Entry-controlled for loop
for (int i = 0; i < 5; i++) {
System.out.print(i + " ");
}
System.out.println();
}
}
Python3
# Entry-controlled for loop
for i in range(5):
print(i, end=" ")
print()
C#
using System;
class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 5; i++)
{
Console.Write(i + " ");
}
Console.WriteLine();
}
}
Javascript
let output = '';
for (let i = 0; i < 5; i++) {
output += i + ' ';
}
console.log(output);
While Loop:
A while loop in programming is an entry-controlled control flow structure that repeatedly executes a block of code as long as a specified condition is true. The loop continues to iterate while the condition remains true, and it terminates once the condition evaluates to false.
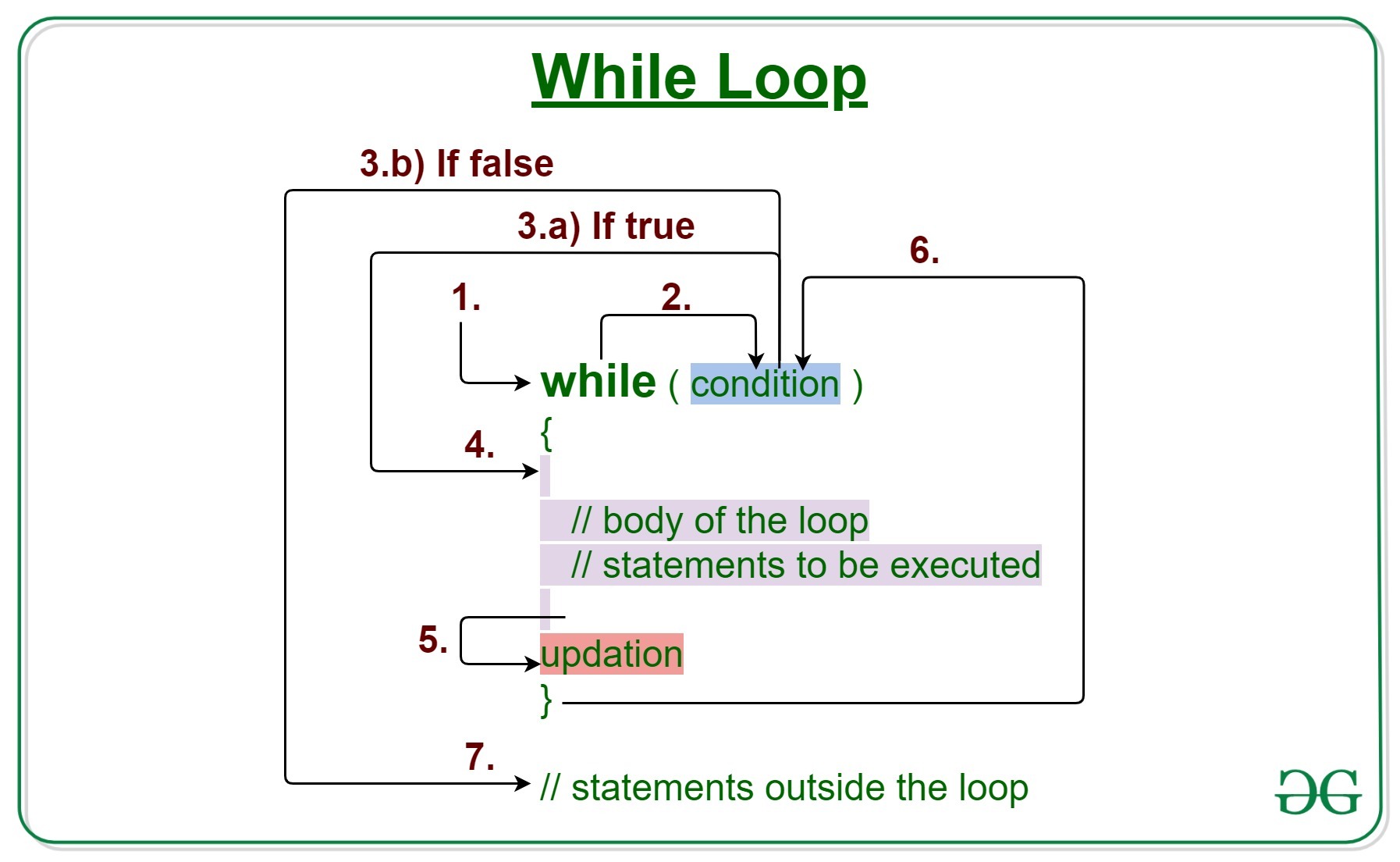
while loop
The basic syntax for a for loop often includes a condition which is checked before each iteration and if the condition evaluates to true, the loop body containing the code gets executed otherwise the loop gets terminated.
