What is Angular Router?
Last Updated :
19 Apr, 2024
Angular Router is an important feature of the Angular framework that helps you to build rich and interactive single-page applications (SPAs) with multiple views. In this article, we’ll learn in detail about Angular Router.
Prerequisites
What is Angular Router?
Angular Router is a powerful navigation library that allows you to manage navigation and state within Angular applications. It provides a way to map URL paths to specific components, allowing users to navigate between different views seamlessly.
Syntax:
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'products/:id', component: ProductDetailComponent },
{ path: 'admin', loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule) },
{ path: '**', redirectTo: '/' } // Redirects unmatched paths to the home route
];
Features of Angular Router
- Component-Based Routing: Angular Router enables to define routes for different components. Each route maps a URL path to a specific component, enabling navigation to different views within the application.
- Nested Routing: Angular Router supports nested routing, allowing you to define child routes within parent routes. This enables the creation of complex navigational hierarchies and nested views within Angular applications.
- Route Parameters: Angular Router allows you to define route parameters in the URL path. Route parameters are placeholders in the URL that can be used to pass dynamic data to components, enabling dynamic routing and content generation.
- Router Guards: Angular Router provides router guards that protect routes and control navigation based on certain conditions. Router guards include canActivate, canActivateChild, canDeactivate, and resolve guards, enabling authentication, authorization, and data preloading.
- Lazy Loading: Angular Router supports lazy loading, that helps to load modules and components asynchronously when navigating to a particular route.
Steps to create an Angular app
Step 1: Set Up Angular CLI
npm install -g @angular/cli
Step 2: Create a New Angular Project
Once Angular CLI is installed, you can create a new Angular project using the ng new command:
ng new <-foldername->
Navigate into the newly created project directory:
cd <-folder-name->
Project Structure:
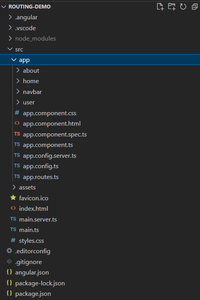
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Step 3: Generate Components for Routing
Generate components for demonstration purposes. For example, let’s create four components named home and about:
ng generate component navbar
ng generate component home
ng generate component about
ng generate component user
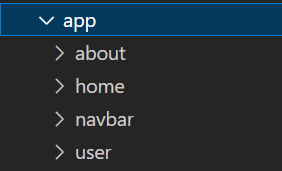
Step 4: Add there following codes in the required files.
HTML
<!-- // app.component.html -->
<app-navbar></app-navbar>
<router-outlet></router-outlet>
HTML
<!-- Navbar.component.html -->
<nav>
<ul>
<li><a routerLink="/">Home</a></li>
<li><a routerLink="/about">About</a></li>
<li><a routerLink="/user">User</a></li>
</ul>
</nav>
<style>
nav {
background-color: rgb(129, 236, 125);
padding: 6px;
}
li {
display: inline;
align-items: center;
margin: 12px;
}
</style>
HTML
<!-- home.component.html -->
<p>home works!</p>
<p>Angular router project overview</p>
<style>
p {
background-color: white;
border: 1px solid #777;
padding: 5px;
}
</style>
HTML
<!-- about.component.html -->
<p>about works! the Project all about routing in Angular</p>
<style>
p {
background-color: white;
border: 1px solid #777;
padding: 5px;
}
</style>
HTML
<!-- user.component.html -->
<p>user works!</p>
<p>Angular router project overview</p>
<style>
p {
background-color: white;
border: 1px solid #777;
padding: 5px;
}
</style>
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
import { RouterOutlet } from '@angular/router';
import { NavbarComponent } from './navbar/navbar.component';
@Component({
selector: 'app-root',
standalone: true,
templateUrl: './app.component.html',
styleUrl: './app.component.css',
imports: [CommonModule, RouterOutlet, NavbarComponent]
})
export class AppComponent {
title = 'routing-demo';
}
JavaScript
// navbar.component.ts
import { Component } from '@angular/core';
import { RouterLink } from '@angular/router';
@Component({
selector: 'app-navbar',
standalone: true,
imports: [RouterLink],
templateUrl: './navbar.component.html',
styleUrl: './navbar.component.css'
})
export class NavbarComponent {
}
JavaScript
// app.routes.ts
import { Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
import { UserComponent } from './user/user.component';
export const routes: Routes = [
{ 'path': '', component: HomeComponent },
{ 'path': 'about', component: AboutComponent },
{ 'path': 'user', component: UserComponent },
];
Step 5: Serve the Application
Navigate back to the root of your project directory and serve the application using Angular CLI:
ng serve --open
Output:
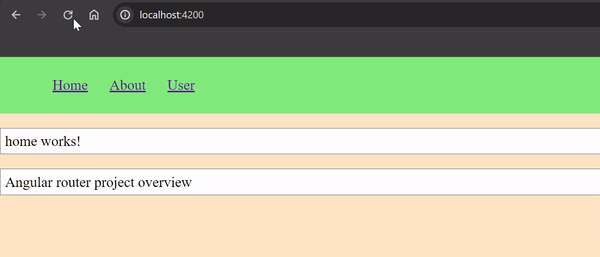
This setup provides a basic demonstration of routing in Angular. You can expand upon it by adding more components and routes as needed for your application.
Angular Router – FAQs
How do I define routes in Angular Router?
Routes in Angular Router are defined using a configuration array where each route is an object with properties like path (URL path) and component (corresponding component to render). These routes are typically defined in the app-routing.module.ts file.
How do I navigate between routes programmatically?
You can navigate between routes programmatically using the Router service provided by Angular. Import the Router service in your component or service, and then use methods like navigateByUrl or navigate to navigate to a specific route.
How do I handle 404 page not found errors in Angular Router?
Angular Router provides a wildcard route ({ path: ‘**’, redirectTo: ” }) that matches any URL path not defined by other routes. You can use this wildcard route to redirect to a custom 404 page or display a default error message.
Can I use Angular Router with lazy loading?
Yes, Angular Router supports lazy loading, allowing you to load modules asynchronously when navigating to a route. This can improve initial loading times and application performance by only loading the necessary modules when needed.
Share your thoughts in the comments
Please Login to comment...