What is a custom directive in Angular?
Last Updated :
22 Mar, 2024
Angular, a popular framework for building dynamic web applications, offers a powerful feature known as custom directives. These directives extend the functionality of HTML elements, enabling to create reusable components and add behavior to their applications. In this article, we’ll learn about the concept of custom directives in Angular.
What is Custom Directive?
Custom directives in Angular provide a mechanism to create reusable components and add behavior to HTML elements. They help to encapsulate complex functionality, apply dynamic behavior, and enhance the structure of the DOM. Custom directives are defined as TypeScript classes decorated with Angular’s @Directive()
decorator.
Features of Custom Directives:
- Encapsulation of Behavior: Custom directives encapsulate specific functionality, provides code reusability and maintainability.
- Dynamic Behavior: They enable the application of dynamic behavior to HTML elements based on user interactions, data changes, or application logic.
- Access to DOM: Directives can access and manipulate the DOM using Angular’s
ElementRef
service, providing fine-grained control over the presentation layer. - Reusable Components: Custom directives can be reused across different parts of the application, provides modular development and reducing code duplication.
Uses of Custom Directives:
- Adding Styling: Directives can be used to apply dynamic styles to HTML elements based on application state or user interactions.
- Handling User Input: They enable the creation of custom input controls and validation logic for forms.
- Structural Manipulation: Directives can manipulate the structure of the DOM, conditionally rendering elements or repeating content based on data.
- Integrating Third-party Libraries: Custom directives can integrate with third-party libraries or frameworks, encapsulating their functionality within Angular components.
Creating Custom Attribute
Step 1: Setting Up Angular Project
Install Angular CLI globally (if not already installed)
npm install -g @angular/cli
Create a new Angular project:
ng new custom-attribute-demo
Navigate into the project directory:
cd custom-attribute-demo
Step 2: Create a Custom Attribute Directive
Create a new custom attribute directive:
ng generate directive highlight
Folder Structure:
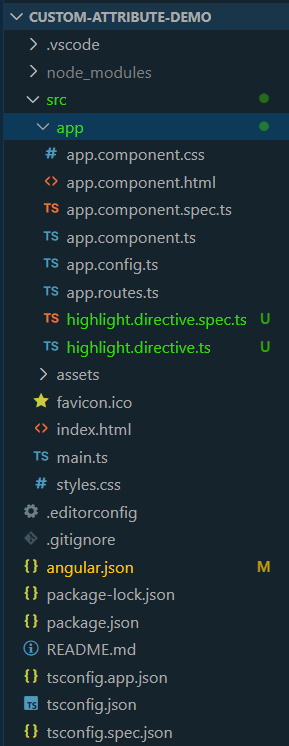
Folder Structure.
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/jasmine": "~5.1.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Example: Add the following codes in the respective files.
HTML
<!-- app.component.html -->
<p appHighlight="cyan">
AngularJS was developed in 2008-2009 by Misko Hevery and Adam Abrons and is
now maintained by Google. AngularJS is a Javascript open-source front-end
framework. It is used to develop single-page web applications (SPAs).<br/> It is a
continuously growing and expanding framework which provides better ways for
developing web applications.<br/> In this AngularJS tutorial, we will explore the
key concepts, features, and steps to kickstart your journey in creating robust
and interactive web applications.
<br />
This AngularJS tutorial is designed for beginners as well as professionals,
which covers a wide range of important topics, including AngularJS
Expressions,<br/> AngularJS directives, AngularJS Data Binding, AngularJS
controllers, AngularJS modules, AngularJS scopes, filters, and more. <br />
Vehicula ipsum a arcu cursus vitae. Lectus nulla at volutpat diam ut venenatis
tellus. Malesuada nunc vel risus commodo. Rutrum quisque non tellus orci ac.
<br />
AngularJS is an open-source, client-side framework that allows developers to
build dynamic and responsive web applications. It extends HTML with new
attributes and binds data to HTML with expressions. <br/>The framework is designed
to make both the development and testing of such applications more accessible
by providing a well-defined structure and a set of tools.
<br />
Two-Way Data Binding: One of the standout features of AngularJS is its two-way
data binding. This means that changes in the user interface automatically
update the underlying data model and vice versa. <br/>This simplifies the
synchronization of data between the view and the model, reducing the need for
manual manipulation.
</p>
JavaScript
//highlight.directive.ts
import { Directive, ElementRef, Input, OnInit } from '@angular/core';
@Directive({
standalone: true,
selector: '[appHighlight]'
})
export class HighlightDirective implements OnInit {
@Input() appHighlight: string = "";
constructor(private el: ElementRef) { }
ngOnInit() {
this.el.nativeElement.style.backgroundColor = this.appHighlight || 'yellow';
}
}
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { HighlightDirective } from './highlight.directive'
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, HighlightDirective],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
title = 'custom-attribute-demo';
}
To start the application run the following command.
ng serve
Output:
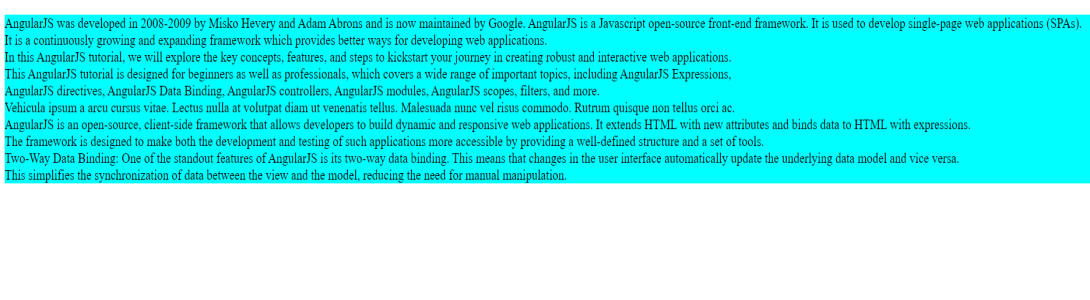
What is a custom directive in Angular
Share your thoughts in the comments
Please Login to comment...