C++
#include <iostream>
using namespace std;
int main()
{
int i = 0;
while (i < 5) {
cout << i << " ";
i++;
}
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
int i = 0;
while (i < 5) {
System.out.print(i + " ");
i++;
}
}
}
Python3
# code
i = 0
while i < 5:
print(i, end=" ")
i += 1
C#
using System;
class Program
{
static void Main()
{
// Initialize the counter variable
int i = 0;
// Execute the loop while the condition is true
while (i < 5)
{
// Print the current value of i
Console.Write($"{i} ");
// Increment the counter
i++;
}
// Return 0 to indicate successful completion
Environment.Exit(0);
}
}
JavaScript
function main() {
let i = 0;
while (i < 5) {
process.stdout.write(i + " ");
i++;
}
}
// Call the main function
main();
Do-While Loop:
A do-while loop in programming is an exit-controlled control flow structure that executes a block of code at least once and then repeatedly executes the block as long as a specified condition remains true. The distinctive feature of a do-while loop is that the condition is evaluated after the code block, ensuring that the block is executed at least once, even if the condition is initially false.
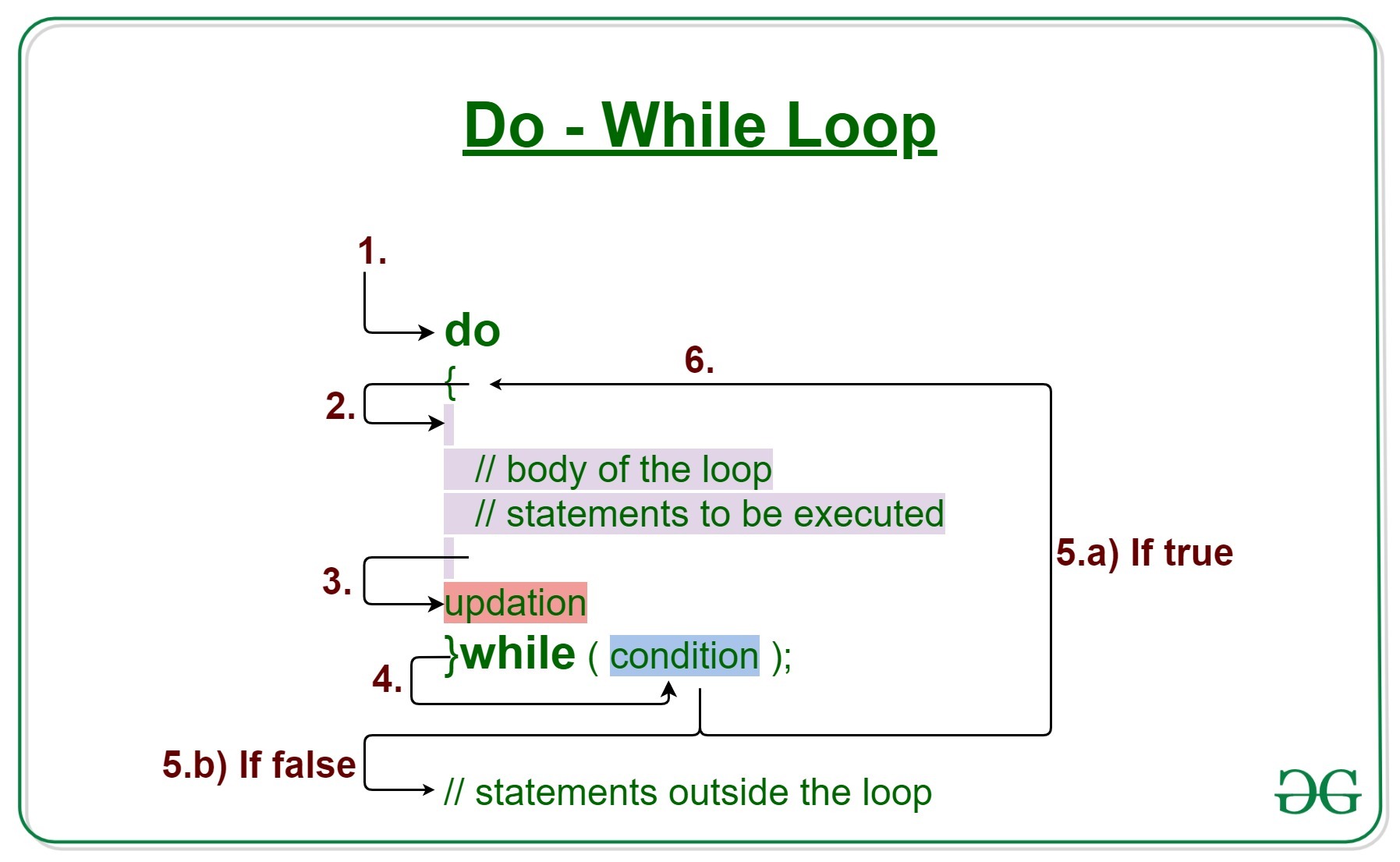
Do-while loop
The basic syntax for a for loop includes a block of code followed by a condition which is checked after each iteration and if the condition evaluates to true, the next iteration takes place otherwise the loop gets terminated.
C++
#include <iostream>
using namespace std;
int main()
{
int i = 0;
do {
cout << i << " ";
i++;
} while (i < 5);
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
int i = 0;
do {
System.out.print(i + " ");
i++;
} while (i < 5);
}
}
Python3
# Python program to demonstrate a simple do-while loop
def main():
# Initialize the counter variable
i = 0
# Execute the loop at least once, and continue while the condition is true
while True:
# Print the current value of i
print(i, end=" ")
# Increment the counter
i += 1
# Check if the loop condition is no longer true
if i >= 5:
break
# Call the main function
main()
C#
using System;
class Program
{
static void Main()
{
// Initialize the counter variable
int i = 0;
// Execute the loop at least once
do
{
// Print the current value of i
Console.Write($"{i} ");
// Increment the counter
i++;
} while (i < 5);
// Return 0 to indicate successful completion
Environment.Exit(0);
}
}
JavaScript
// Define the main function
function main() {
// Initialize the variable i
let i = 0;
// Start a do-while loop
do {
// Print the current value of i
process.stdout.write(i + " ");
// Increment i
i++;
} while (i < 5); // Continue looping while i is less than 5
}
// Call the main function
main();
Nested Loops:
Nested loops in programming are when one loop is placed inside another. This allows for the iteration of one or more loops within the body of another loop. Each time the outer loop executes, the inner loop completes its full iteration. Nested loops are commonly employed for tasks involving multidimensional data processing, pattern printing, or handling complex data structures. Efficient use of nested loops is essential, considering potential impacts on code readability and execution time for larger datasets.
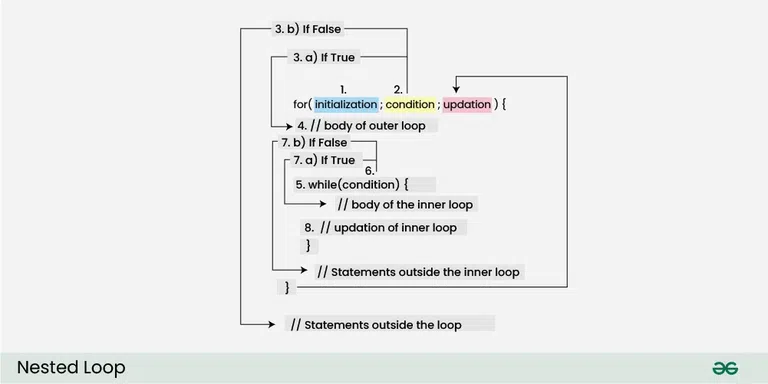
Nested Loop
C++
#include <iostream>
using namespace std;
int main()
{
for (int i = 0; i < 3; i++) {
int j = 0;
while (j < 5) {
cout << "i = " << i << " j = " << j << " ";
j++;
}
cout << endl;
}
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
for (int i = 0; i < 3; i++) {
int j = 0;
while (j < 5) {
System.out.print("i = " + i + " j = " + j
+ " ");
j++;
}
System.out.println();
}
}
}
Python3
for i in range(3):
j = 0
while j < 5:
print(f"i = {i} j = {j} ", end="")
j += 1
print()
C#
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 3; i++)
{
int j = 0;
while (j < 5)
{
Console.Write($"i = {i} j = {j} ");
j++;
}
Console.WriteLine();
}
}
}
JavaScript
// Define the main function
function main() {
// Start a for loop to iterate from 0 to 2
for (let i = 0; i < 3; i++) {
// Initialize j
let j = 0;
// Start a while loop to iterate while j is less than 5
while (j < 5) {
// Print the values of i and j
console.log("i = " + i + " j = " + j + " ");
// Increment j
j++;
}
// Print a newline after each inner loop iteration
console.log();
}
}
// Call the main function
main();
Outputi = 0 j = 0 i = 0 j = 1 i = 0 j = 2 i = 0 j = 3 i = 0 j = 4
i = 1 j = 0 i = 1 j = 1 i = 1 j = 2 i = 1 j = 3 i = 1 j = 4
i = 2 j = 0 i = 2 j = 1 i = 2 j = 2 i = 2 j = 3 i = 2 j = 4
For Loop vs While Loop vs Do-While Loop in Programming:
Characteristic | for Loop | while Loop | do-while Loop |
---|
Syntax | for (initialization; condition; update) | while (condition) | do { } while (condition); |
---|
Condition Check | Checked before each iteration. | Checked before entering the loop. | Checked after executing the loop body. |
---|
Update/Increment | Executed after each iteration. | Should be included within the loop body. | Executed after each iteration. |
---|
Use Cases | Suitable when the number of iterations is known. | Useful when the loop condition is based on a state that can change anywhere in the loop body. | Ensures the loop body is executed at least once before checking the condition. |
---|
Common mistakes to avoid in Programming:
- Infinite Loops: Not ensuring that the loop condition will eventually become false can lead to infinite loops, causing the program to hang.
- Off-by-One Errors: Mismanaging loop counters or conditions can lead to off-by-one errors, either skipping the first iteration or causing an extra, unintended iteration.
- Uninitialized Variables: Forgetting to initialize loop control variables properly can result in unpredictable behavior.
- Inefficient Nesting: Excessive or inefficient use of nested loops can lead to performance issues, especially with large datasets.
- Modifying Loop Variable Inside the Loop: Changing the loop variable inside the loop can lead to unexpected behavior. For example, modifying the iterator variable in a for loop.
In conclusion, loops in Programming are very useful in programming as they help to repeat and automate tasks. Whether through entry-controlled loops like for and while, or exit-controlled loops like do-while, loops form the backbone of algorithmic logic, enabling the creation of robust software that can handle repetitive operations, iterate through data structures, and execute complex tasks. Mastering loop structures is fundamental for any programmer seeking to optimize code, enhance readability, and master algorithm design.
Share your thoughts in the comments
Please Login to comment...